ERAD 2022 Open Source Workshop
LROSE workflow - combining 3 NEXRAD radars, computing PID and Echo Type
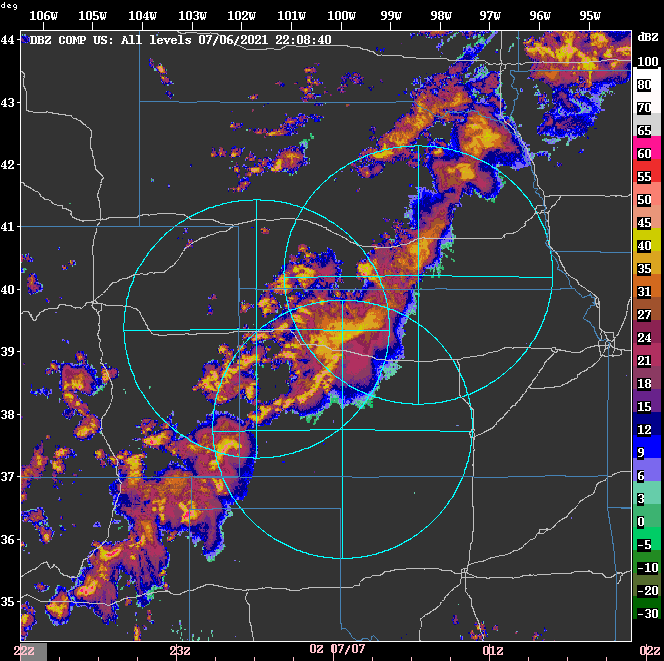
The case we will use is from 2021/07/06 22:00 UTC, when a series of MCSs passed through the NW region of Kansas in the USA.
We will combine the reflectivity of 3 NEXRAD radars into a mini-mosaic:
KGLD - Goodland, Kansas
KUEX - Hastings, Nebraska
KDDC - Dodge City, Kansas
We will download the following data sets from the cloud:
raw NEXRAD files for KGLD, KUEX and KDDC, 2021/07/06 from 22:00 UTC to 22:30 UTC
RUC model output for Kansas region, CF-NetCDF format, from 2021/07/06 at 23:00 UTC
The model data will provide a temperature profile for computing PID and Echo Type.
The workflow is as follows:
download the data into
/tmp/lrose_data/nexrad_mosaic
.run LROSE app RadxConvert, to convert raw NEXRAD files to CfRadial.
plot an example PPI from KGLD using PyArt.
read in temperature data from RUC file, plot cross sections using Matplotlib.
run the LROSE app Mdv2SoundingSpdb to derive the temperature profile for each radar site from the RUC data file, and store in SPDB (a simple time-indexed data base).
run the LROSE app RadxRate to compute precipition rate and Particle ID (PID).
plot KDP, PID and precipitation rate for an example PPI.
run the LROSE app Radx2Grid to convert the polar CfRadial files into Cartesian coordinates.
run the LROSE app MdvMerge2 to merge the Cartesian files from the 3 radars into a reflectivity mini-mosaic.
plot selected views of the reflectivity mosaic, using Matplotlib.
run the LROSE app Ecco to compute the convective/stratiform partition using the reflectivity mosaic and the RUC temperature profile.
plot the results of Ecco using Matplotlib.
We will use the following parameter files for the LROSE applications:
params/RadxConvert.nexrad - convert raw NEXRAD files to CfRadial.
params/Mdv2SoundingSpdb.ruc - create temperature profiles for each radar from RUC model temperature.
params/RadxRate.nexrad - computes KDP, PID and precipition rate.
params/kdp_params.nexrad - used by RadxRate to compute KDP.
params/pid_params.nexrad - used by RadxRate to compute PID.
params/pid_thresholds.nexrad - used by RadxRate to compute PID.
params/rate_params.nexrad - used by RadxRate to compute precipitation rate.
params/Ecco.nexrad_mosaic - used by Ecco to compute echo type classifications.
After the download step, the input files will be in:
/tmp/lrose_data/nexrad_mosaic/raw/KGLD
/tmp/lrose_data/nexrad_mosaic/raw/KUEX
/tmp/lrose_data/nexrad_mosaic/raw/KDDC
/tmp/lrose_data/nexrad_mosaic/mdv/ruc
The output files will be stored in:
/tmp/lrose_data/nexrad_mosaic/cfradial (polar data)
/tmp/lrose_data/nexrad_mosaic/mdv (Cartesian data)
/tmp/lrose_data/nexrad_mosaic/spdb (temperature profile per radar)
Initialize python
#
# Extra packages to be added to anaconda3 standard packages for this notebook:
#
# conda update --all
# conda install cartopy netCDF4
# conda install -c conda-forge arm_pyart
#
import warnings
warnings.filterwarnings('ignore')
import os
import datetime
import pytz
import math
import numpy as np
import matplotlib as mp
import matplotlib.pyplot as plt
import matplotlib.ticker as plticker
from matplotlib.lines import Line2D
import cartopy
import cartopy.crs as ccrs
import cartopy.io.shapereader as shpreader
import cartopy.geodesic as cgds
from cartopy.mpl.gridliner import LONGITUDE_FORMATTER, LATITUDE_FORMATTER
from cartopy import feature as cfeature
import shapely
import netCDF4 as nc
import pyart
# Set data dir in environment variable
os.environ['NEXRAD_DATA_DIR'] = '/tmp/lrose_data/nexrad_mosaic'
nexradDataDir = os.environ['NEXRAD_DATA_DIR']
print('====>> nexradDataDir: ', nexradDataDir)
## You are using the Python ARM Radar Toolkit (Py-ART), an open source
## library for working with weather radar data. Py-ART is partly
## supported by the U.S. Department of Energy as part of the Atmospheric
## Radiation Measurement (ARM) Climate Research Facility, an Office of
## Science user facility.
##
## If you use this software to prepare a publication, please cite:
##
## JJ Helmus and SM Collis, JORS 2016, doi: 10.5334/jors.119
====>> nexradDataDir: /tmp/lrose_data/nexrad_mosaic
Download data sets from web
# Download input data sets
#
# 1. NEXRAD raw data files for KGLD, KDDC and KUEX
# 2. RUC model data for temperature profile, Kansas and surroundings
#
# These will be put in:
# ${NEXRAD_DATA_DIR}
#
# ensure the data dir exists and is clean
!/bin/rm -rf ${NEXRAD_DATA_DIR}
!mkdir -p ${NEXRAD_DATA_DIR}
# download the data from github
print("====>> Downloading data tar files into dir: ", nexradDataDir)
!cd ${NEXRAD_DATA_DIR}; wget http://front.eol.ucar.edu/data/notebooks/nexrad_mosaic/nexrad_mosaic.KGLD.20210706_220000.tgz
!cd ${NEXRAD_DATA_DIR}; wget http://front.eol.ucar.edu/data/notebooks/nexrad_mosaic/nexrad_mosaic.KDDC.20210706_220000.tgz
!cd ${NEXRAD_DATA_DIR}; wget http://front.eol.ucar.edu/data/notebooks/nexrad_mosaic/nexrad_mosaic.KUEX.20210706_220000.tgz
!cd ${NEXRAD_DATA_DIR}; wget http://front.eol.ucar.edu/data/notebooks/nexrad_mosaic/nexrad_mosaic.ruc.20210706_220000.tgz
# !cd ${NEXRAD_DATA_DIR}; wget https://raw.githubusercontent.com/NCAR/lrose-data-examples/master/notebooks/nexrad_mosaic/nexrad_mosaic.ruc.20210706_220000.tgz
# !cd ${NEXRAD_DATA_DIR}; wget https://raw.githubusercontent.com/NCAR/lrose-data-examples/master/notebooks/nexrad_mosaic/nexrad_mosaic.KGLD.20210706_220000.tgz
# !cd ${NEXRAD_DATA_DIR}; wget https://raw.githubusercontent.com/NCAR/lrose-data-examples/master/notebooks/nexrad_mosaic/nexrad_mosaic.KDDC.20210706_220000.tgz
# !cd ${NEXRAD_DATA_DIR}; wget https://raw.githubusercontent.com/NCAR/lrose-data-examples/master/notebooks/nexrad_mosaic/nexrad_mosaic.KUEX.20210706_220000.tgz
# extract the data from the tar files
print("====>> The following data files are unpacked in dir: ", nexradDataDir)
!cd ${NEXRAD_DATA_DIR}; tar xvfz nexrad_mosaic.ruc.20210706_220000.tgz
!cd ${NEXRAD_DATA_DIR}; tar xvfz nexrad_mosaic.KGLD.20210706_220000.tgz
!cd ${NEXRAD_DATA_DIR}; tar xvfz nexrad_mosaic.KDDC.20210706_220000.tgz
!cd ${NEXRAD_DATA_DIR}; tar xvfz nexrad_mosaic.KUEX.20210706_220000.tgz
# clean up
!cd ${NEXRAD_DATA_DIR}; /bin/rm -f *tgz
====>> Downloading data tar files into dir: /tmp/lrose_data/nexrad_mosaic
--2024-02-26 08:55:57-- http://front.eol.ucar.edu/data/notebooks/nexrad_mosaic/nexrad_mosaic.KGLD.20210706_220000.tgz
Resolving front.eol.ucar.edu (front.eol.ucar.edu)...
128.117.43.125
Connecting to front.eol.ucar.edu (front.eol.ucar.edu)|128.117.43.125|:80...
connected.
HTTP request sent, awaiting response...
200 OK
Length: 74762580 (71M) [application/x-gzip]
Saving to: ‘nexrad_mosaic.KGLD.20210706_220000.tgz’
nexrad_mo 0%[ ] 0 --.-KB/s
nexrad_mos 1%[ ] 969.98K 4.68MB/s
nexrad_mosa 22%[===> ] 16.26M 40.4MB/s
nexrad_mosai 47%[========> ] 34.09M 56.5MB/s
nexrad_mosaic 72%[=============> ] 51.60M 64.2MB/s
nexrad_mosaic. 97%[==================> ] 69.49M 69.2MB/s
nexrad_mosaic.KGLD. 100%[===================>] 71.30M 69.0MB/s in 1.0s
2024-02-26 08:55:59 (69.0 MB/s) - ‘nexrad_mosaic.KGLD.20210706_220000.tgz’ saved [74762580/74762580]
--2024-02-26 08:55:59-- http://front.eol.ucar.edu/data/notebooks/nexrad_mosaic/nexrad_mosaic.KDDC.20210706_220000.tgz
Resolving front.eol.ucar.edu (front.eol.ucar.edu)...
128.117.43.125
Connecting to front.eol.ucar.edu (front.eol.ucar.edu)|128.117.43.125|:80... connected.
HTTP request sent, awaiting response...
200 OK
Length: 69853288 (67M) [application/x-gzip]
Saving to: ‘nexrad_mosaic.KDDC.20210706_220000.tgz’
nexrad_mo 0%[ ] 0 --.-KB/s
nexrad_mos 1%[ ] 857.04K 4.03MB/s
nexrad_mosa 26%[====> ] 17.61M 43.2MB/s
nexrad_mosai 56%[==========> ] 37.51M 60.5MB/s
nexrad_mosaic 86%[================> ] 57.47M 70.0MB/s
nexrad_mosaic.KDDC. 100%[===================>] 66.62M 72.5MB/s in 0.9s
2024-02-26 08:56:00 (72.5 MB/s) - ‘nexrad_mosaic.KDDC.20210706_220000.tgz’ saved [69853288/69853288]
--2024-02-26 08:56:01-- http://front.eol.ucar.edu/data/notebooks/nexrad_mosaic/nexrad_mosaic.KUEX.20210706_220000.tgz
Resolving front.eol.ucar.edu (front.eol.ucar.edu)... 128.117.43.125
Connecting to front.eol.ucar.edu (front.eol.ucar.edu)|128.117.43.125|:80... connected.
HTTP request sent, awaiting response...
