Xradar Basics¶
Overview¶
- Xradar general overview
- Radar data IO
- Radar data georeferencing
- Data visualization
Prerequisites¶
Concepts | Importance | Notes |
---|---|---|
Intro to Xarray | Necessary | Basic features |
Radar Cookbook | Necessary | Radar basics |
Matplotlib | Necessary | Plotting basic features |
- Time to learn: 30 minutes
Imports¶
import xradar as xd
import pyart
import wradlib as wrl
import fsspec
import numpy as np
from xarray.backends.api import open_datatree
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
## You are using the Python ARM Radar Toolkit (Py-ART), an open source
## library for working with weather radar data. Py-ART is partly
## supported by the U.S. Department of Energy as part of the Atmospheric
## Radiation Measurement (ARM) Climate Research Facility, an Office of
## Science user facility.
##
## If you use this software to prepare a publication, please cite:
##
## JJ Helmus and SM Collis, JORS 2016, doi: 10.5334/jors.119
Xradar¶
Xradar
is a Python
package developed to streamline the reading and writing of radar data across various formats, with exports aligned to standards like ODIM_H5 and CfRadial. Born from the Open Radar Science Community’s collaboration at ERAD2022, Xradar
uses an xarray-based
data model, compatible with the upcoming CfRadial2.1/FM301 standard. This ensures seamless integration with other xarray-based software and existing open-source radar processing tools.
Xradar data model¶
Xradar leverages the upcoming FM301 standard, a subset of CfRadial2.0, using Xarray to efficiently manage radar data.
DataTree Structure (CfRadial2.1/FM301 standard)¶
Xradar employs xarray.DataTree
objects to organize radar sweeps within a single structure, where each sweep is an xarray.Dataset
containing relevant metadata and variables.
Xradar importers¶
Xradar supports several importers to handle different radar data formats. These importers typically include:
ODIM_H5: For reading radar data in the ODIM_H5 format, which is widely used in Europe and supported by the EUMETNET OPERA program.
CfRadial: For importing data in the CfRadial format, which is commonly used in the atmospheric sciences community.
SIGMET/IRIS: For ingesting radar data from SIGMET/IRIS formats, which are used by various weather radar systems globally.
GAMIC: For reading data from GAMIC radars, which use their proprietary format.
For more importers check here
Let’s find some Italian radar data located at the Erad 2024 Bucket
# Set the URL and path for the cloud
URL = 'https://js2.jetstream-cloud.org:8001/'
path = f'pythia/radar/erad2024'
fs = fsspec.filesystem("s3", anon=True, client_kwargs=dict(endpoint_url=URL))
fs.glob(f"{path}/*")
['pythia/radar/erad2024/.DS_Store',
'pythia/radar/erad2024/20240522_MeteoSwiss_ARPA_Lombardia',
'pythia/radar/erad2024/baltrad',
'pythia/radar/erad2024/dda_data',
'pythia/radar/erad2024/gpm_api',
'pythia/radar/erad2024/lrose',
'pythia/radar/erad2024/pyrad',
'pythia/radar/erad2024/wradlib',
'pythia/radar/erad2024/zarr_radar']
files = fs.glob("pythia/radar/erad2024/20240522_MeteoSwiss_ARPA_Lombardia/Data/Cband/*.nc")
files[:3]
['pythia/radar/erad2024/20240522_MeteoSwiss_ARPA_Lombardia/Data/Cband/MonteLema_202405221300.nc',
'pythia/radar/erad2024/20240522_MeteoSwiss_ARPA_Lombardia/Data/Cband/MonteLema_202405221305.nc',
'pythia/radar/erad2024/20240522_MeteoSwiss_ARPA_Lombardia/Data/Cband/MonteLema_202405221310.nc']
radar_files = [f"s3://{i}" for i in files]
radar_files[:3]
['s3://pythia/radar/erad2024/20240522_MeteoSwiss_ARPA_Lombardia/Data/Cband/MonteLema_202405221300.nc',
's3://pythia/radar/erad2024/20240522_MeteoSwiss_ARPA_Lombardia/Data/Cband/MonteLema_202405221305.nc',
's3://pythia/radar/erad2024/20240522_MeteoSwiss_ARPA_Lombardia/Data/Cband/MonteLema_202405221310.nc']
local_files = [
fsspec.open_local(
f"simplecache::{URL}{i}", s3={"anon": True}, filecache={"cache_storage": "."}
)
for i in files[:5]
]
We can open one of this nc
files using xradar.io.open_cfradial1_datree
method
dt = xd.io.open_cfradial1_datatree(local_files[0])
display(dt)
In this Xarray.Datatree
object, the first two nodes contains radar metadata and the others contains Xarray.Datasets
for each sweeps.
