Py-ART Gridding
Overview
Within this notebook, we will cover:
What is gridding and why is it important?
An overview of gridding with Py-ART
How to choose a gridding routine
Gridding multiple radars to the same grid
Prerequisites
Concepts |
Importance |
Notes |
---|---|---|
Helpful |
Basic features |
|
Helpful |
Basic features |
|
Helpful |
Basic plotting |
|
Helpful |
Basic arrays |
Time to learn: 45 minutes
Imports
import os
import warnings
import cartopy.crs as ccrs
import matplotlib.pyplot as plt
import pyart
from pyart.testing import get_test_data
warnings.filterwarnings('ignore')
## You are using the Python ARM Radar Toolkit (Py-ART), an open source
## library for working with weather radar data. Py-ART is partly
## supported by the U.S. Department of Energy as part of the Atmospheric
## Radiation Measurement (ARM) Climate Research Facility, an Office of
## Science user facility.
##
## If you use this software to prepare a publication, please cite:
##
## JJ Helmus and SM Collis, JORS 2016, doi: 10.5334/jors.119
/srv/conda/envs/notebook/lib/python3.9/site-packages/requests/__init__.py:102: RequestsDependencyWarning: urllib3 (1.26.8) or chardet (5.2.0)/charset_normalizer (2.0.10) doesn't match a supported version!
warnings.warn("urllib3 ({}) or chardet ({})/charset_normalizer ({}) doesn't match a supported "
What is gridding and why is it important?
Antenna vs. Cartesian Coordinates
Radar data, by default, is stored in a polar (or antenna) coordinate system, with the data coordinates stored as an angle (ranging from 0 to 360 degrees with 0 == North), and a radius from the radar, and an elevation which is the angle between the ground and the ground.
This format can be challenging to plot, since it is scan/radar specific. Also, it can make comparing with model data, which is on a lat/lon grid, challenging since one would need to transform the model daa cartesian coordinates to polar/antenna coordiantes.
Fortunately, PyART has a variety of gridding routines, which can be used to grid your data to a Cartesian grid. Once it is in this new grid, one can easily slice/dice the dataset, and compare to other data sources.
Why is Gridding Important?
Gridding is essential to combining multiple data sources (ex. multiple radars), and comparing to other data sources (ex. model data). There are also decisions that are made during the gridding process that have a large impact on the regridded data - for example:
What resolution should my grid be?
Which interpolation routine should I use?
How smooth should my interpolated data be?
While there is not always a right or wrong answer, it is important to understand the options available, and document which routine you used with your data! Also - experiment with different options and choose the best for your use case!
An overview of gridding with Py-ART
Let’s dig into the regridding process with PyART!
Read in and Visualize a Test Dataset
Let’s start with the same file used in the previous notebook (PyART Basics
), which is a radar file from Northern Oklahoma.