200 OK
Length: 59062156 (56M) [application/x-gzip]
Saving to: ‘nexrad_mosaic.KUEX.20210706_220000.tgz’
nexrad_mo 0%[ ] 0 --.-KB/s
nexrad_mos 1%[ ] 577.20K 2.61MB/s
nexrad_mosa 2%[ ] 1.66M 3.99MB/s
nexrad_mosai 4%[ ] 2.75M 4.30MB/s
nexrad_mosaic 7%[> ] 3.96M 4.72MB/s
nexrad_mosaic. 9%[> ] 5.14M 4.84MB/s
nexrad_mosaic.K 11%[=> ] 6.45M 5.10MB/s
nexrad_mosaic.KU 13%[=> ] 7.70M 5.19MB/s
nexrad_mosaic.KUE 16%[==> ] 9.03M 5.36MB/s
nexrad_mosaic.KUEX 18%[==> ] 10.33M 5.48MB/s
nexrad_mosaic.KUEX. 20%[===> ] 11.67M 5.54MB/s
exrad_mosaic.KUEX.2 23%[===> ] 13.07M 5.67MB/s
xrad_mosaic.KUEX.20 25%[====> ] 14.49M 5.78MB/s
rad_mosaic.KUEX.202 28%[====> ] 15.90M 5.83MB/s
ad_mosaic.KUEX.2021 30%[=====> ] 17.40M 5.95MB/s
d_mosaic.KUEX.20210 33%[=====> ] 18.95M 6.06MB/s eta 6s
_mosaic.KUEX.202107 36%[======> ] 20.48M 6.12MB/s eta 6s
mosaic.KUEX.2021070 39%[======> ] 22.11M 6.46MB/s eta 6s
osaic.KUEX.20210706 42%[=======> ] 23.77M 6.62MB/s eta 6s
saic.KUEX.20210706_ 45%[========> ] 25.40M 6.75MB/s eta 6s
aic.KUEX.20210706_2 48%[========> ] 27.18M 6.94MB/s eta 4s
ic.KUEX.20210706_22 51%[=========> ] 28.92M 7.10MB/s eta 4s
c.KUEX.20210706_220 54%[=========> ] 30.68M 7.29MB/s eta 4s
.KUEX.20210706_2200 57%[==========> ] 32.53M 7.47MB/s eta 4s
KUEX.20210706_22000 60%[===========> ] 34.35M 7.62MB/s eta 4s
UEX.20210706_220000 64%[===========> ] 36.29M 7.83MB/s eta 3s
EX.20210706_220000. 67%[============> ] 38.23M 8.01MB/s eta 3s
X.20210706_220000.t 71%[=============> ] 40.23M 8.20MB/s eta 3s
.20210706_220000.tg 75%[==============> ] 42.26M 8.42MB/s eta 3s
20210706_220000.tgz 78%[==============> ] 44.28M 8.59MB/s eta 3s
0210706_220000.tgz 82%[===============> ] 46.41M 8.78MB/s eta 1s
210706_220000.tgz 86%[================> ] 48.48M 8.94MB/s eta 1s
10706_220000.tgz 89%[================> ] 50.61M 9.15MB/s eta 1s
0706_220000.tgz 93%[=================> ] 52.85M 9.35MB/s eta 1s
706_220000.tgz 97%[==================> ] 55.04M 9.51MB/s eta 1s
nexrad_mosaic.KUEX. 100%[===================>] 56.33M 9.64MB/s in 7.2s
2024-02-26 08:56:08 (7.86 MB/s) - ‘nexrad_mosaic.KUEX.20210706_220000.tgz’ saved [59062156/59062156]
--2024-02-26 08:56:08-- http://front.eol.ucar.edu/data/notebooks/nexrad_mosaic/nexrad_mosaic.ruc.20210706_220000.tgz
Resolving front.eol.ucar.edu (front.eol.ucar.edu)...
128.117.43.125
Connecting to front.eol.ucar.edu (front.eol.ucar.edu)|128.117.43.125|:80...
connected.
HTTP request sent, awaiting response... 200 OK
Length: 4039462 (3.9M) [application/x-gzip]
Saving to: ‘nexrad_mosaic.ruc.20210706_220000.tgz’
nexrad_mo 0%[ ] 0 --.-KB/s
nexrad_mos 9%[> ] 389.20K 1.80MB/s
nexrad_mosa 30%[=====> ] 1.18M 2.72MB/s
nexrad_mosai 52%[=========> ] 2.02M 3.07MB/s
nexrad_mosaic 75%[==============> ] 2.90M 3.30MB/s
nexrad_mosaic. 99%[==================> ] 3.82M 3.48MB/s
nexrad_mosaic.ruc.2 100%[===================>] 3.85M 3.50MB/s in 1.1s
2024-02-26 08:56:09 (3.50 MB/s) - ‘nexrad_mosaic.ruc.20210706_220000.tgz’ saved [4039462/4039462]
====>> The following data files are unpacked in dir: /tmp/lrose_data/nexrad_mosaic
mdv/
mdv/ruc/
mdv/ruc/20210706/
mdv/ruc/20210706/20210706_230000.mdv.cf.nc
raw/KGLD/
raw/KGLD/20210706/
raw/KGLD/20210706/KGLD20210706_220003_V06
raw/KGLD/20210706/KGLD20210706_220448_V06
raw/KGLD/20210706/KGLD20210706_220935_V06
raw/KGLD/20210706/KGLD20210706_221420_V06
raw/KGLD/20210706/KGLD20210706_221906_V06
raw/KGLD/20210706/KGLD20210706_222350_V06
raw/KGLD/20210706/KGLD20210706_222834_V06
raw/KDDC/
raw/KDDC/20210706/
raw/KDDC/20210706/KDDC20210706_220000_V06
raw/KDDC/20210706/KDDC20210706_220430_V06
raw/KDDC/20210706/KDDC20210706_220921_V06
raw/KDDC/20210706/KDDC20210706_221600_V06
raw/KDDC/20210706/KDDC20210706_222051_V06
raw/KDDC/20210706/KDDC20210706_222533_V06
raw/KUEX/
raw/KUEX/20210706/
raw/KUEX/20210706/KUEX20210706_220249_V06
raw/KUEX/20210706/KUEX20210706_220723_V06
raw/KUEX/20210706/KUEX20210706_221204_V06
raw/KUEX/20210706/KUEX20210706_221633_V06
raw/KUEX/20210706/KUEX20210706_222102_V06
raw/KUEX/20210706/KUEX20210706_222531_V06
Notes on LROSE Parameter files
All LROSE applications have a detailed parameter file, which is read in at startup.
The parameters allow the user to control the processing in the LROSE apps.
To generate a default parameter file, you use the -print_params option for the app.
For example, for RadxConvert you would use:
RadxConvert -print_params > RadxConvert.nexrad
and then edit RadxConvert.nexrad appropriately.
At runtime you would use:
RadxConvert -params RadxConvert.nexrad ... etc ...
View the RadxConvert parameter file
Note that we can use environment variables in the parameter files.
Environment variables are inserted using the format:
$(env_var_name)
For example:
input_dir = "$(NEXRAD_DATA_DIR)/raw/$(RADAR_NAME)";
# View the param file
!cat ./params/RadxConvert.nexrad
/**********************************************************************
* TDRP params for RadxConvert
**********************************************************************/
//======================================================================
//
// Converts files between CfRadial and other radial formats.
//
//======================================================================
//======================================================================
//
// DEBUGGING.
//
//======================================================================
///////////// debug ///////////////////////////////////
//
// Debug option.
// If set, debug messages will be printed appropriately.
//
// Type: enum
// Options:
// DEBUG_OFF
// DEBUG_NORM
// DEBUG_VERBOSE
// DEBUG_EXTRA
//
debug = DEBUG_OFF;
///////////// instance ////////////////////////////////
//
// Program instance for process registration.
// This application registers with procmap. This is the instance used
// for registration.
// Type: string
//
instance = "$(RADAR_NAME)";
//======================================================================
//
// DATA INPUT.
//
//======================================================================
///////////// input_dir ///////////////////////////////
//
// Input directory for searching for files.
// Files will be searched for in this directory.
// Type: string
//
input_dir = "$(NEXRAD_DATA_DIR)/raw/$(RADAR_NAME)";
///////////// mode ////////////////////////////////////
//
// Operating mode.
// In REALTIME mode, the program waits for a new input file. In ARCHIVE
// mode, it moves through the data between the start and end times set
// on the command line. In FILELIST mode, it moves through the list of
// file names specified on the command line. Paths (in ARCHIVE mode, at
// least) MUST contain a day-directory above the data file --
// ./data_file.ext will not work as a file path, but
// ./yyyymmdd/data_file.ext will.
//
// Type: enum
// Options:
// REALTIME
// ARCHIVE
// FILELIST
//
mode = ARCHIVE;
///////////// max_realtime_data_age_secs //////////////
//
// Maximum age of realtime data (secs).
// Only data less old than this will be used.
// Type: int
//
max_realtime_data_age_secs = 300;
///////////// latest_data_info_avail //////////////////
//
// Is _latest_data_info file available?.
// If TRUE, will watch the latest_data_info file. If FALSE, will scan
// the input directory for new files.
// Type: boolean
//
latest_data_info_avail = FALSE;
///////////// search_recursively //////////////////////
//
// Option to recurse to subdirectories while looking for new files.
// If TRUE, all subdirectories with ages less than max_dir_age will be
// searched. This may take considerable CPU, so be careful in its use.
// Only applies if latest_data_info_avail is FALSE.
// Type: boolean
//
search_recursively = TRUE;
///////////// max_recursion_depth /////////////////////
//
// Maximum depth for recursive directory scan.
// Only applies search_recursively is TRUE. This is the max depth, below
// input_dir, to which the recursive directory search will be carried
// out. A depth of 0 will search the top-level directory only. A depth
// of 1 will search the level below the top directory, etc.
// Type: int
//
max_recursion_depth = 5;
///////////// wait_between_checks /////////////////////
//
// Sleep time between checking directory for input - secs.
// If a directory is large and files do not arrive frequently, set this
// to a higher value to reduce the CPU load from checking the directory.
// Only applies if latest_data_info_avail is FALSE.
// Minimum val: 1
// Type: int
//
wait_between_checks = 2;
///////////// file_quiescence /////////////////////////
//
// File quiescence when checking for files - secs.
// This allows you to make sure that a file coming from a remote machine
// is complete before reading it. Only applies if latest_data_info_avail
// is FALSE.
// Type: int
//
file_quiescence = 60;
///////////// search_ext //////////////////////////////
//
// File name extension.
// If set, only files with this extension will be processed.
// Type: string
//
search_ext = "";
///////////// gematronik_realtime_mode ////////////////
//
// Set to TRUE if we are watching for Gematronik XML volumes.
// Gematronik volumes (for a given time) are stored in multiple files,
// one for each field. Therefore, after the time on a volume changes and
// a new field file is detected, we need to wait a while to ensure that
// all of the files have had a chance to be writted to disk. You need to
// set gematronik_realtime_wait_secs to a value in excess of the time it
// takes for all of the files to be written.
// Type: boolean
//
gematronik_realtime_mode = FALSE;
///////////// gematronik_realtime_wait_secs ///////////
//
// Number of seconds to wait, so that all field files can be written to
// disk before we start to read.
// See 'gematronik_realtime_mode'.
// Type: int
//
gematronik_realtime_wait_secs = 5;
//======================================================================
//
// OPTIONAL FIXED ANGLE OR SWEEP NUMBER LIMITS.
//
// Fixed angles are elevation in PPI mode and azimuth in RHI mode.
//
//======================================================================
///////////// set_fixed_angle_limits //////////////////
//
// Option to set fixed angle limits.
// Only use sweeps within the specified fixed angle limits.
// Type: boolean
//
set_fixed_angle_limits = FALSE;
///////////// lower_fixed_angle_limit /////////////////
//
// Lower fixed angle limit - degrees.
// Type: double
//
lower_fixed_angle_limit = 0;
///////////// upper_fixed_angle_limit /////////////////
//
// Upper fixed angle limit - degrees.
// Type: double
//
upper_fixed_angle_limit = 90;
///////////// set_sweep_num_limits ////////////////////
//
// Option to set sweep number limits.
// If 'apply_strict_angle_limits' is set, only read sweeps within the
// specified limits. If strict checking is false and no data lies within
// the limits, return the closest applicable sweep.
// Type: boolean
//
set_sweep_num_limits = FALSE;
///////////// lower_sweep_num /////////////////////////
//
// Lower sweep number limit.
// Type: int
//
lower_sweep_num = 0;
///////////// upper_sweep_num /////////////////////////
//
// Upper sweep number limit.
// Type: int
//
upper_sweep_num = 0;
///////////// apply_strict_angle_limits ///////////////
//
// Option to apply strict checking for angle or sweep number limits on
// read.
// If true, an error will occur if the fixed angle limits or sweep num
// limits are outside the bounds of the data. If false, a read is
// guaranteed to return at least 1 sweep - if no sweep lies within the
// angle limits set, the nearest sweep will be returned.
// Type: boolean
//
apply_strict_angle_limits = TRUE;
///////////// read_set_radar_num //////////////////////
//
// Option to set the radar number.
// See read_radar_num.
// Type: boolean
//
read_set_radar_num = FALSE;
///////////// read_radar_num //////////////////////////
//
// Set the radar number for the data to be extracted.
// Most files have data from a single radar, so this does not apply. The
// NOAA HRD files, however, have data from both the lower fuselage (LF,
// radar_num = 1) and tail (TA, radar_num = 2) radars. For HRD files, by
// default the TA radar will be used, unless the radar num is set to 1
// for the LF radar.
// Type: int
//
read_radar_num = 0;
//======================================================================
//
// READ OPTIONS.
//
//======================================================================
///////////// aggregate_sweep_files_on_read ///////////
//
// Option to aggregate sweep files into a volume on read.
// If true, and the input data is in sweeps rather than volumes (e.g.
// DORADE), the sweep files from a volume will be aggregated into a
// volume.
// Type: boolean
//
aggregate_sweep_files_on_read = FALSE;
///////////// aggregate_all_files_on_read /////////////
//
// Option to aggregate all files in the file list on read.
// If true, all of the files specified with the '-f' arg will be
// aggregated into a single volume as they are read in. This only
// applies to FILELIST mode. Overrides 'aggregate_sweep_files_on_read'.
// Type: boolean
//
aggregate_all_files_on_read = FALSE;
///////////// ignore_idle_scan_mode_on_read ///////////
//
// Option to ignore data taken in IDLE mode.
// If true, on read will ignore files with an IDLE scan mode.
// Type: boolean
//
ignore_idle_scan_mode_on_read = TRUE;
///////////// remove_rays_with_all_data_missing ///////
//
// Option to remove rays for which all data is missing.