for sweep in dt.children:
try:
print(f"{sweep} - elevation {np.round(dt[sweep]['sweep_fixed_angle'].values[...], 1)}")
except KeyError:
print (sweep)
radar_parameters
georeferencing_correction
sweep_0 - elevation -0.20000000298023224
sweep_1 - elevation 0.4000000059604645
sweep_2 - elevation 1.0
sweep_3 - elevation 1.600000023841858
sweep_4 - elevation 2.5
sweep_5 - elevation 3.5
sweep_6 - elevation 4.5
sweep_7 - elevation 5.5
sweep_8 - elevation 6.5
sweep_9 - elevation 7.5
sweep_10 - elevation 8.5
sweep_11 - elevation 9.5
sweep_12 - elevation 11.0
sweep_13 - elevation 13.0
sweep_14 - elevation 16.0
sweep_15 - elevation 20.0
sweep_16 - elevation 25.0
sweep_17 - elevation 30.0
sweep_18 - elevation 35.0
sweep_19 - elevation 40.0
Let’s explore the 1.0
degrees elevation (‘sweep_2’)
ds_sw2 = dt["sweep_2"].ds
display(ds_sw2)
Xradar visualization¶
We can make a plot using xarray.plot
functionality.
ds_sw2.reflectivity.plot(
cmap="ChaseSpectral",
vmax=60,
vmin=-10,
)
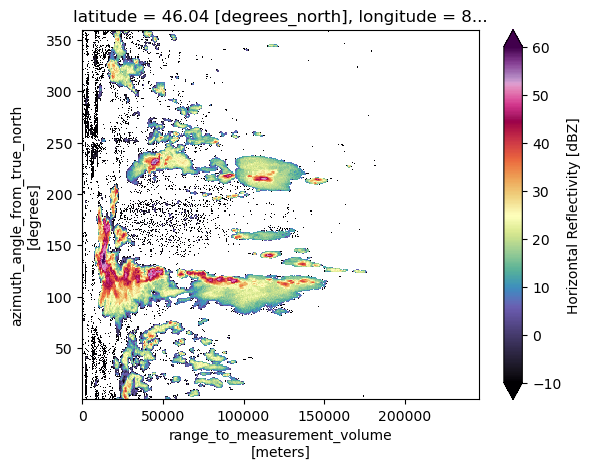
The radar data in the Xarray.Dataset
object includes range and azimuth as coordinates. To create a radial plot, apply the xradar.georeference
method. This method will generate x, y, and z coordinates for the plot.
ds_sw2 = ds_sw2.xradar.georeference()
display(ds_sw2)
We can now create a radial plot passing x
and y
coordinates
ds_sw2.reflectivity.plot(
x="x",
y= "y",
cmap="ChaseSpectral",
vmax=60,
vmin=-10,
)
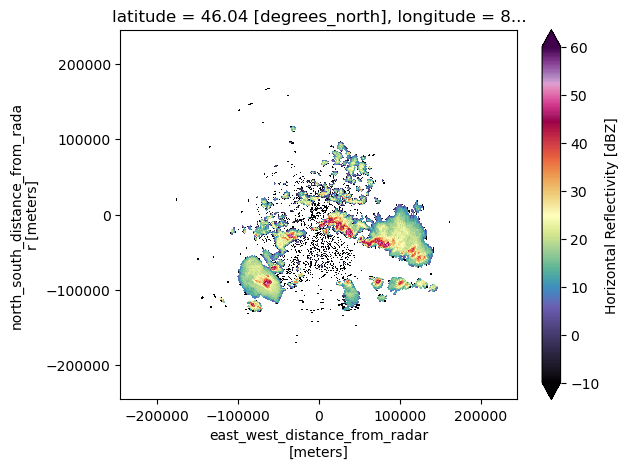
Data slicing¶
We can use the power of Xarray
to acces data within the first 50 kilometers by slicing along coordinates.
