Doppler Velocity Dealiasing with Py-ART and BALTRAD
In this notebook Doppler Velocity data from the ARM C-band SAPR radar is read using Py-ART and dealiased using BALTRAD.
%matplotlib inline
Import the necessary modules
import pyart
import baltrad_pyart_bridge as bridge # routines to pass data from Py-ART to BALTRAD
import _dealias # BALTRAD's dealiasing module
## You are using the Python ARM Radar Toolkit (Py-ART), an open source
## library for working with weather radar data. Py-ART is partly
## supported by the U.S. Department of Energy as part of the Atmospheric
## Radiation Measurement (ARM) Climate Research Facility, an Office of
## Science user facility.
##
## If you use this software to prepare a publication, please cite:
##
## JJ Helmus and SM Collis, JORS 2016, doi: 10.5334/jors.119
/srv/conda/envs/notebook/lib/python3.9/site-packages/requests/__init__.py:102: RequestsDependencyWarning: urllib3 (1.26.8) or chardet (5.2.0)/charset_normalizer (2.0.10) doesn't match a supported version!
warnings.warn("urllib3 ({}) or chardet ({})/charset_normalizer ({}) doesn't match a supported "
Read in the data using Py-ART
radar = pyart.io.read('data/sgpcsaprppi_20110520095101.nc')
Examine the velocity data using Py-ART Display object.
display = pyart.graph.RadarDisplay(radar)
nyquist_velocity = radar.instrument_parameters['nyquist_velocity']['data'][0]
display.plot_ppi('velocity', 1, colorbar_label='m/s',
vmin=-nyquist_velocity, vmax=nyquist_velocity)
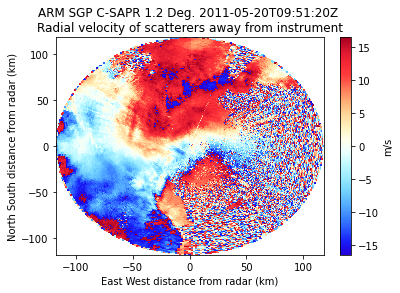
Convert the radar data into a RaveIO object with the velocity data having the correct name.
vel_data = radar.fields['velocity']['data']
radar.add_field_like('velocity', 'VRAD', vel_data)
rio = bridge.radar2raveio(radar)
Perform Doppler velocity dealiasing using BALTRAD.
ret = _dealias.dealias(rio.object)
print("This first scan is dealiased:"), _dealias.dealiased(rio.object.getScan(0))
This first scan is dealiased:
(None, False)
Add the dealiased velocity field to the origin Py-ART radar object.
temp = bridge.raveio2radar(rio)
if 'dealiased_velocity' in radar.fields:
radar.fields.pop('dealiased_velocity')
radar.add_field_like('velocity', 'dealiased_velocity', temp.fields['VRAD']['data'])
Plot the dealiased velocities.
display.plot_ppi('dealiased_velocity', 1, colorbar_label='m/s',
vmin=-2*nyquist_velocity, vmax=2*nyquist_velocity)
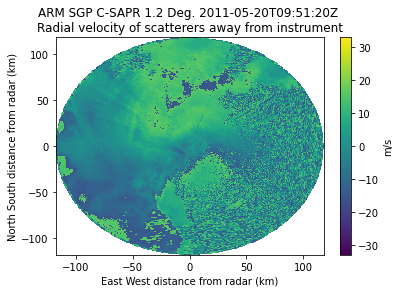