// If true, ray data will be checked. If all fields have missing data at
// all gates, the ray will be removed after reading.
// Type: boolean
//
remove_rays_with_all_data_missing = FALSE;
///////////// remove_rays_with_antenna_transitions ////
//
// Option to remove rays taken while the antenna was in transition.
// If true, rays with the transition flag set will not be used. The
// transiton flag is set when the antenna is in transtion between one
// sweep and the next.
// Type: boolean
//
remove_rays_with_antenna_transitions = FALSE;
///////////// transition_nrays_margin /////////////////
//
// Number of transition rays to include as a margin.
// Sometimes the transition flag is turned on too early in a transition,
// on not turned off quickly enough after a transition. If you set this
// to a number greater than 0, that number of rays will be included at
// each end of the transition, i.e. the transition will effectively be
// shorter at each end by this number of rays.
// Type: int
//
transition_nrays_margin = 0;
///////////// trim_surveillance_sweeps_to_360deg //////
//
// Option to trip surveillance sweeps so that they only cover 360
// degrees.
// Some sweeps will have rays which cover more than a 360-degree
// rotation. Often these include antenna transitions. If this is set to
// true, rays are trimmed off either end of the sweep to limit the
// coverage to 360 degrees. The median elevation angle is computed and
// the end ray which deviates from the median in elevation is trimmed
// first.
// Type: boolean
//
trim_surveillance_sweeps_to_360deg = FALSE;
///////////// set_max_range ///////////////////////////
//
// Option to set the max range for any ray.
// Type: boolean
//
set_max_range = TRUE;
///////////// max_range_km ////////////////////////////
//
// Specified maximim range - km.
// Gates beyond this range are removed.
// Type: double
//
max_range_km = 230.0;
///////////// preserve_sweeps /////////////////////////
//
// Preserve sweeps just as they are in the file.
// Applies generally to NEXRAD data. If true, the sweep details are
// preserved. If false, we consolidate sweeps from split cuts into a
// single sweep.
// Type: boolean
//
preserve_sweeps = FALSE;
///////////// remove_long_range_rays //////////////////
//
// Option to remove long range rays.
// Applies to NEXRAD data. If true, data from the non-Doppler long-range
// sweeps will be removed.
// Type: boolean
//
remove_long_range_rays = TRUE;
///////////// remove_short_range_rays /////////////////
//
// Option to remove short range rays.
// Applies to NEXRAD data. If true, data from the Doppler short-range
// sweeps will be removed.
// Type: boolean
//
remove_short_range_rays = FALSE;
///////////// set_ngates_constant /////////////////////
//
// Option to force the number of gates to be constant.
// If TRUE, the number of gates on all rays will be set to the maximum,
// and gates added to shorter rays will be filled with missing values.
// Type: boolean
//
set_ngates_constant = FALSE;
//======================================================================
//
// OPTION TO OVERRIDE INSTRUMENT AND/OR NAME.
//
//======================================================================
///////////// override_instrument_name ////////////////
//
// Option to override the instrument name.
// If true, the name provided will be used.
// Type: boolean
//
override_instrument_name = FALSE;
///////////// instrument_name /////////////////////////
//
// Instrument name.
// See override_instrument_name.
// Type: string
//
instrument_name = "unknown";
///////////// override_site_name //////////////////////
//
// Option to override the site name.
// If true, the name provided will be used.
// Type: boolean
//
override_site_name = FALSE;
///////////// site_name ///////////////////////////////
//
// Site name.
// See override_site_name.
// Type: string
//
site_name = "unknown";
//======================================================================
//
// OPTION TO OVERRIDE RADAR LOCATION.
//
//======================================================================
///////////// override_radar_location /////////////////
//
// Option to override the radar location.
// If true, the location in this file will be used. If not, the location
// in the time series data will be used.
// Type: boolean
//
override_radar_location = FALSE;
///////////// radar_latitude_deg //////////////////////
//
// Radar latitude (deg).
// See override_radar_location.
// Type: double
//
radar_latitude_deg = -999;
///////////// radar_longitude_deg /////////////////////
//
// Radar longitude (deg).
// See override_radar_location.
// Type: double
//
radar_longitude_deg = -999;
///////////// radar_altitude_meters ///////////////////
//
// Radar altitude (meters).
// See override_radar_location.
// Type: double
//
radar_altitude_meters = -999;
///////////// change_radar_latitude_sign //////////////
//
// Option to negate the latitude.
// Mainly useful for RAPIC files. In RAPIC, latitude is always positive,
// so mostly you need to set the latitiude to the negative value of
// itself.
// Type: boolean
//
change_radar_latitude_sign = FALSE;
///////////// apply_georeference_corrections //////////
//
// Option to apply the georeference info for moving platforms.
// For moving platforms, measured georeference information is sometimes
// available. If this is set to true, the georeference data is applied
// and appropriate corrections made. If possible, Earth-centric azimuth
// and elevation angles will be computed.
// Type: boolean
//
apply_georeference_corrections = FALSE;
//======================================================================
//
// OPTION TO OVERRIDE SELECTED GLOBAL ATTRIBUTES.
//
//======================================================================
///////////// version_override ////////////////////////
//
// Option to override the version global attribute.
// If empty, no effect. If not empty, this string is used to override
// the version attribute.
// Type: string
//
version_override = "";
///////////// title_override //////////////////////////
//
// Option to override the title global attribute.
// If empty, no effect. If not empty, this string is used to override
// the title attribute.
// Type: string
//
title_override = "";
///////////// institution_override ////////////////////
//
// Option to override the institution global attribute.
// If empty, no effect. If not empty, this string is used to override
// the institution attribute.
// Type: string
//
institution_override = "";
///////////// references_override /////////////////////
//
// Option to override the references global attribute.
// If empty, no effect. If not empty, this string is used to override
// the references attribute.
// Type: string
//
references_override = "";
///////////// source_override /////////////////////////
//
// Option to override the source global attribute.
// If empty, no effect. If not empty, this string is used to override
// the source attribute.
// Type: string
//
source_override = "";
///////////// history_override ////////////////////////
//
// Option to override the history global attribute.
// If empty, no effect. If not empty, this string is used to override
// the history attribute.
// Type: string
//
history_override = "";
///////////// comment_override ////////////////////////
//
// Option to override the comment global attribute.
// If empty, no effect. If not empty, this string is used to override
// the comment attribute.
// Type: string
//
comment_override = "";
///////////// author_override /////////////////////////
//
// Option to override the author global attribute.
// If empty, no effect. If not empty, this string is used to override
// the author attribute.
// Type: string
//
author_override = "";
//======================================================================
//
// OPTION TO CORRECT ANTENNA ANGLES.
//
//======================================================================
///////////// apply_azimuth_offset ////////////////////
//
// Option to apply an offset to the azimuth values.
// If TRUE, this offset will be ADDED to the measured azimuth angles.
// This is useful, for example, in the case of a mobile platform which
// is not set up oriented to true north. Suppose you have a truck (like
// the DOWs) which is oriented off true north. Then if you add in the
// truck HEADING relative to true north, the measured azimuth angles
// will be adjusted by the heading, to give azimuth relative to TRUE
// north.
// Type: boolean
//
apply_azimuth_offset = FALSE;
///////////// azimuth_offset //////////////////////////
//
// Azimuth offset (degrees).
// See 'apply_azimuth_offset'. This value will be ADDED to the measured
// azimuths.
// Type: double
//
azimuth_offset = 0;
///////////// apply_elevation_offset //////////////////
//
// Option to apply an offset to the elevation values.
// If TRUE, this offset will be ADDED to the measured elevation angles.
// This is useful to correct for a systematic bias in measured elevation
// angles.
// Type: boolean
//
apply_elevation_offset = FALSE;
///////////// elevation_offset ////////////////////////
//
// Elevation offset (degrees).
// See 'apply_elevation_offset'. This value will be ADDED to the
// measured elevations.
// Type: double
//
elevation_offset = 0;
//======================================================================
//
// OPTION TO SPECIFY FIELD NAMES AND OUTPUT ENCODING.
//
//======================================================================
///////////// set_output_fields ///////////////////////
//
// Set the field names and output encoding.
// If false, all fields will be used.
// Type: boolean
//
set_output_fields = TRUE;
///////////// output_fields ///////////////////////////
//
// Output field details.
// Set the details for the output fields. The output_field_name is the
// ndtCDF variable name. Set the long name to a more descriptive name.
// Set the standard name to the CF standard name for this field. If the
// long name or standard name are empty, the existing names are used. If
// SCALING_SPECIFIED, then the scale and offset is used.
//
// Type: struct
// typedef struct {
// string input_field_name;
// string output_field_name;
// string long_name;
// string standard_name;
// string output_units;
// output_encoding_t encoding;
// Options:
// OUTPUT_ENCODING_ASIS
// OUTPUT_ENCODING_FLOAT32
// OUTPUT_ENCODING_INT32
// OUTPUT_ENCODING_INT16
// OUTPUT_ENCODING_INT08
// output_scaling_t output_scaling;
// Options:
// SCALING_DYNAMIC
// SCALING_SPECIFIED
// double output_scale;
// double output_offset;
// }
//
// 1D array - variable length.
//
output_fields = {
{
input_field_name = "REF",
output_field_name = "DBZ",
long_name = "radar_reflectivity",
standard_name = "equivalent_reflectivity_factor",
output_units = "dBZ",
encoding = OUTPUT_ENCODING_INT16,
output_scaling = SCALING_DYNAMIC,
output_scale = 0.01,
output_offset = 0
}
,
{
input_field_name = "VEL",
output_field_name = "VEL",
long_name = "radial_velocity",
standard_name = "radial_velocity_of_scatterers_away_from_instrument",
output_units = "m/s",
encoding = OUTPUT_ENCODING_INT16,
output_scaling = SCALING_DYNAMIC,
output_scale = 0.01,
output_offset = 0
}
,
{
input_field_name = "SW",
output_field_name = "WIDTH",
long_name = "spectrum_width",
standard_name = "doppler_spectrum_width",
output_units = "m/s",
encoding = OUTPUT_ENCODING_INT16,
output_scaling = SCALING_DYNAMIC,
output_scale = 0.01,
output_offset = 0
}
,
{
input_field_name = "ZDR",
output_field_name = "ZDR",
long_name = "differential_reflectivity",
standard_name = "log_differential_reflectivity_hv",
output_units = "dB",
encoding = OUTPUT_ENCODING_INT16,
output_scaling = SCALING_DYNAMIC,
output_scale = 0.01,
output_offset = 0
}
,
{
input_field_name = "PHI",
output_field_name = "PHIDP",
long_name = "differential_phase",
standard_name = "differential_phase_hv",
output_units = "deg",
encoding = OUTPUT_ENCODING_INT16,
output_scaling = SCALING_DYNAMIC,
output_scale = 0.01,
output_offset = 0
}
,
{
input_field_name = "RHO",
output_field_name = "RHOHV",
long_name = "cross_correlation",
standard_name = "cross_correlation_ratio_hv",
output_units = "",
encoding = OUTPUT_ENCODING_INT16,
output_scaling = SCALING_DYNAMIC,
output_scale = 0.01,
output_offset = 0
}
};
///////////// write_other_fields_unchanged ////////////
//
// Option to write out the unspecified fields as they are.
// If false, only the fields listed in output_fields will be written. If
// this is true, all other fields will be written unchanged.
// Type: boolean
//
write_other_fields_unchanged = FALSE;
///////////// exclude_specified_fields ////////////////
//
// Option to exclude fields in the specified list.
// If true, the specified fields will be excluded. This may be easier
// than specifiying all of the fields to be included, if that list is
// very long.
// Type: boolean
//
exclude_specified_fields = FALSE;
///////////// excluded_fields /////////////////////////
//
// List of fields to be excluded.
// List the names to be excluded.
// Type: string
// 1D array - variable length.
//
excluded_fields = {
"DBZ",
"VEL"
};
//======================================================================
//
// OPTION TO SPECIFY OUTPUT ENCODING FOR ALL FIELDS.
//
//======================================================================
///////////// set_output_encoding_for_all_fields //////
//
// Option to set output encoding for all fields.
// Type: boolean
//
set_output_encoding_for_all_fields = FALSE;
///////////// output_encoding /////////////////////////
//
// Output encoding for all fields, if requested.
//
// Type: enum
// Options:
// OUTPUT_ENCODING_ASIS
// OUTPUT_ENCODING_FLOAT32
// OUTPUT_ENCODING_INT32
// OUTPUT_ENCODING_INT16
// OUTPUT_ENCODING_INT08
//
output_encoding = OUTPUT_ENCODING_ASIS;
//======================================================================
//
// CENSORING.
//
// You have the option of censoring the data fields - i.e. setting the
// fields to missing values - at gates which meet certain criteria. If
// this is done correctly, it allows you to preserve the valid data and
// discard the noise, thereby improving compression.
//
//======================================================================
///////////// apply_censoring /////////////////////////
//
// Apply censoring based on field values and thresholds.
// If TRUE, censoring will be performed. See 'censoring_fields' for
// details on how the censoring is applied.
// Type: boolean
//
apply_censoring = FALSE;
///////////// censoring_fields ////////////////////////
//
// Fields to be used for censoring.
