Py-ART Basics
Overview
Within this notebook, we will cover:
General overview of Py-ART and its functionality
Reading data using Py-ART
An overview of the
pyart.Radar
objectCreate a Plot of our Radar Data
Prerequisites
Concepts |
Importance |
Notes |
---|---|---|
Helpful |
Basic features |
|
Helpful |
Basic plotting |
|
Helpful |
Basic arrays |
Time to learn: 45 minutes
Imports
import os
import warnings
import cartopy.crs as ccrs
import matplotlib.pyplot as plt
import pyart
from pyart.testing import get_test_data
warnings.filterwarnings('ignore')
## You are using the Python ARM Radar Toolkit (Py-ART), an open source
## library for working with weather radar data. Py-ART is partly
## supported by the U.S. Department of Energy as part of the Atmospheric
## Radiation Measurement (ARM) Climate Research Facility, an Office of
## Science user facility.
##
## If you use this software to prepare a publication, please cite:
##
## JJ Helmus and SM Collis, JORS 2016, doi: 10.5334/jors.119
An Overview of Py-ART
History of the Py-ART
Development began to address the needs of ARM with the acquisition of a number of new scanning cloud and precipitation radar as part of the American Recovery Act.
The project has since expanded to work with a variety of weather radars and a wider user base including radar researchers and climate modelers.
The software has been released on GitHub as open source software under a BSD license. Runs on Linux, OS X. It also runs on Windows with more limited functionality.
What can PyART Do?
Py-ART can be used for a variety of tasks from basic plotting to more complex processing pipelines. Specific uses for Py-ART include:
Reading radar data in a variety of file formats.
Creating plots and visualization of radar data.
Correcting radar moments while in antenna coordinates, such as:
Doppler unfolding/de-aliasing.
Attenuation correction.
Phase processing using a Linear Programming method.
Mapping data from one or multiple radars onto a Cartesian grid.
Performing retrievals.
Writing radial and Cartesian data to NetCDF files.
Reading in Data Using Py-ART
Reading data in using pyart.io.read
When reading in a radar file, we use the pyart.io.read
module.
pyart.io.read
can read a variety of different radar formats, such as Cf/Radial, LASSEN, and more.
The documentation on what formats can be read by Py-ART can be found here:
For most file formats listed on the page, using pyart.io.read
should suffice since Py-ART has the ability to automatically detect the file format.
Let’s check out what arguments arguments pyart.io.read()
takes in!
pyart.io.read?
Let’s use a sample data file from pyart
- which is cfradial format.
When we read this in, we get a pyart.Radar
object!
file = get_test_data('swx_20120520_0641.nc')
radar = pyart.io.read(file)
radar
Downloading file 'swx_20120520_0641.nc' from 'https://adc.arm.gov/pyart/example_data/swx_20120520_0641.nc' to '/github/home/.cache/pyart-datasets'.
<pyart.core.radar.Radar at 0x7fac1f4c1eb0>
Investigate the pyart.Radar
object
Within this pyart.Radar
object object are the actual data fields.
This is where data such as reflectivity and velocity are stored.
To see what fields are present we can add the fields and keys additions to the variable where the radar object is stored.
radar.fields.keys()
dict_keys(['corrected_reflectivity_horizontal', 'reflectivity_horizontal', 'recalculated_diff_phase', 'specific_attenuation', 'unf_dp_phase_shift', 'mean_doppler_velocity', 'diff_phase', 'rain_rate_A', 'norm_coherent_power', 'dp_phase_shift', 'diff_reflectivity', 'proc_dp_phase_shift', 'copol_coeff'])
Extract a sample data field
The fields are stored in a dictionary, each containing coordinates, units and more. All can be accessed by just adding the fields addition to the radar object variable.
For an individual field, we add a string in brackets after the fields addition to see the contents of that field.