ds_sw2.sel(range=slice(0, 5e4)).reflectivity.plot(
x="x",
y= "y",
cmap="ChaseSpectral",
vmax=60,
vmin=-10,
)
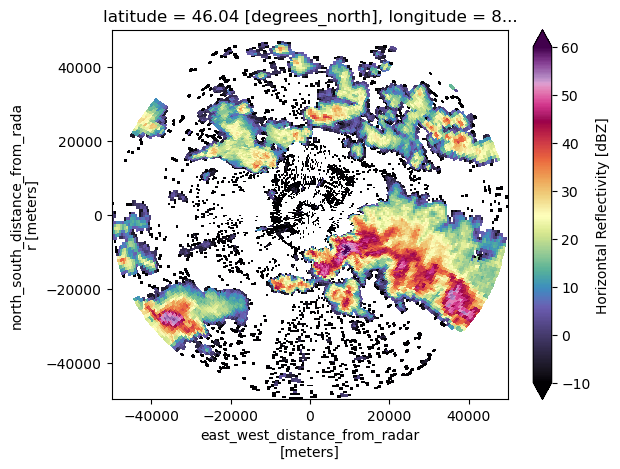
Let’s suposse we want to subset between 90 and 180 degrees angle in azumith
ds_sw2.sel(azimuth=slice(90, 180), range=slice(0, 5e4)).reflectivity.plot(
x="x",
y= "y",
cmap="ChaseSpectral",
vmax=60,
vmin=-10,
)
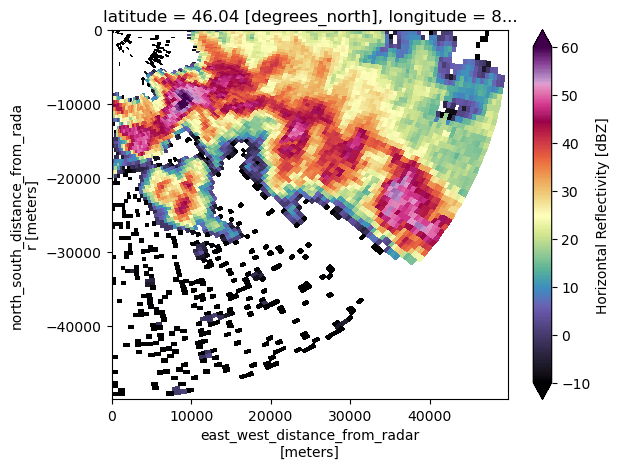
Perhaps, we just what to see radar reflectivity along the 100 degrees angle in azimuth
ds_sw2.sel(azimuth=100, method="nearest").reflectivity.plot()
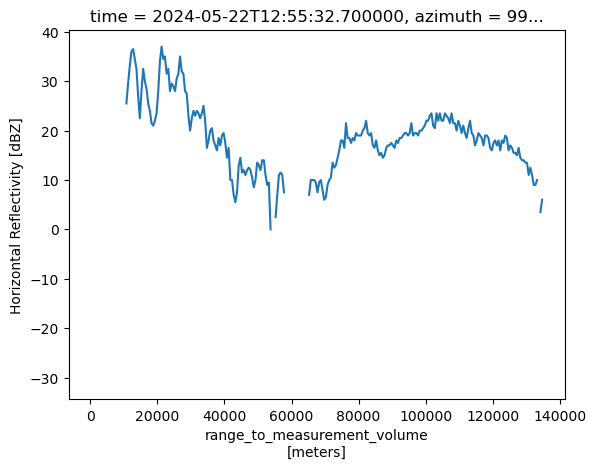
Xradar integration¶
Py-Art¶
Xradar
datatree objects can be ported to Py-ART
radar objects using the pyart.xradar.Xradar method.