// Specify the fields to be used to determine whether a gate should be
// censored. The name refers to the input data field names. Valid field
// values lie in the range from min_valid_value to max_valid_value
// inclusive. If the value of a field at a gate lies within this range,
// it is considered valid. Each specified field is examined at each
// gate, and is flagged as valid if its value lies in the valid range.
// These field flags are then combined as follows: first, all of the
// LOGICAL_OR flags are combined, yielding a single combined_or flag
// which is true if any of the LOGICAL_OR fields is true. The
// combined_or flag is then combined with all of the LOGICAL_AND fields,
// yielding a true value only if the combined_or flag and the
// LOGICAL_AND fields are all true. If this final flag is true, then the
// data at the gate is regarded as valid and is retained. If the final
// flag is false, the data at the gate is censored, and all of the
// fields at the gate are set to missing.
//
// Type: struct
// typedef struct {
// string name;
// double min_valid_value;
// double max_valid_value;
// logical_t combination_method;
// Options:
// LOGICAL_AND
// LOGICAL_OR
// }
//
// 1D array - variable length.
//
censoring_fields = {
{
name = "SNR",
min_valid_value = 0,
max_valid_value = 1000,
combination_method = LOGICAL_OR
}
,
{
name = "NCP",
min_valid_value = 0.15,
max_valid_value = 1000,
combination_method = LOGICAL_OR
}
};
///////////// censoring_min_valid_run /////////////////
//
// Minimum valid run of non-censored gates.
// Only active if set to 2 or greater. A check is made to remove short
// runs of noise. Looking along the radial, we compute the number of
// contiguous gates (a 'run') with uncensored data. For the gates in
// this run to be accepted the length of the run must exceed
// censoring_min_valid_run. If the number of gates in a run is less than
// this, then all gates in the run are censored.
// Type: int
//
censoring_min_valid_run = 1;
//======================================================================
//
// OPTION TO APPLY LINEAR TRANSFORM TO SPECIFIED FIELDS.
//
// These transforms are fixed. The same transform is applied to all
// files.
//
//======================================================================
///////////// apply_linear_transforms /////////////////
//
// Apply linear transform to specified fields.
// If true, we will apply a linear transform to selected fields.
// Type: boolean
//
apply_linear_transforms = FALSE;
///////////// transform_fields ////////////////////////
//
// transform field details.
// Set the field name, scale and offset to be applied to the selected
// fields. NOTE: the field name is the INPUT field name.
//
// Type: struct
// typedef struct {
// string input_field_name;
// double transform_scale;
// double transform_offset;
// }
//
// 1D array - variable length.
//
transform_fields = {
{
input_field_name = "DBZ",
transform_scale = 1,
transform_offset = 0
}
,
{
input_field_name = "VEL",
transform_scale = 1,
transform_offset = 0
}
};
//======================================================================
//
// OPTION TO APPLY VARIABLE LINEAR TRANSFORM TO SPECIFIED FIELDS.
//
// These transforms vary from file to file, controlled by specific
// metadata.
//
//======================================================================
///////////// apply_variable_transforms ///////////////
//
// Apply linear transforms that vary based on specific metadata.
// If true, we will apply variable linear transform to selected fields.
// Type: boolean
//
apply_variable_transforms = FALSE;
///////////// variable_transform_fields ///////////////
//
// Details for variable transforms.
// We based the field decision off the input_field_name. You need to
// pick the method of control: STATUS_XML_FIELD - based on the value
// associated with an XML tag in the status block; ELEVATION_DEG - based
// on the elevation in degrees; PULSE_WIDTH_US - based on the pulse with
// in microsecs. For STATUS_XML_FIELD Set the relevant status_xml_tag,
// which will be used to find the relevant value. The lookup table is a
// series of entries specifying the metadata_value and the scale and
// offset to be appied for that given metadata value. Each entry is
// enclosed in parentheses, and is of the form (metadata_value, scale,
// offset). The entries themselves are also are comma-separated.
// Interpolation is used for metadata values that lie between those
// specified in the lookup table. The enries in the lookup table should
// have metadata_values that are monotonically increasing.
//
// Type: struct
// typedef struct {
// string input_field_name;
// variable_transform_control_t control;
// Options:
// STATUS_XML_FIELD
// string xml_tag;
// string lookup_table;
// }
//
// 1D array - variable length.
//
variable_transform_fields = {
{
input_field_name = "dBZ",
control = STATUS_XML_FIELD,
xml_tag = "gdrxanctxfreq",
lookup_table = "(57.0, 1.0, -0.7), (60.0, 1.0, -0.2), (64.0, 1.0, -0.3), (67.0, 1.0, -1.8), (68.0, 1.0, -1.2), (69.0, 1.0, -1.3)"
}
,
{
input_field_name = "dBZv",
control = STATUS_XML_FIELD,
xml_tag = "gdrxanctxfreq",
lookup_table = "(57.0, 1.0, 0.1), (58.0, 1.0, 0.3), (60.0, 1.0, -0.3), (67.0, 1.0, -2.3), (69.0, 1.0, -2.0)"
}
,
{
input_field_name = "ZDR",
control = STATUS_XML_FIELD,
xml_tag = "gdrxanctxfreq",
lookup_table = "(56.0, 1.0, -0.75), (58.0, 1.0, -0.75), (61.0, 1.0, 0.1), (63.5, 1.0, 0.2), (64.0, 1.0, 0.6), (69.0, 1.0, 0.6)"
}
};
//======================================================================
//
// OUTPUT FORMAT.
//
//======================================================================
///////////// output_format ///////////////////////////
//
// Format for the output files.
//
// Type: enum
// Options:
// OUTPUT_FORMAT_CFRADIAL
// OUTPUT_FORMAT_DORADE
// OUTPUT_FORMAT_FORAY
// OUTPUT_FORMAT_NEXRAD
// OUTPUT_FORMAT_UF
// OUTPUT_FORMAT_MDV_RADIAL
//
output_format = OUTPUT_FORMAT_CFRADIAL;
///////////// netcdf_style ////////////////////////////
//
// NetCDF style - if output_format is CFRADIAL.
// netCDF classic format, netCDF 64-bit offset format, netCDF4 using
// HDF5 format, netCDF4 using HDF5 format but only netCDF3 calls.
//
// Type: enum
// Options:
// CLASSIC
// NC64BIT
// NETCDF4
// NETCDF4_CLASSIC
//
netcdf_style = NETCDF4;
//======================================================================
//
// OUTPUT BYTE-SWAPPING and COMPRESSION.
//
//======================================================================
///////////// output_native_byte_order ////////////////
//
// Option to leave data in native byte order.
// If false, data will be byte-swapped as appropriate on output.
// Type: boolean
//
output_native_byte_order = FALSE;
///////////// output_compressed ///////////////////////
//
// Option to compress data fields on output.
// Applies to netCDF and Dorade. UF does not support compression.
// Type: boolean
//
output_compressed = TRUE;
//======================================================================
//
// OUTPUT OPTIONS FOR CfRadial FILES.
//
//======================================================================
///////////// output_force_ngates_vary ////////////////
//
// Option to force the use of ragged arrays for CfRadial files.
// Only applies to CfRadial. If true, forces the use of ragged arrays
// even if the number of gates for all rays is constant.
// Type: boolean
//
output_force_ngates_vary = FALSE;
///////////// compression_level ///////////////////////
//
// Compression level for output, if compressed.
// Applies to netCDF only. Dorade compression is run-length encoding,
// and has not options..
// Type: int
//
compression_level = 4;
//======================================================================
//
// OUTPUT DIRECTORY AND FILE NAME.
//
//======================================================================
///////////// output_dir //////////////////////////////
//
// Output directory path.
// Files will be written to this directory.
// Type: string
//
output_dir = "$(NEXRAD_DATA_DIR)/cfradial/moments/$(RADAR_NAME)";
///////////// output_filename_mode ////////////////////
//
// Mode for computing output file name.
// START_AND_END_TIMES: include both start and end times in file name.
// START_TIME_ONLY: include only start time in file name. END_TIME_ONLY:
// include only end time in file name. SPECIFY_FILE_NAME: file of this
// name will be written to output_dir.
//
// Type: enum
// Options:
// START_AND_END_TIMES
// START_TIME_ONLY
// END_TIME_ONLY
// SPECIFY_FILE_NAME
//
output_filename_mode = START_AND_END_TIMES;
///////////// output_filename_prefix //////////////////
//
// Optional prefix for output filename.
// If empty, the standard prefix will be used.
// Type: string
//
output_filename_prefix = "";
///////////// include_instrument_name_in_file_name ////
//
// Option to include the instrument name in the file name.
// Default is true. Only applies to CfRadial files. If true, the
// instrument name will be included just before the volume number in the
// output file name.
// Type: boolean
//
include_instrument_name_in_file_name = TRUE;
///////////// include_subsecs_in_file_name ////////////
//
// Option to include sub-seconds in date-time part of file name.
// Default is true. Only applies to CfRadial files. If true, the
// millisecs of the start and end time will be included in the file
// name.
// Type: boolean
//
include_subsecs_in_file_name = TRUE;
///////////// use_hyphen_in_file_name_datetime_part ///
//
// Option to use a hyphen between date and time in filename.
// Default is false. Only applies to CfRadial files. Normally an
// underscore is used.
// Type: boolean
//
use_hyphen_in_file_name_datetime_part = FALSE;
///////////// output_filename /////////////////////////
//
// Name of output file.
// Applies only if output_filename_mode is SPECIFY_FILE_NAME. File of
// this name will be written to output_dir.
// Type: string
//
output_filename = "cfradial.test.nc";
///////////// append_day_dir_to_output_dir ////////////
//
// Add the day directory to the output directory.
// Path will be output_dir/yyyymmdd/filename.
// Type: boolean
//
append_day_dir_to_output_dir = TRUE;
///////////// append_year_dir_to_output_dir ///////////
//
// Add the year directory to the output directory.
// Path will be output_dir/yyyy/yyyymmdd/filename.
// Type: boolean
//
append_year_dir_to_output_dir = FALSE;
///////////// write_individual_sweeps /////////////////
//
// Option to write out individual sweeps if appropriate.
// If true, the volume is split into individual sweeps for writing.
// Applies to CfRadial format. This is always true for DORADE format
// files.
// Type: boolean
//
write_individual_sweeps = FALSE;
///////////// write_latest_data_info //////////////////
//
// Option to write out _latest_data_info files.
// If true, the _latest_data_info files will be written after the
// converted file is written.
// Type: boolean
//
write_latest_data_info = TRUE;
//======================================================================
//
// OPTION TO OVERRIDE MISSING VALUES.
//
// Missing values are applicable to both metadata and field data. The
// default values should be satisfactory for most purposes. However, you
// can choose to override these if you are careful with the selected
// values.
// The default values for metadata are:
// missingMetaDouble = -9999.0
// missingMetaFloat = -9999.0
// missingMetaInt = -9999
// missingMetaChar = -128
// The default values for field data are:
// missingFl64 = -9.0e33
// missingFl32 = -9.0e33
// missingSi32 = -2147483647
// missingSi16 = -32768
// missingSi08 = -128.
//
//======================================================================
///////////// override_missing_metadata_values ////////
//
// Option to override the missing values for meta-data.
// See following parameter options.
// Type: boolean
//
override_missing_metadata_values = FALSE;
///////////// missing_metadata_double /////////////////
//
// Missing value for metadata of type double.
// Only applies if override_missing_metadata_values is TRUE.
// Type: double
//
missing_metadata_double = -9999;
///////////// missing_metadata_float //////////////////
//
// Missing value for metadata of type float.
// Only applies if override_missing_metadata_values is TRUE.
// Type: float
//
missing_metadata_float = -9999;
///////////// missing_metadata_int ////////////////////
//
// Missing value for metadata of type int.
// Only applies if override_missing_metadata_values is TRUE.
// Type: int
//
missing_metadata_int = -9999;
///////////// missing_metadata_char ///////////////////
//
// Missing value for metadata of type char.
// Only applies if override_missing_metadata_values is TRUE.
// Type: int
//
missing_metadata_char = -128;
///////////// override_missing_field_values ///////////
//
// Option to override the missing values for field data.
// See following parameter options.
// Type: boolean
//
override_missing_field_values = FALSE;
///////////// missing_field_fl64 //////////////////////
//
// Missing value for field data of type 64-bit float.
// Only applies if override_missing_field_values is TRUE.
// Type: double
//
missing_field_fl64 = -9999;
///////////// missing_field_fl32 //////////////////////
//
// Missing value for field data of type 32-bit float.
// Only applies if override_missing_field_values is TRUE.
// Type: double
//
missing_field_fl32 = -9999;
///////////// missing_field_si32 //////////////////////
//
// Missing value for field data of type 32-bit integer.
// Only applies if override_missing_field_values is TRUE.
// Type: int
//
missing_field_si32 = -999999;
///////////// missing_field_si16 //////////////////////
//
// Missing value for field data of type 16-bit integer.
// Only applies if override_missing_field_values is TRUE.
// Type: int
//
missing_field_si16 = -232768;
///////////// missing_field_si08 //////////////////////
//
// Missing value for field data of type 8-bit integer.
// Only applies if override_missing_field_values is TRUE.