Let’s take a look at 'corrected_reflectivity_horizontal'
, which is a common field to investigate.
print(radar.fields['corrected_reflectivity_horizontal'])
{'_FillValue': -9999.0, 'least_significant_digit': 2, 'units': 'dBZ', 'long_name': 'equivalent_reflectivity_factor', 'valid_min': -45.0, 'valid_max': 80.0, 'standard_name': 'equivalent_reflectivity_factor', 'data': masked_array(
data=[[-5.671875, 2.28125, -8.1171875, ..., --, -13.4765625, --],
[-5.6171875, 1.8984375, -10.0703125, ..., -2.6796875, -1.5390625,
--],
[-5.0390625, 2.625, -11.484375, ..., -8.984375, --, --],
...,
[-5.9375, 1.46875, -12.3203125, ..., --, --, --],
[-5.9609375, 1.53125, -12.84375, ..., --, --, --],
[-8.7890625, 2.9140625, -12.09375, ..., --, --, --]],
mask=[[False, False, False, ..., True, False, True],
[False, False, False, ..., False, False, True],
[False, False, False, ..., False, True, True],
...,
[False, False, False, ..., True, True, True],
[False, False, False, ..., True, True, True],
[False, False, False, ..., True, True, True]],
fill_value=-9999.0,
dtype=float32)}
We can go even further in the dictionary and access the actual reflectivity data.
We use add 'data'
at the end, which will extract the data array (which is a masked numpy array) from the dictionary.
reflectivity = radar.fields['corrected_reflectivity_horizontal']['data']
print(type(reflectivity), reflectivity)
<class 'numpy.ma.core.MaskedArray'> [[-5.671875 2.28125 -8.1171875 ... -- -13.4765625 --]
[-5.6171875 1.8984375 -10.0703125 ... -2.6796875 -1.5390625 --]
[-5.0390625 2.625 -11.484375 ... -8.984375 -- --]
...
[-5.9375 1.46875 -12.3203125 ... -- -- --]
[-5.9609375 1.53125 -12.84375 ... -- -- --]
[-8.7890625 2.9140625 -12.09375 ... -- -- --]]
Lets’ check the size of this array…
reflectivity.shape
(8800, 667)
This reflectivity data array, numpy array, is a two-dimensional array with dimensions:
Gates (number of samples away from the radar)
Rays (direction around the radar)
print(radar.nrays, radar.ngates)
8800 667
If we wanted to look the 300th ray, at the second gate, we would use something like the following:
print(reflectivity[300, 2])
-4.8046875
Plotting our Radar Data
An Overview of Py-ART Plotting Utilities
Now that we have loaded the data and inspected it, the next logical thing to do is to visualize the data! Py-ART’s visualization functionality is done through the objects in the pyart.graph module.
In Py-ART there are 4 primary visualization classes in pyart.graph:
Plotting grid data
Use the RadarMapDisplay with our data
For the this example, we will be using RadarMapDisplay
, using Cartopy to deal with geographic coordinates.
We start by creating a figure first.
fig = plt.figure(figsize=[10, 10])
<Figure size 720x720 with 0 Axes>
Once we have a figure, let’s add our RadarMapDisplay
fig = plt.figure(figsize=[10, 10])
display = pyart.graph.RadarMapDisplay(radar)
<Figure size 720x720 with 0 Axes>
Adding our map display without specifying a field to plot won’t do anything we need to specifically add a field to field using .plot_ppi_map()
display.plot_ppi_map('corrected_reflectivity_horizontal')
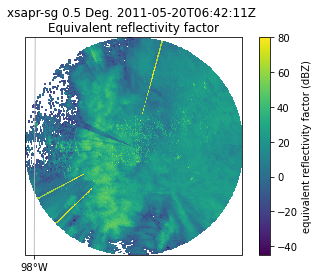
By default, it will plot the elevation scan, the the default colormap from Matplotlib
… let’s customize!