file = get_test_data('swx_20120520_0641.nc')
radar = pyart.io.read(file)
Let’s plot up quick look of reflectivity, at the lowest elevation scan (closest to the ground)
fig = plt.figure(figsize=[12, 12])
display = pyart.graph.RadarDisplay(radar)
display.plot_ppi('corrected_reflectivity_horizontal',
cmap='pyart_HomeyerRainbow')
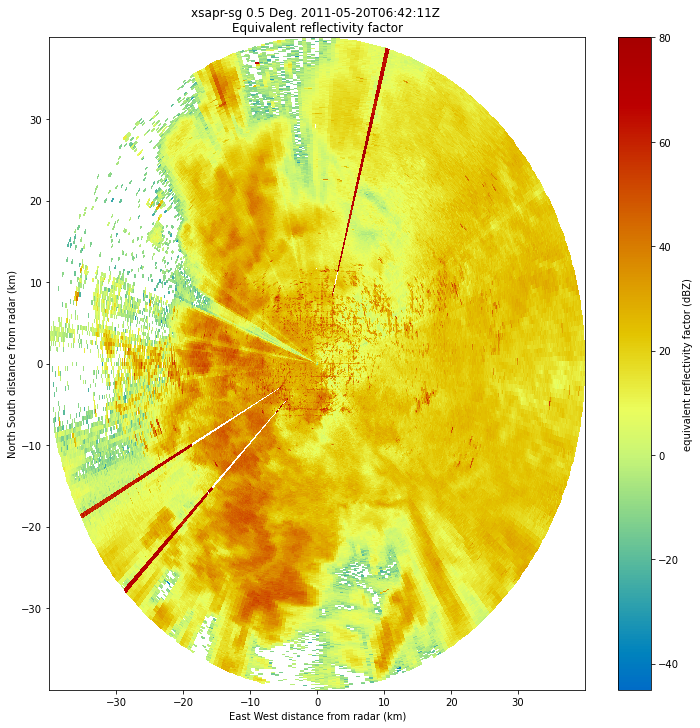
As mentioned before, the dataset is currently in the antenna coordinate system measured as distance from the radar
Setup our Gridding Routine with pyart.map.grid_from_radars()
Py-ART has the Grid object which has characteristics similar to that of the Radar object, except that the data are stored in Cartesian coordinates instead of the radar’s antenna coordinates.
pyart.core.Grid?
We can transform our data into this grid object, from the radars, using pyart.map.grid_from_radars()
.
Beforing gridding our data, we need to make a decision about the desired grid resolution and extent. For example, one might imagine a grid configuration of:
Grid extent/limits
20 km in the x-direction (north/south)
20 km in the y-direction (west/east)
15 km in the z-direction (vertical)
500 m spatial resolution
The pyart.map.grid_from_radars()
function takes the grid shape and grid limits as input, with the order (z, y, x)
.
Let’s setup our configuration, setting our grid extent first, with the distance measured in meters
z_grid_limits = (500.,15_000.)
y_grid_limits = (-20_000.,20_000.)
x_grid_limits = (-20_000.,20_000.)
Now that we have our grid limits, we can set our desired resolution (again, in meters)
grid_resolution = 500
Let’s compute our grid shape - using the extent and resolution to compute the number of grid points in each direction.
def compute_number_of_points(extent, resolution):
return int((extent[1] - extent[0])/resolution)
Now that we have a helper function to compute this, let’s apply it to our vertical dimension
z_grid_points = compute_number_of_points(z_grid_limits, grid_resolution)
z_grid_points
29
We can apply this to the horizontal (x, y) dimensions as well.
x_grid_points = compute_number_of_points(x_grid_limits, grid_resolution)
y_grid_points = compute_number_of_points(y_grid_limits, grid_resolution)
print(z_grid_points,
y_grid_points,
x_grid_points)
29 80 80
Use our configuration to grid the data!
Now that we have the grid shape and grid limits, let’s grid up our radar!
grid = pyart.map.grid_from_radars(radar,
grid_shape=(z_grid_points,
y_grid_points,
x_grid_points),
grid_limits=(z_grid_limits,
y_grid_limits,
x_grid_limits),
)
grid
<pyart.core.grid.Grid at 0x7ff615d8b730>
We now have a pyart.core.Grid
object!
Plot up the Grid Object
Plot a horizontal view of the data
We can use the GridMapDisplay
from pyart.graph
to visualize our regridded data, starting with a horizontal view (slice along a single vertical level)
display = pyart.graph.GridMapDisplay(grid)
display.plot_grid('corrected_reflectivity_horizontal',
level=0,
vmin=-20,
vmax=60,
cmap='pyart_HomeyerRainbow')
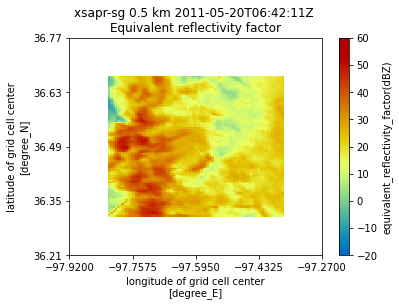
Plot a Latitudinal Slice
We can also slice through a single latitude or longitude!