// Type: int
//
missing_field_si08 = -128;
Convert raw nexrad files to CfRadial format
# Convert raw nexrad data to cfradial for 3 NEXRAD radars
for radar_name in ['KGLD', 'KUEX', 'KDDC']:
# Set radar in name environment variable
os.environ['RADAR_NAME'] = radar_name
# Run RadxConvert using param file
!/usr/local/lrose/bin/RadxConvert -params ./params/RadxConvert.nexrad -debug -start "2021 07 06 22 00 00" -end "2021 07 06 22 30 00"
======================================================================
Program 'RadxConvert'
Run-time 2024/02/26 08:56:13.
Copyright (c) 1992 - 2024
University Corporation for Atmospheric Research (UCAR)
National Center for Atmospheric Research (NCAR)
Boulder, Colorado, USA.
Redistribution and use in source and binary forms, with
or without modification, are permitted provided that the following
conditions are met:
1) Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
2) Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
3) Neither the name of UCAR, NCAR nor the names of its contributors, if
any, may be used to endorse or promote products derived from this
software without specific prior written permission.
4) If the software is modified to produce derivative works, such modified
software should be clearly marked, so as not to confuse it with the
version available from UCAR.
======================================================================
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_220003_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_220003_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937777.250579.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937777.250579.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_220448_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_220448_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220448.793_to_20210706_220926.383_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937781.774386.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937781.774386.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220448.793_to_20210706_220926.383_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220448.793_to_20210706_220926.383_KGLD_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_220935_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_220935_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220935.631_to_20210706_221411.627_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937786.295387.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937786.295387.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220935.631_to_20210706_221411.627_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220935.631_to_20210706_221411.627_KGLD_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_221420_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_221420_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_221420.555_to_20210706_221857.324_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937790.795951.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937790.795951.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_221420.555_to_20210706_221857.324_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_221420.555_to_20210706_221857.324_KGLD_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_221906_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_221906_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_221906.199_to_20210706_222341.850_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937795.296733.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937795.296733.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_221906.199_to_20210706_222341.850_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_221906.199_to_20210706_222341.850_KGLD_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_222350_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_222350_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_222350.154_to_20210706_222826.584_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937799.795447.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937799.795447.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_222350.154_to_20210706_222826.584_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_222350.154_to_20210706_222826.584_KGLD_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_222834_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KGLD/20210706/KGLD20210706_222834_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_222834.963_to_20210706_223310.845_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937804.287574.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/tmp.747.1708937804.287574.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_222834.963_to_20210706_223310.845_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_222834.963_to_20210706_223310.845_KGLD_SUR.nc
======================================================================
Program 'RadxConvert'
Run-time 2024/02/26 08:56:45.
Copyright (c) 1992 - 2024
University Corporation for Atmospheric Research (UCAR)
National Center for Atmospheric Research (NCAR)
Boulder, Colorado, USA.
Redistribution and use in source and binary forms, with
or without modification, are permitted provided that the following
conditions are met:
1) Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
2) Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
3) Neither the name of UCAR, NCAR nor the names of its contributors, if
any, may be used to endorse or promote products derived from this
software without specific prior written permission.
4) If the software is modified to produce derivative works, such modified
software should be clearly marked, so as not to confuse it with the
version available from UCAR.
======================================================================
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_220249_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_220249_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_220249.032_to_20210706_220715.866_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937809.246465.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937809.246465.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_220249.032_to_20210706_220715.866_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_220249.032_to_20210706_220715.866_KUEX_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_220723_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_220723_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_220723.969_to_20210706_221157.362_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937813.704149.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937813.704149.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_220723.969_to_20210706_221157.362_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_220723.969_to_20210706_221157.362_KUEX_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_221204_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_221204_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_221204.520_to_20210706_221625.502_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937818.153386.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937818.153386.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_221204.520_to_20210706_221625.502_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_221204.520_to_20210706_221625.502_KUEX_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_221633_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_221633_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_221633.850_to_20210706_222054.868_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937822.605691.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937822.605691.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_221633.850_to_20210706_222054.868_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_221633.850_to_20210706_222054.868_KUEX_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_222102_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_222102_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_222102.216_to_20210706_222523.504_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937827.55554.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937827.55554.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_222102.216_to_20210706_222523.504_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_222102.216_to_20210706_222523.504_KUEX_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_222531_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KUEX/20210706/KUEX20210706_222531_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_222531.244_to_20210706_222952.818_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937831.458592.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/tmp.748.1708937831.458592.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_222531.244_to_20210706_222952.818_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_222531.244_to_20210706_222952.818_KUEX_SUR.nc
======================================================================
Program 'RadxConvert'
Run-time 2024/02/26 08:57:12.
Copyright (c) 1992 - 2024
University Corporation for Atmospheric Research (UCAR)
National Center for Atmospheric Research (NCAR)
Boulder, Colorado, USA.
Redistribution and use in source and binary forms, with
or without modification, are permitted provided that the following
conditions are met:
1) Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
2) Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
3) Neither the name of UCAR, NCAR nor the names of its contributors, if
any, may be used to endorse or promote products derived from this
software without specific prior written permission.
4) If the software is modified to produce derivative works, such modified
software should be clearly marked, so as not to confuse it with the
version available from UCAR.
======================================================================
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_220000_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_220000_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220000.765_to_20210706_220422.888_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937836.730667.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937836.730667.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220000.765_to_20210706_220422.888_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220000.765_to_20210706_220422.888_KDDC_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_220430_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_220430_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220430.757_to_20210706_220912.758_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937841.694859.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937841.694859.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220430.757_to_20210706_220912.758_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220430.757_to_20210706_220912.758_KDDC_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_220921_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_220921_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220921.610_to_20210706_221350.957_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937846.575596.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937846.575596.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220921.610_to_20210706_221350.957_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220921.610_to_20210706_221350.957_KDDC_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_221600_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_221600_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_221600.999_to_20210706_222043.450_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937851.534200.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937851.534200.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_221600.999_to_20210706_222043.450_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_221600.999_to_20210706_222043.450_KDDC_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_222051_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_222051_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_222051.218_to_20210706_222526.565_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937856.498616.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937856.498616.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_222051.218_to_20210706_222526.565_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_222051.218_to_20210706_222526.565_KDDC_SUR.nc
INFO - RadxConvert::Run
Input path: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_222533_V06
WARNING - NexradRadxFile::readFromPath
Adaptation data probably not set, ignoring
File: /tmp/lrose_data/nexrad_mosaic/raw/KDDC/20210706/KDDC20210706_222533_V06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_222533.934_to_20210706_223025.212_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937861.472506.tmp
Writing fields and compressing ...
... writing field: DBZ
... writing field: VEL
... writing field: WIDTH
... writing field: ZDR
... writing field: PHIDP
... writing field: RHOHV
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/tmp.749.1708937861.472506.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_222533.934_to_20210706_223025.212_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_222533.934_to_20210706_223025.212_KDDC_SUR.nc
List the CfRadial files created by RadxConvert
# List the CfRadial files created by RadxConvert
!ls -R ${NEXRAD_DATA_DIR}/cfradial/moments/K*/20*
/tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706:
cfrad.20210706_220000.765_to_20210706_220422.888_KDDC_SUR.nc
cfrad.20210706_220430.757_to_20210706_220912.758_KDDC_SUR.nc
cfrad.20210706_220921.610_to_20210706_221350.957_KDDC_SUR.nc
cfrad.20210706_221600.999_to_20210706_222043.450_KDDC_SUR.nc
cfrad.20210706_222051.218_to_20210706_222526.565_KDDC_SUR.nc
cfrad.20210706_222533.934_to_20210706_223025.212_KDDC_SUR.nc
/tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706:
cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc
cfrad.20210706_220448.793_to_20210706_220926.383_KGLD_SUR.nc
cfrad.20210706_220935.631_to_20210706_221411.627_KGLD_SUR.nc
cfrad.20210706_221420.555_to_20210706_221857.324_KGLD_SUR.nc
cfrad.20210706_221906.199_to_20210706_222341.850_KGLD_SUR.nc
cfrad.20210706_222350.154_to_20210706_222826.584_KGLD_SUR.nc
cfrad.20210706_222834.963_to_20210706_223310.845_KGLD_SUR.nc
/tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706:
cfrad.20210706_220249.032_to_20210706_220715.866_KUEX_SUR.nc
cfrad.20210706_220723.969_to_20210706_221157.362_KUEX_SUR.nc
cfrad.20210706_221204.520_to_20210706_221625.502_KUEX_SUR.nc
cfrad.20210706_221633.850_to_20210706_222054.868_KUEX_SUR.nc
cfrad.20210706_222102.216_to_20210706_222523.504_KUEX_SUR.nc
cfrad.20210706_222531.244_to_20210706_222952.818_KUEX_SUR.nc
Plot one example of the NEXRAD Goodland radar (KGLD) CfRadial files
# Read CfRadial file into radar object
filePathRadar = os.path.join(nexradDataDir, "cfradial/moments/KGLD/20210706/cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc")
radar_kgld = pyart.io.read_cfradial(filePathRadar)
radar_kgld.info('compact')
altitude: <ndarray of type: float64 and shape: (1,)>
altitude_agl: <ndarray of type: float64 and shape: (1,)>
antenna_transition: <ndarray of type: int8 and shape: (6120,)>
azimuth: <ndarray of type: float32 and shape: (6120,)>
elevation: <ndarray of type: float32 and shape: (6120,)>
fields:
DBZ: <ndarray of type: float32 and shape: (6120, 912)>
VEL: <ndarray of type: float32 and shape: (6120, 912)>
WIDTH: <ndarray of type: float32 and shape: (6120, 912)>
ZDR: <ndarray of type: float32 and shape: (6120, 912)>
PHIDP: <ndarray of type: float32 and shape: (6120, 912)>
RHOHV: <ndarray of type: float32 and shape: (6120, 912)>
fixed_angle: <ndarray of type: float32 and shape: (14,)>
instrument_parameters:
follow_mode: <ndarray of type: |S1 and shape: (14, 32)>
pulse_width: <ndarray of type: float32 and shape: (6120,)>
prt_mode: <ndarray of type: |S1 and shape: (14, 32)>
prt: <ndarray of type: float32 and shape: (6120,)>
prt_ratio: <ndarray of type: float32 and shape: (6120,)>
polarization_mode: <ndarray of type: |S1 and shape: (14, 32)>
nyquist_velocity: <ndarray of type: float32 and shape: (6120,)>
unambiguous_range: <ndarray of type: float32 and shape: (6120,)>
n_samples: <ndarray of type: int32 and shape: (6120,)>
radar_antenna_gain_h: <ndarray of type: float32 and shape: (1,)>
radar_antenna_gain_v: <ndarray of type: float32 and shape: (1,)>
radar_beam_width_h: <ndarray of type: float32 and shape: (1,)>
radar_beam_width_v: <ndarray of type: float32 and shape: (1,)>
radar_rx_bandwidth: <ndarray of type: float32 and shape: (1,)>
measured_transmit_power_v: <ndarray of type: float32 and shape: (6120,)>
measured_transmit_power_h: <ndarray of type: float32 and shape: (6120,)>
latitude: <ndarray of type: float64 and shape: (1,)>
longitude: <ndarray of type: float64 and shape: (1,)>
nsweeps: 14
ngates: 912
nrays: 6120
radar_calibration:
r_calib_time: <ndarray of type: |S1 and shape: (1, 32)>
r_calib_pulse_width: <ndarray of type: float32 and shape: (1,)>
r_calib_xmit_power_h: <ndarray of type: float32 and shape: (1,)>
r_calib_xmit_power_v: <ndarray of type: float32 and shape: (1,)>
r_calib_two_way_waveguide_loss_h: <ndarray of type: float32 and shape: (1,)>
r_calib_two_way_waveguide_loss_v: <ndarray of type: float32 and shape: (1,)>
r_calib_two_way_radome_loss_h: <ndarray of type: float32 and shape: (1,)>
r_calib_two_way_radome_loss_v: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_mismatch_loss: <ndarray of type: float32 and shape: (1,)>
r_calib_k_squared_water: <ndarray of type: float32 and shape: (1,)>
r_calib_radar_constant_h: <ndarray of type: float32 and shape: (1,)>
r_calib_radar_constant_v: <ndarray of type: float32 and shape: (1,)>
r_calib_antenna_gain_h: <ndarray of type: float32 and shape: (1,)>
r_calib_antenna_gain_v: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_i0_dbm_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_i0_dbm_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_i0_dbm_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_i0_dbm_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_gain_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_gain_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_gain_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_gain_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_slope_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_slope_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_slope_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_slope_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_dynamic_range_db_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_dynamic_range_db_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_dynamic_range_db_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_dynamic_range_db_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_base_dbz_1km_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_base_dbz_1km_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_base_dbz_1km_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_base_dbz_1km_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_sun_power_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_sun_power_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_sun_power_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_sun_power_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_source_power_h: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_source_power_v: <ndarray of type: float32 and shape: (1,)>
r_calib_power_measure_loss_h: <ndarray of type: float32 and shape: (1,)>
r_calib_power_measure_loss_v: <ndarray of type: float32 and shape: (1,)>
r_calib_coupler_forward_loss_h: <ndarray of type: float32 and shape: (1,)>
r_calib_coupler_forward_loss_v: <ndarray of type: float32 and shape: (1,)>
r_calib_dbz_correction: <ndarray of type: float32 and shape: (1,)>
r_calib_zdr_correction: <ndarray of type: float32 and shape: (1,)>
r_calib_ldr_correction_h: <ndarray of type: float32 and shape: (1,)>
r_calib_ldr_correction_v: <ndarray of type: float32 and shape: (1,)>
r_calib_system_phidp: <ndarray of type: float32 and shape: (1,)>
r_calib_test_power_h: <ndarray of type: float32 and shape: (1,)>
r_calib_test_power_v: <ndarray of type: float32 and shape: (1,)>
r_calib_index: <ndarray of type: int32 and shape: (6120,)>
range: <ndarray of type: float32 and shape: (912,)>
scan_rate: <ndarray of type: float32 and shape: (6120,)>
scan_type: other
sweep_end_ray_index: <ndarray of type: int32 and shape: (14,)>
sweep_mode: <ndarray of type: |S1 and shape: (14, 32)>
sweep_number: <ndarray of type: int32 and shape: (14,)>
sweep_start_ray_index: <ndarray of type: int32 and shape: (14,)>
target_scan_rate: <ndarray of type: float32 and shape: (14,)>
time: <ndarray of type: float64 and shape: (6120,)>
metadata:
Conventions: CF-1.7
Sub_conventions: CF-Radial instrument_parameters radar_parameters radar_calibration
version: CF-Radial-1.4
title:
institution:
references:
source: ARCHIVE 2 data
history:
comment:
original_format: NEXRAD
driver: RadxConvert(NCAR)
created: 2024/02/26 08:56:17.249
start_datetime: 2021-07-06T22:00:03Z
time_coverage_start: 2021-07-06T22:00:03Z
start_time: 2021-07-06 22:00:03.963
end_datetime: 2021-07-06T22:04:39Z
time_coverage_end: 2021-07-06T22:04:39Z
end_time: 2021-07-06 22:04:39.770
instrument_name: KGLD
site_name: DLGK
scan_name: Surveillance
scan_id: 212
platform_is_mobile: false
n_gates_vary: false
ray_times_increase: true
volume_number: 79
platform_type: fixed
instrument_type: radar
primary_axis: axis_z
# Plot KGLD fields from this CfRadial file
displayKgld = pyart.graph.RadarDisplay(radar_kgld)
figKgld = plt.figure(1, (12, 10))
# DBZ
axDbz = figKgld.add_subplot(221)
displayKgld.plot_ppi('DBZ', 0, vmin=-32, vmax=64.,
axislabels=("x(km)", "y(km)"),
colorbar_label="DBZ")
displayKgld.plot_range_rings([50, 100, 150, 200])
displayKgld.plot_cross_hair(200.)