radar = pyart.xradar.Xradar(dt)
fig = plt.figure(figsize=[10, 8])
display = pyart.graph.RadarMapDisplay(radar)
display.plot_ppi_map('reflectivity', sweep=0)
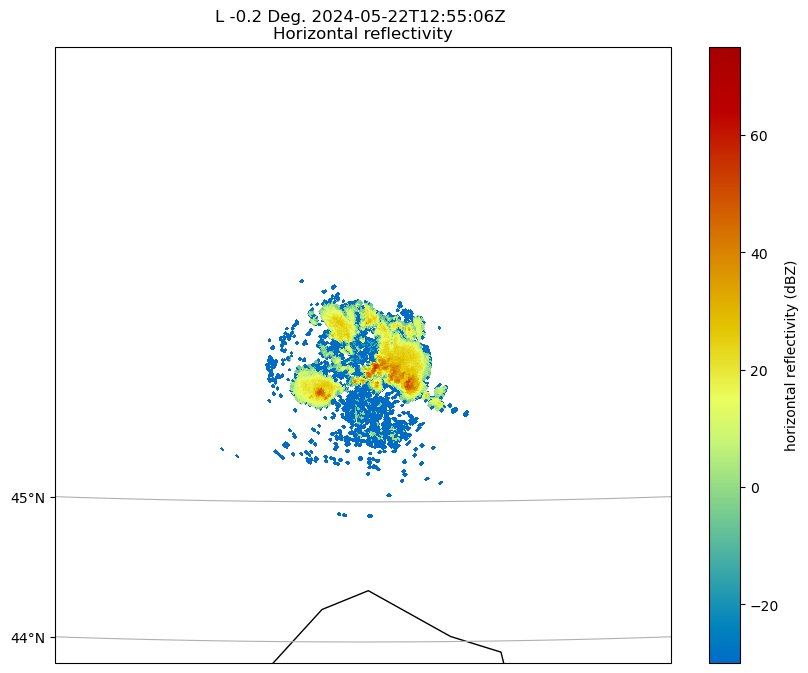
del display
Wradlib¶
Wradlib
functionality can also be applied to Xarray.Datatree
objects. For example, the wradlib.georef
module can be used to enrich data by adding geographical coordinates.
for key in list(dt.children):
if "sweep" in key:
dt[key].ds = dt[key].ds.wrl.georef.georeference(
crs=wrl.georef.get_default_projection()
)
dt["sweep_0"].ds.reflectivity.sel(range=slice(0, 5e4)).plot(
x="x",
y= "y",
cmap="ChaseSpectral",
vmax=60,
vmin=-10,
)
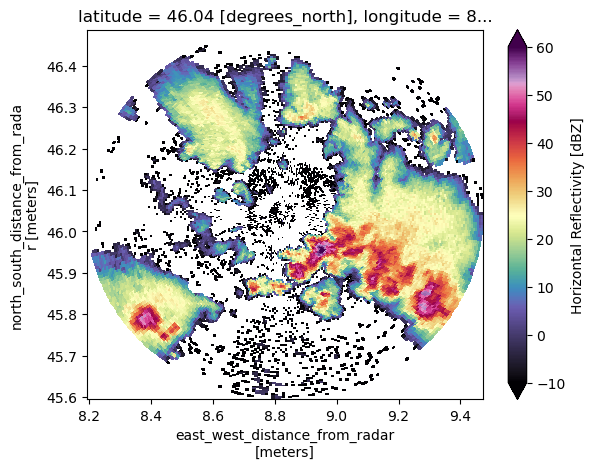
Xradar exporters¶
In xradar, exporters convert radar data into various formats for analysis or integration. Exporting is supported for recognized standards, including:
- CfRadial1
- CfRadial2
- ODIM
- Zarr
Although Zarr
is not a traditional standard format, it provides an analysis-ready, cloud-optimized format that enhances data accessibility and performance.
dt.to_zarr("tree.zarr", consolidated=True, mode="w")
dt_back = open_datatree("tree.zarr", engine="zarr",chunks={})
display(dt_back)
Summary¶
Xradar
is a Python library designed for working with radar data. It extends xarray to include radar-specific functionality, such as purpose-based accessors and georeferencing methods. It supports exporting data in various formats, including CfRadial1, CfRadial2, ODIM, and Zarr. xradar facilitates the analysis, visualization, and integration of radar data with other tools and systems.