We add the following arguements:
sweep=3
- The fourth elevation scan (since we are using Python indexing)vmin=-20
- Minimum value for our plotted field/colorbarvmax=60
- Maximum value for our plotted field/colorbarprojection=ccrs.PlateCarree()
- Cartopy latitude/longitude coordinate systemcmap='pyart_HomeyerRainbow'
- Colormap to use, selecting one provided by PyART
fig = plt.figure(figsize=[12, 12])
display = pyart.graph.RadarMapDisplay(radar)
display.plot_ppi_map('corrected_reflectivity_horizontal',
sweep=3,
vmin=-20,
vmax=60,
projection=ccrs.PlateCarree(),
cmap='pyart_HomeyerRainbow')
plt.show()
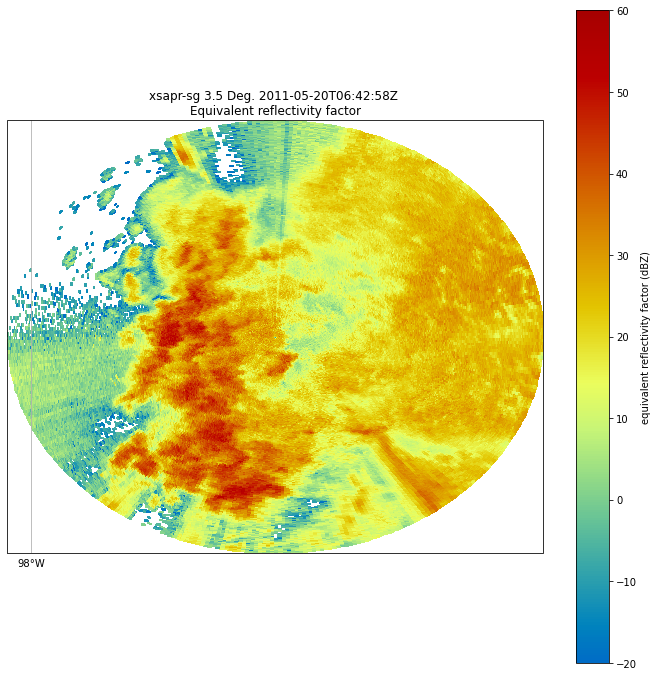
You can change many parameters in the graph by changing the arguments to plot_ppi_map. As you can recall from earlier. simply view these arguments in a Jupyter notebook by typing:
display.plot_ppi_map?
For example, let’s change the colormap to something different
fig = plt.figure(figsize=[12, 12])
display = pyart.graph.RadarMapDisplay(radar)
display.plot_ppi_map('corrected_reflectivity_horizontal',
sweep=3,
vmin=-20,
vmax=60,
projection=ccrs.PlateCarree(),
cmap='pyart_Carbone42')
plt.show()
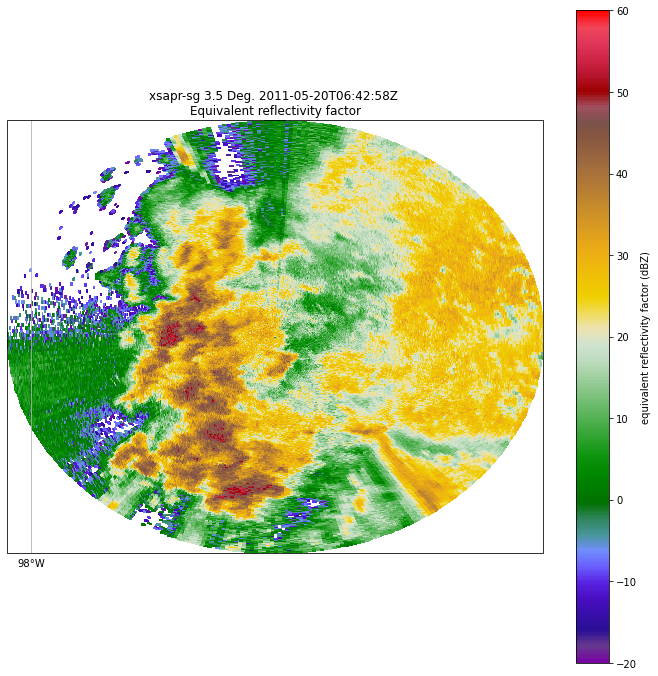
Or, let’s view a different elevation scan! To do this, change the sweep parameter in the plot_ppi_map function.