display.plot_latitude_slice('corrected_reflectivity_horizontal',
lat=36.5,
vmin=-20,
vmax=60,
cmap='pyart_HomeyerRainbow')
plt.xlim([-20, 20]);
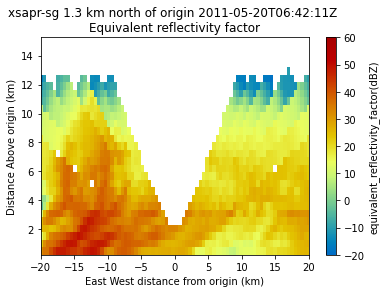
Plot with Xarray
Another neat feature of the Grid
object is that we can transform it to an xarray.Dataset
!
ds = grid.to_xarray()
ds
<xarray.Dataset> Dimensions: (time: 1, z: 29, y: 80, x: 80) Coordinates: * time (time) object 2011-05-20 06:42:11 * z (z) float64 500.0 1.018e+03 ... 1.5e+04 lat (y) float64 36.31 36.32 ... 36.67 36.67 lon (x) float64 -97.82 -97.81 ... -97.37 * y (y) float64 -2e+04 -1.949e+04 ... 2e+04 * x (x) float64 -2e+04 -1.949e+04 ... 2e+04 Data variables: (12/14) dp_phase_shift (time, z, y, x) float32 153.0 ... nan norm_coherent_power (time, z, y, x) float32 0.2725 ... 0.0... mean_doppler_velocity (time, z, y, x) float32 -11.43 ... 1.22 specific_attenuation (time, z, y, x) float32 0.007137 ... 0.0 proc_dp_phase_shift (time, z, y, x) float32 67.05 ... 42.07 corrected_reflectivity_horizontal (time, z, y, x) float32 11.35 ... nan ... ... recalculated_diff_phase (time, z, y, x) float32 0.3767 ... 0.0... copol_coeff (time, z, y, x) float32 0.5355 ... nan unf_dp_phase_shift (time, z, y, x) float32 66.88 ... 42.07 diff_reflectivity (time, z, y, x) float32 0.0 0.0 ... 0.0 rain_rate_A (time, z, y, x) float32 0.7812 ... 0.0 ROI (time, z, y, x) float32 765.6 ... 1.49...
Now, our plotting routine is a one-liner, starting with the horizontal slice:
ds.isel(z=0).corrected_reflectivity_horizontal.plot(cmap='pyart_HomeyerRainbow',
vmin=-20,
vmax=60);
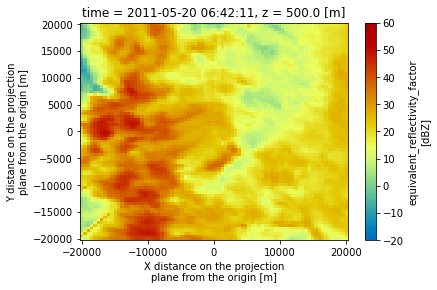
And a vertical slice at a given y dimension (latitude)
ds.sel(y=1300,
method='nearest').corrected_reflectivity_horizontal.plot(cmap='pyart_HomeyerRainbow',
vmin=-20,
vmax=60);
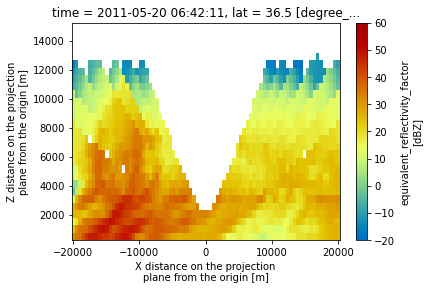
Summary
Within this notebook, we covered the basics of gridding radar data using pyart
, including:
What we mean by gridding and why is it matters
Configuring your gridding routine
Visualize gridded radar data