# VEL
axVel = figKgld.add_subplot(222)
displayKgld.plot_ppi('VEL', 0, vmin=-30, vmax=30.,
axislabels=("x(km)", "y(km)"),
colorbar_label="VEL(m/s)",
cmap = "rainbow")
displayKgld.plot_range_rings([50, 100, 150, 200])
displayKgld.plot_cross_hair(200.)
# ZDR
axZdr = figKgld.add_subplot(223)
displayKgld.plot_ppi('ZDR', 0, vmin=-4, vmax=16.,
axislabels=("x(km)", "y(km)"),
colorbar_label="ZDR(dB)",
cmap = "rainbow")
displayKgld.plot_range_rings([50, 100, 150, 200])
displayKgld.plot_cross_hair(200.)
# PHIDP
axPhidp = figKgld.add_subplot(224)
displayKgld.plot_ppi('PHIDP', 0,
axislabels=("x(km)", "y(km)"),
colorbar_label="PHIDP(deg)",
cmap = "rainbow")
displayKgld.plot_range_rings([50, 100, 150, 200])
displayKgld.plot_cross_hair(200.)
# set layout and display
figKgld.tight_layout()
plt.show()
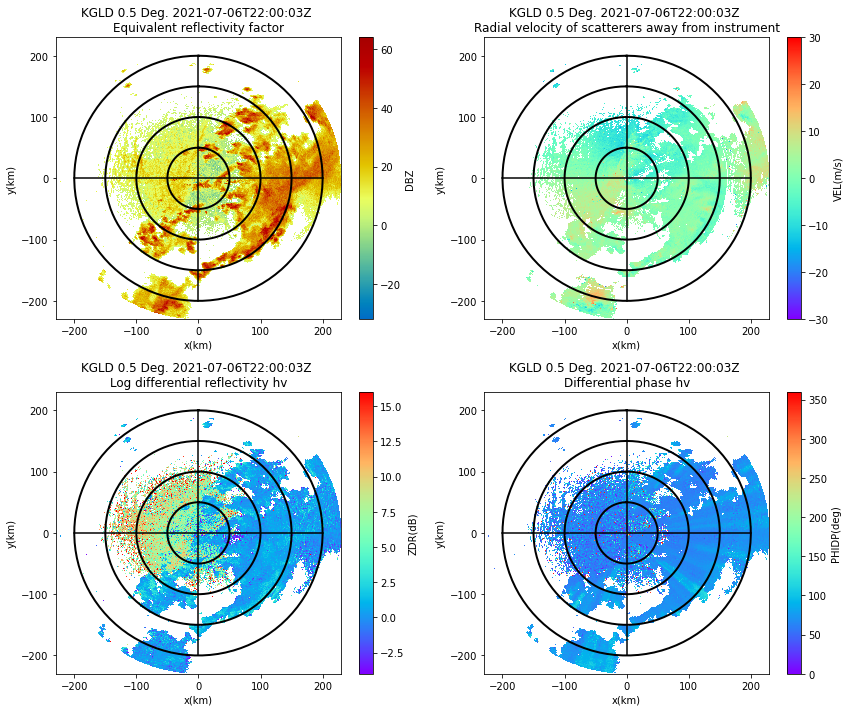
Read in temperature field from RUC model, to provide temperature profile for PID and Ecco
filePathModel = os.path.join(nexradDataDir, 'mdv/ruc/20210706/20210706_230000.mdv.cf.nc')
dsModel = nc.Dataset(filePathModel)
print(dsModel)
dstemp = dsModel['TMP']
temp3D = np.array(dstemp)
fillValueTemp = dstemp._FillValue
if (len(temp3D.shape) == 4):
temp3D = temp3D[0]
# Compute time
uTimeSecsModel = dsModel['start_time'][0]
startTimeModel = datetime.datetime.fromtimestamp(int(uTimeSecsModel))
startTimeStrModel = startTimeModel.strftime('%Y/%m/%d-%H:%M:%S UTC')
print("Start time model: ", startTimeStrModel)
# Compute Model grid limits
(nZModel, nYModel, nXModel) = temp3D.shape
lon = np.array(dsModel['x0'])
lat = np.array(dsModel['y0'])
ht = np.array(dsModel['z0'])
dLonModel = lon[1] - lon[0]
dLatModel = lat[1] - lat[0]
minLonModel = lon[0] - dLonModel / 2.0
maxLonModel = lon[-1] + dLonModel / 2.0
minLatModel = lat[0] - dLatModel / 2.0
maxLatModel = lat[-1] + dLatModel / 2.0
minHtModel = ht[0]
maxHtModel = ht[-1]
print("nZModel, nYModel, nXModel", nZModel, nYModel, nXModel)
print("minLonModel, maxLonModel: ", minLonModel, maxLonModel)
print("minLatModel, maxLatModel: ", minLatModel, maxLatModel)
print("minHt, maxHt: ", minHtModel, maxHtModel)
print("Model hts: ", ht)
del lon, lat, ht
<class 'netCDF4._netCDF4.Dataset'>
root group (NETCDF4 data model, file format HDF5):
Conventions: CF-1.6
history: Converted from NetCDF to MDV, 2022/08/21 03:33:17
Ncf:comment:
source: Grib2
title: RUC Rapid Refresh
comment:
dimensions(sizes): time(1), bounds(2), x0(139), y0(72), z0(32), nbytes_mdv_chunk_0000(174)
variables(dimensions): float64 time(time), float64 forecast_reference_time(time), float64 forecast_period(time), float64 start_time(time), float64 stop_time(time), float32 x0(x0), float32 y0(y0), float32 z0(z0), int32 grid_mapping_0(), int32 mdv_master_header(time), int8 mdv_chunk_0000(time, nbytes_mdv_chunk_0000), float32 TMP(time, z0, y0, x0), float32 RH(time, z0, y0, x0), float32 UGRD(time, z0, y0, x0), float32 VGRD(time, z0, y0, x0), float32 VVEL(time, z0, y0, x0), float32 HGT(time, z0, y0, x0), float32 Pressure(time, z0, y0, x0)
groups:
Start time model: 2021/07/06-23:00:00 UTC
nZModel, nYModel, nXModel 32 72 139
minLonModel, maxLonModel: -110.42499923706055 -89.57499313354492
minLatModel, maxLatModel: 34.625 45.42500305175781
minHt, maxHt: 1.2202008 16.17987
Model hts: [ 1.2202008 1.4573147 1.7001446 1.9490081 2.2042506 2.4662497
2.7354188 3.0122116 3.2971282 3.5907218 3.893606 4.2064652
4.530064 4.865264 5.213037 5.574489 5.950885 6.343679
6.75456 7.185502 7.6388354 8.117341 8.624373 9.164038
9.741439 10.363035 11.037209 11.784151 12.630965 13.60854
14.764765 16.17987 ]
Plot W-E and N-S vertical sections of temperature data
# Compute Temp N-S vertical section
nXHalfModel = int(nXModel/2)
tempVertNS = temp3D[:, :, nXHalfModel:(nXHalfModel+1)]
tempVertNS = tempVertNS.reshape(tempVertNS.shape[0], tempVertNS.shape[1])
tempVertNS[tempVertNS == fillValueTemp] = np.nan
print(tempVertNS.shape)
tempNSMax = np.amax(temp3D, (2))
tempNSMax[tempNSMax == fillValueTemp] = np.nan
# Compute Temp W-E vertical section
nYHalfModel = int(nYModel/2)
tempVertWE = temp3D[:, nYHalfModel:(nYHalfModel+1), :]
print(tempVertWE.shape)
tempVertWE = tempVertWE.reshape(tempVertWE.shape[0], tempVertWE.shape[2])
tempVertWE[tempVertWE == fillValueTemp] = np.nan
tempWEMax = np.amax(temp3D, (1))
tempWEMax[tempWEMax == fillValueTemp] = np.nan
print(tempVertWE.shape)
(32, 72)
(32, 1, 139)
(32, 139)
# Plot model temp vertical sections at mid-points of grid
figModelTemp = plt.figure(num=1, figsize=[12, 8], layout='constrained')
# Plot W-E temp
axWETemp = figModelTemp.add_subplot(1, 2, 1,
xlim = (minLonModel, maxLonModel),
ylim = (minHtModel, maxHtModel))
plt.imshow(tempVertWE,
cmap='jet',
interpolation = 'bilinear',
origin = 'lower',
extent = (minLonModel, maxLonModel, minHtModel, maxHtModel))
axWETemp.set_aspect(1.0)
axWETemp.set_xlabel('Longitude (deg)')
axWETemp.set_ylabel('Height (km)')
plt.colorbar(label="Temperature (C)", orientation="horizontal", fraction=0.1)
plt.title("Vert slice mid W-E temp: " + startTimeStrModel)
# Plot N-S temp vertical section
axNSTemp = figModelTemp.add_subplot(1, 2, 2,
xlim = (minLatModel, maxLatModel),
ylim = (minHtModel, maxHtModel))
plt.imshow(tempVertNS,
cmap='jet',
interpolation = 'bilinear',
origin = 'lower',
extent = (minLatModel, maxLatModel, minHtModel, maxHtModel))
axNSTemp.set_aspect(0.52)
axNSTemp.set_xlabel('Latitude (deg)')
axNSTemp.set_ylabel('Height (km)')
plt.colorbar(label="Temperature (C)", orientation="horizontal", fraction=0.1)
plt.title("Vert slice mid N-S temp: " + startTimeStrModel)
Text(0.5, 1.0, 'Vert slice mid N-S temp: 2021/07/06-23:00:00 UTC')
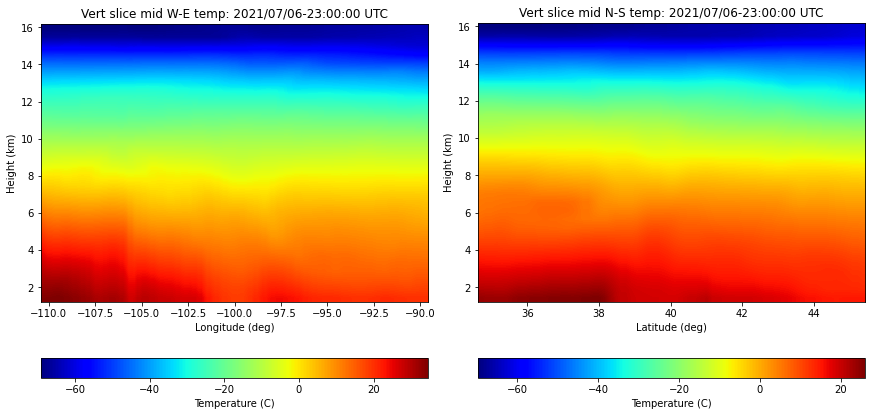
Sample RUC temperatures at 3 radar locations, save in SPDB data base
# Run Mdv2SoundingSpdb to sample temperature data and store in SPDB
!/usr/local/lrose/bin/Mdv2SoundingSpdb -debug -params params/Mdv2SoundingSpdb.ruc -start "2021 07 06 00 00 00" -end "2021 07 07 00 00 00"
======================================================================
Program 'Mdv2SoundingSpdb'
Run-time 2024/02/26 08:57:46.