fig = plt.figure(figsize=[12, 12])
display = pyart.graph.RadarMapDisplay(radar)
display.plot_ppi_map('corrected_reflectivity_horizontal',
sweep=0,
vmin=-20,
vmax=60,
projection=ccrs.PlateCarree(),
cmap='pyart_Carbone42')
plt.show()
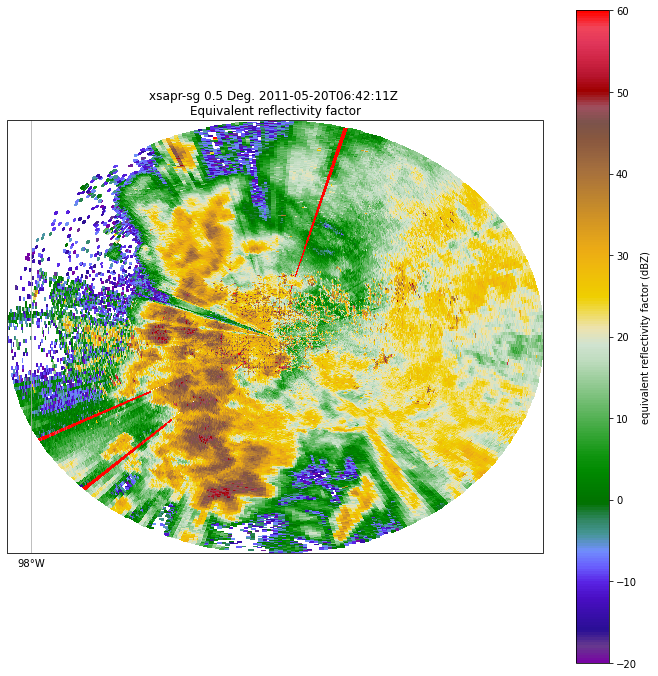
Let’s take a look at a different field - for example, correlation coefficient (corr_coeff
)
fig = plt.figure(figsize=[12, 12])
display = pyart.graph.RadarMapDisplay(radar)
display.plot_ppi_map('copol_coeff',
sweep=0,
vmin=0.8,
vmax=1.,
projection=ccrs.PlateCarree(),
cmap='pyart_Carbone42')
plt.show()
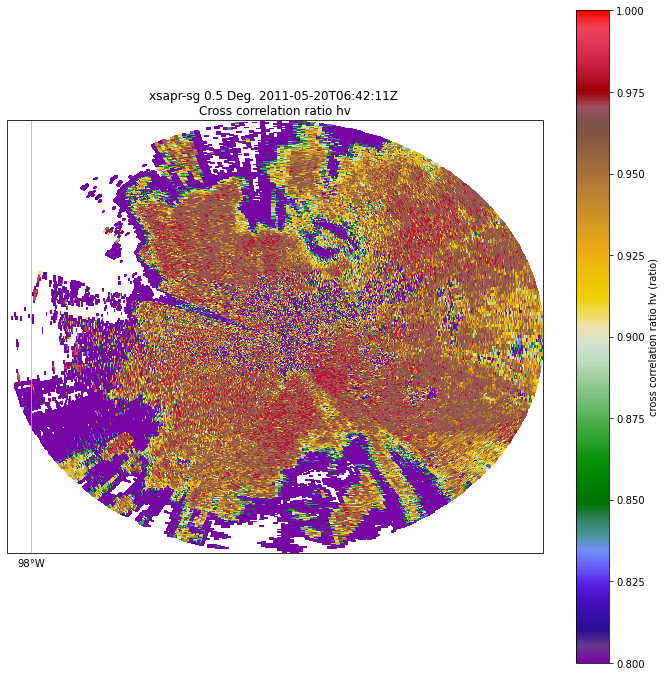
Summary
Within this notebook, we covered the basics of working with radar data using pyart
, including:
Reading in a file using
pyart.io
Investigating the
Radar
objectVisualizing radar data using the
RadarMapDisplay