Copyright (c) 1992 - 2024
University Corporation for Atmospheric Research (UCAR)
National Center for Atmospheric Research (NCAR)
Boulder, Colorado, USA.
Redistribution and use in source and binary forms, with
or without modification, are permitted provided that the following
conditions are met:
1) Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
2) Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
3) Neither the name of UCAR, NCAR nor the names of its contributors, if
any, may be used to endorse or promote products derived from this
software without specific prior written permission.
4) If the software is modified to produce derivative works, such modified
software should be clearly marked, so as not to confuse it with the
version available from UCAR.
======================================================================
Processing file: /tmp/lrose_data/nexrad_mosaic/mdv/ruc/20210706/20210706_230000.mdv.cf.nc
Mdvx::readVsection - reading file: /tmp/lrose_data/nexrad_mosaic/mdv/ruc/20210706/20210706_230000.mdv.cf.nc
SUCCESS - opened file: /tmp/lrose_data/nexrad_mosaic/mdv/ruc/20210706/20210706_230000.mdv.cf.nc
SUCCESS - setting master header
Default time dimension: time
time: 2021/07/06 23:00:00
SUCCESS - setting time coord variable
Ncf2MdvTrans::_shouldAddField
-->> rejecting field: mdv_chunk_0000
Ncf2MdvTrans::_shouldAddField
-->> rejecting field: mdv_chunk_0000
Ncf2MdvTrans::_shouldAddField
-->> adding field: TMP
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: TMP
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: TMP
Adding data field: TMP
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: RH
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: RH
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: RH
Adding data field: RH
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: UGRD
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: UGRD
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: UGRD
Adding data field: UGRD
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: VGRD
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: VGRD
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: VGRD
Adding data field: VGRD
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: VVEL
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: VVEL
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: VVEL
Adding data field: VVEL
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: HGT
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: HGT
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: HGT
Adding data field: HGT
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: Pressure
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: Pressure
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: Pressure
Adding data field: Pressure
time: 2021/07/06 23:00:00
Ncf2MdvTrans::addDataFieldsTime elapsed = 0
Adding chunk: NetCDF file global attributes
Ncf2MdvTrans::addGlobalAttrXmlChunk()
Wrote spdb data, URL: /tmp/lrose_data/nexrad_mosaic/spdb/sounding/ruc
Station name : KGLD
Sounding time: 2021/07/06 23:00:00
Mdvx::readVsection - reading file: /tmp/lrose_data/nexrad_mosaic/mdv/ruc/20210706/20210706_230000.mdv.cf.nc
SUCCESS - opened file: /tmp/lrose_data/nexrad_mosaic/mdv/ruc/20210706/20210706_230000.mdv.cf.nc
SUCCESS - setting master header
Default time dimension: time
time: 2021/07/06 23:00:00
SUCCESS - setting time coord variable
Ncf2MdvTrans::_shouldAddField
-->> rejecting field: mdv_chunk_0000
Ncf2MdvTrans::_shouldAddField
-->> rejecting field: mdv_chunk_0000
Ncf2MdvTrans::_shouldAddField
-->> adding field: TMP
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: TMP
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: TMP
Adding data field: TMP
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: RH
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: RH
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: RH
Adding data field: RH
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: UGRD
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: UGRD
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: UGRD
Adding data field: UGRD
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: VGRD
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: VGRD
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: VGRD
Adding data field: VGRD
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: VVEL
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: VVEL
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: VVEL
Adding data field: VVEL
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: HGT
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: HGT
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: HGT
Adding data field: HGT
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: Pressure
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: Pressure
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: Pressure
Adding data field: Pressure
time: 2021/07/06 23:00:00
Ncf2MdvTrans::addDataFieldsTime elapsed = 0
Adding chunk: NetCDF file global attributes
Ncf2MdvTrans::addGlobalAttrXmlChunk()
Wrote spdb data, URL: /tmp/lrose_data/nexrad_mosaic/spdb/sounding/ruc
Station name : KDDC
Sounding time: 2021/07/06 23:00:00
Mdvx::readVsection - reading file: /tmp/lrose_data/nexrad_mosaic/mdv/ruc/20210706/20210706_230000.mdv.cf.nc
SUCCESS - opened file: /tmp/lrose_data/nexrad_mosaic/mdv/ruc/20210706/20210706_230000.mdv.cf.nc
SUCCESS - setting master header
Default time dimension: time
time: 2021/07/06 23:00:00
SUCCESS - setting time coord variable
Ncf2MdvTrans::_shouldAddField
-->> rejecting field: mdv_chunk_0000
Ncf2MdvTrans::_shouldAddField
-->> rejecting field: mdv_chunk_0000
Ncf2MdvTrans::_shouldAddField
-->> adding field: TMP
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: TMP
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: TMP
Adding data field: TMP
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: RH
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: RH
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: RH
Adding data field: RH
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: UGRD
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: UGRD
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: UGRD
Adding data field: UGRD
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: VGRD
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: VGRD
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: VGRD
Adding data field: VGRD
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: VVEL
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: VVEL
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: VVEL
Adding data field: VVEL
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: HGT
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: HGT
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: HGT
Adding data field: HGT
time: 2021/07/06 23:00:00
Ncf2MdvTrans::_shouldAddField
-->> adding field: Pressure
Ncf2MdvTrans::_shouldAddField
Checking variable for field data: Pressure
SUCCESS - FIELD has X coordinate
SUCCESS - FIELD has Y coordinate
NOTE - FIELD has Z coordinate
Ncf2MdvTrans::_addOneField
-->> adding field: Pressure
Adding data field: Pressure
time: 2021/07/06 23:00:00
Ncf2MdvTrans::addDataFieldsTime elapsed = 0
Adding chunk: NetCDF file global attributes
Ncf2MdvTrans::addGlobalAttrXmlChunk()
Wrote spdb data, URL: /tmp/lrose_data/nexrad_mosaic/spdb/sounding/ruc
Station name : KUEX
Sounding time: 2021/07/06 23:00:00
List sounding data base
# List SPDB files
!ls -alR /tmp/lrose_data/nexrad_mosaic/spdb/sounding/ruc/20210706*
-rw-r--r-- 1 root root 4704 Feb 26 08:57 /tmp/lrose_data/nexrad_mosaic/spdb/sounding/ruc/20210706.data
-rw-r--r-- 1 root root 6360 Feb 26 08:57 /tmp/lrose_data/nexrad_mosaic/spdb/sounding/ruc/20210706.indx
Run RadxRate on the CfRadial files
RadxRate will compute:
KDP - specific differential phase
KDP_SC - KDP conditioned using Z and ZDR self-consistency
PID - NCAR Particle ID (type)
RATE_ZH - precip rate from standard ZR relationship
RATE_HYBRID - precip rate from NCAR hybrid estimator
RadxRate has a main parameter file, which then specifies a parameter file computing each of KDP, PID and precip rate.
The PID step requires an additional parameter file specifying the fuzzy logic thresholds.
The parameter files used here are:
kdp_params.nexrad
pid_params.nexrad
pid_thresholds.nexrad
rate_params.nexrad
# Run RadxRate for 3 NEXRAD radars
for radar_name in ['KGLD', 'KUEX', 'KDDC']:
# Set radar in name environment variable
os.environ['RADAR_NAME'] = radar_name
# Run RadxRate using param file
!/usr/local/lrose/bin/RadxRate -params ./params/RadxRate.nexrad -debug -start "2021 07 06 22 00 00" -end "2021 07 06 22 30 00"
RadxRate::_runArchive
Input dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD
Start time: 2021/07/06 22:00:00
End time: 2021/07/06 22:30:00
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:00:03
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937876.593232.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937876.593232.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220448.793_to_20210706_220926.383_KGLD_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:04:48
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220448.793_to_20210706_220926.383_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937885.685647.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937885.685647.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220448.793_to_20210706_220926.383_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220448.793_to_20210706_220926.383_KGLD_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_220935.631_to_20210706_221411.627_KGLD_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:09:35
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220935.631_to_20210706_221411.627_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937894.690504.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937894.690504.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220935.631_to_20210706_221411.627_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220935.631_to_20210706_221411.627_KGLD_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_221420.555_to_20210706_221857.324_KGLD_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:14:20
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_221420.555_to_20210706_221857.324_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937903.680136.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937903.680136.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_221420.555_to_20210706_221857.324_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_221420.555_to_20210706_221857.324_KGLD_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_221906.199_to_20210706_222341.850_KGLD_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:19:06
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_221906.199_to_20210706_222341.850_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937912.660498.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937912.660498.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_221906.199_to_20210706_222341.850_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_221906.199_to_20210706_222341.850_KGLD_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_222350.154_to_20210706_222826.584_KGLD_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:23:50
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_222350.154_to_20210706_222826.584_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937921.607988.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937921.607988.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_222350.154_to_20210706_222826.584_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_222350.154_to_20210706_222826.584_KGLD_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KGLD/20210706/cfrad.20210706_222834.963_to_20210706_223310.845_KGLD_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:28:34
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_222834.963_to_20210706_223310.845_KGLD_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937930.554045.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/tmp.756.1708937930.554045.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_222834.963_to_20210706_223310.845_KGLD_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_222834.963_to_20210706_223310.845_KGLD_SUR.nc
RadxRate::_runArchive
Input dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX
Start time: 2021/07/06 22:00:00
End time: 2021/07/06 22:30:00
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_220249.032_to_20210706_220715.866_KUEX_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:02:49
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_220249.032_to_20210706_220715.866_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937940.899845.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937940.899845.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_220249.032_to_20210706_220715.866_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_220249.032_to_20210706_220715.866_KUEX_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_220723.969_to_20210706_221157.362_KUEX_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:07:23
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_220723.969_to_20210706_221157.362_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937949.885110.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937949.885110.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_220723.969_to_20210706_221157.362_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_220723.969_to_20210706_221157.362_KUEX_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_221204.520_to_20210706_221625.502_KUEX_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:12:04
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_221204.520_to_20210706_221625.502_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937958.842928.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937958.842928.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_221204.520_to_20210706_221625.502_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_221204.520_to_20210706_221625.502_KUEX_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_221633.850_to_20210706_222054.868_KUEX_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:16:33
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_221633.850_to_20210706_222054.868_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937967.772199.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937967.772199.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_221633.850_to_20210706_222054.868_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_221633.850_to_20210706_222054.868_KUEX_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_222102.216_to_20210706_222523.504_KUEX_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:21:02
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_222102.216_to_20210706_222523.504_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937976.702679.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937976.702679.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_222102.216_to_20210706_222523.504_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_222102.216_to_20210706_222523.504_KUEX_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KUEX/20210706/cfrad.20210706_222531.244_to_20210706_222952.818_KUEX_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:25:31
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_222531.244_to_20210706_222952.818_KUEX_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937985.572341.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/tmp.763.1708937985.572341.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_222531.244_to_20210706_222952.818_KUEX_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_222531.244_to_20210706_222952.818_KUEX_SUR.nc
RadxRate::_runArchive
Input dir: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC
Start time: 2021/07/06 22:00:00
End time: 2021/07/06 22:30:00
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220000.765_to_20210706_220422.888_KDDC_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:00:00
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220000.765_to_20210706_220422.888_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708937995.649133.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708937995.649133.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220000.765_to_20210706_220422.888_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220000.765_to_20210706_220422.888_KDDC_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220430.757_to_20210706_220912.758_KDDC_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:04:30
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220430.757_to_20210706_220912.758_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708938004.673416.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708938004.673416.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220430.757_to_20210706_220912.758_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220430.757_to_20210706_220912.758_KDDC_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_220921.610_to_20210706_221350.957_KDDC_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:09:21
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220921.610_to_20210706_221350.957_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708938013.464464.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708938013.464464.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220921.610_to_20210706_221350.957_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220921.610_to_20210706_221350.957_KDDC_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_221600.999_to_20210706_222043.450_KDDC_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:16:00
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_221600.999_to_20210706_222043.450_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708938022.460707.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708938022.460707.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_221600.999_to_20210706_222043.450_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_221600.999_to_20210706_222043.450_KDDC_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_222051.218_to_20210706_222526.565_KDDC_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:20:51
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_222051.218_to_20210706_222526.565_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708938031.497967.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708938031.497967.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_222051.218_to_20210706_222526.565_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_222051.218_to_20210706_222526.565_KDDC_SUR.nc
INFO - RadxRate::Run
Input path: /tmp/lrose_data/nexrad_mosaic/cfradial/moments/KDDC/20210706/cfrad.20210706_222533.934_to_20210706_223025.212_KDDC_SUR.nc
Thread #: 0
Loading temp profile for time: 2021/07/06 22:25:33
INFO: RadxFile::writeToDir
Writing CfRadial file to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToDir
Writing to dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
DEBUG - NcfRadxFile::writeToPath
Writing to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_222533.934_to_20210706_223025.212_KDDC_SUR.nc
Tmp path is: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708938040.511812.tmp
Writing fields and compressing ...
... writing field: RATE_ZH
... writing field: RATE_HYBRID
... writing field: PID
... writing field: KDP
... writing field: DBZ
DEBUG - NcfRadxFile::writeToPath
Renamed tmp path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/tmp.770.1708938040.511812.tmp
to final path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_222533.934_to_20210706_223025.212_KDDC_SUR.nc
INFO: RadxFile::writeToDir
Wrote CfRadial file to path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_222533.934_to_20210706_223025.212_KDDC_SUR.nc
List files created by RadxRate
# List the CfRadial files created by RadxRate
!ls -R ${NEXRAD_DATA_DIR}/cfradial/rate/K*/2*
/tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706:
cfrad.20210706_220000.765_to_20210706_220422.888_KDDC_SUR.nc
cfrad.20210706_220430.757_to_20210706_220912.758_KDDC_SUR.nc
cfrad.20210706_220921.610_to_20210706_221350.957_KDDC_SUR.nc
cfrad.20210706_221600.999_to_20210706_222043.450_KDDC_SUR.nc
cfrad.20210706_222051.218_to_20210706_222526.565_KDDC_SUR.nc
cfrad.20210706_222533.934_to_20210706_223025.212_KDDC_SUR.nc
/tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706:
cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc
cfrad.20210706_220448.793_to_20210706_220926.383_KGLD_SUR.nc
cfrad.20210706_220935.631_to_20210706_221411.627_KGLD_SUR.nc
cfrad.20210706_221420.555_to_20210706_221857.324_KGLD_SUR.nc
cfrad.20210706_221906.199_to_20210706_222341.850_KGLD_SUR.nc
cfrad.20210706_222350.154_to_20210706_222826.584_KGLD_SUR.nc
cfrad.20210706_222834.963_to_20210706_223310.845_KGLD_SUR.nc
/tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706:
cfrad.20210706_220249.032_to_20210706_220715.866_KUEX_SUR.nc
cfrad.20210706_220723.969_to_20210706_221157.362_KUEX_SUR.nc
cfrad.20210706_221204.520_to_20210706_221625.502_KUEX_SUR.nc
cfrad.20210706_221633.850_to_20210706_222054.868_KUEX_SUR.nc
cfrad.20210706_222102.216_to_20210706_222523.504_KUEX_SUR.nc
cfrad.20210706_222531.244_to_20210706_222952.818_KUEX_SUR.nc
Plot PID and rate results for NEXRAD Goodland radar (KGLD)
# Read CfRadial file into radar object
filePathRate = os.path.join(nexradDataDir, "cfradial/rate/KGLD/20210706/cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc")
rate_kgld = pyart.io.read_cfradial(filePathRate)
rate_kgld.info('compact')
altitude: <ndarray of type: float64 and shape: (1,)>
altitude_agl: <ndarray of type: float64 and shape: (1,)>
antenna_transition: <ndarray of type: int8 and shape: (6120,)>
azimuth: <ndarray of type: float32 and shape: (6120,)>
elevation: <ndarray of type: float32 and shape: (6120,)>
fields:
RATE_ZH: <ndarray of type: float32 and shape: (6120, 912)>
RATE_HYBRID: <ndarray of type: float32 and shape: (6120, 912)>
PID: <ndarray of type: float32 and shape: (6120, 912)>
KDP: <ndarray of type: float32 and shape: (6120, 912)>
DBZ: <ndarray of type: float32 and shape: (6120, 912)>
fixed_angle: <ndarray of type: float32 and shape: (14,)>
instrument_parameters:
follow_mode: <ndarray of type: |S1 and shape: (14, 32)>
pulse_width: <ndarray of type: float32 and shape: (6120,)>
prt_mode: <ndarray of type: |S1 and shape: (14, 32)>
prt: <ndarray of type: float32 and shape: (6120,)>
prt_ratio: <ndarray of type: float32 and shape: (6120,)>
polarization_mode: <ndarray of type: |S1 and shape: (14, 32)>
nyquist_velocity: <ndarray of type: float32 and shape: (6120,)>
unambiguous_range: <ndarray of type: float32 and shape: (6120,)>
n_samples: <ndarray of type: int32 and shape: (6120,)>
radar_antenna_gain_h: <ndarray of type: float32 and shape: (1,)>
radar_antenna_gain_v: <ndarray of type: float32 and shape: (1,)>
radar_beam_width_h: <ndarray of type: float32 and shape: (1,)>
radar_beam_width_v: <ndarray of type: float32 and shape: (1,)>
radar_rx_bandwidth: <ndarray of type: float32 and shape: (1,)>
measured_transmit_power_v: <ndarray of type: float32 and shape: (6120,)>
measured_transmit_power_h: <ndarray of type: float32 and shape: (6120,)>
latitude: <ndarray of type: float64 and shape: (1,)>
longitude: <ndarray of type: float64 and shape: (1,)>
nsweeps: 14
ngates: 912
nrays: 6120
radar_calibration:
r_calib_time: <ndarray of type: |S1 and shape: (1, 32)>
r_calib_pulse_width: <ndarray of type: float32 and shape: (1,)>
r_calib_xmit_power_h: <ndarray of type: float32 and shape: (1,)>
r_calib_xmit_power_v: <ndarray of type: float32 and shape: (1,)>
r_calib_two_way_waveguide_loss_h: <ndarray of type: float32 and shape: (1,)>
r_calib_two_way_waveguide_loss_v: <ndarray of type: float32 and shape: (1,)>
r_calib_two_way_radome_loss_h: <ndarray of type: float32 and shape: (1,)>
r_calib_two_way_radome_loss_v: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_mismatch_loss: <ndarray of type: float32 and shape: (1,)>
r_calib_k_squared_water: <ndarray of type: float32 and shape: (1,)>
r_calib_radar_constant_h: <ndarray of type: float32 and shape: (1,)>
r_calib_radar_constant_v: <ndarray of type: float32 and shape: (1,)>
r_calib_antenna_gain_h: <ndarray of type: float32 and shape: (1,)>
r_calib_antenna_gain_v: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_i0_dbm_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_i0_dbm_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_i0_dbm_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_i0_dbm_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_gain_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_gain_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_gain_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_gain_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_slope_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_slope_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_slope_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_receiver_slope_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_dynamic_range_db_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_dynamic_range_db_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_dynamic_range_db_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_dynamic_range_db_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_base_dbz_1km_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_base_dbz_1km_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_base_dbz_1km_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_base_dbz_1km_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_sun_power_hc: <ndarray of type: float32 and shape: (1,)>
r_calib_sun_power_vc: <ndarray of type: float32 and shape: (1,)>
r_calib_sun_power_hx: <ndarray of type: float32 and shape: (1,)>
r_calib_sun_power_vx: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_source_power_h: <ndarray of type: float32 and shape: (1,)>
r_calib_noise_source_power_v: <ndarray of type: float32 and shape: (1,)>
r_calib_power_measure_loss_h: <ndarray of type: float32 and shape: (1,)>
r_calib_power_measure_loss_v: <ndarray of type: float32 and shape: (1,)>
r_calib_coupler_forward_loss_h: <ndarray of type: float32 and shape: (1,)>
r_calib_coupler_forward_loss_v: <ndarray of type: float32 and shape: (1,)>
r_calib_dbz_correction: <ndarray of type: float32 and shape: (1,)>
r_calib_zdr_correction: <ndarray of type: float32 and shape: (1,)>
r_calib_ldr_correction_h: <ndarray of type: float32 and shape: (1,)>
r_calib_ldr_correction_v: <ndarray of type: float32 and shape: (1,)>
r_calib_system_phidp: <ndarray of type: float32 and shape: (1,)>
r_calib_test_power_h: <ndarray of type: float32 and shape: (1,)>
r_calib_test_power_v: <ndarray of type: float32 and shape: (1,)>
r_calib_index: <ndarray of type: int32 and shape: (6120,)>
range: <ndarray of type: float32 and shape: (912,)>
scan_rate: <ndarray of type: float32 and shape: (6120,)>
scan_type: other
sweep_end_ray_index: <ndarray of type: int32 and shape: (14,)>
sweep_mode: <ndarray of type: |S1 and shape: (14, 32)>
sweep_number: <ndarray of type: int32 and shape: (14,)>
sweep_start_ray_index: <ndarray of type: int32 and shape: (14,)>
target_scan_rate: <ndarray of type: float32 and shape: (14,)>
time: <ndarray of type: float64 and shape: (6120,)>
metadata:
Conventions: CF-1.7
Sub_conventions: CF-Radial instrument_parameters radar_parameters radar_calibration
version: CF-Radial-1.4
title:
institution:
references:
source: ARCHIVE 2 data
history:
comment:
original_format: NEXRAD
driver: RadxConvert(NCAR)
created: 2024/02/26 08:56:17.249
start_datetime: 2021-07-06T22:00:03Z
time_coverage_start: 2021-07-06T22:00:03Z
start_time: 2021-07-06 22:00:03.963
end_datetime: 2021-07-06T22:04:39Z
time_coverage_end: 2021-07-06T22:04:39Z
end_time: 2021-07-06 22:04:39.770
instrument_name: KGLD
site_name: DLGK
scan_name: Surveillance
scan_id: 212
platform_is_mobile: false
n_gates_vary: false
ray_times_increase: true
volume_number: 79
platform_type: fixed
instrument_type: radar
primary_axis: axis_z
# Plot results of RadxRate
displayRate = pyart.graph.RadarDisplay(rate_kgld)
figRate = plt.figure(1, (12, 10))
# DBZ (input)
axDbz = figRate.add_subplot(221)
displayRate.plot_ppi('DBZ', 0, vmin=-32, vmax=64.,
axislabels=("x(km)", "y(km)"),
colorbar_label="DBZ")
displayRate.plot_range_rings([50, 100, 150, 200])
displayRate.plot_cross_hair(200.)
# KDP (computed)
axKdp = figRate.add_subplot(222)
displayRate.plot_ppi('KDP', 0, vmin=0, vmax=2.,
axislabels=("x(km)", "y(km)"),
colorbar_label="KDP (deg/km)",
cmap="rainbow")
displayRate.plot_range_rings([50, 100, 150, 200])
displayRate.plot_cross_hair(200.)
# RATE_HYBRID (computed)
axHybrid = figRate.add_subplot(223)
displayRate.plot_ppi('RATE_HYBRID', 0, vmin=0, vmax=50.,
axislabels=("x(km)", "y(km)"),
colorbar_label="RATE_HYBRID(mm/hr)",
cmap = "rainbow")
displayRate.plot_range_rings([50, 100, 150, 200])
displayRate.plot_cross_hair(200.)
# NCAR PID (computed)
axPID = figRate.add_subplot(224)
displayRate.plot_ppi('PID', 0,
axislabels=("x(km)", "y(km)"),
colorbar_label="PID",
cmap = "rainbow")
displayRate.plot_range_rings([50, 100, 150, 200])
displayRate.plot_cross_hair(200.)
pid_cbar = displayRate.cbs[3]
pid_cbar.set_ticks([1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17])
pid_cbar.set_ticklabels(['cld-drops', 'drizzle', 'lt-rain', 'mod-rain', 'hvy-rain', 'hail', 'rain/hail', 'sm-hail', 'gr/rain', 'dry-snow', 'wet-snow', 'ice', 'irreg-ice', 'slw', 'insects', '2nd-trip', 'clutter'])
figRate.tight_layout()
plt.show()
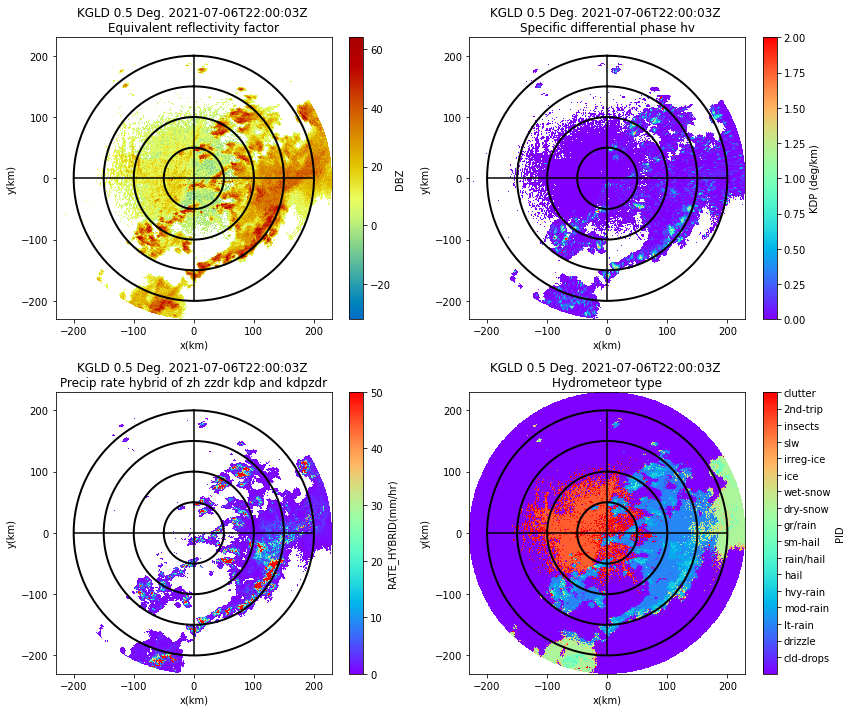
Convert CfRadial polar files to Cartesian
# Run Radx2Grid for 3 NEXRAD radars
for radar_name in ['KGLD', 'KUEX', 'KDDC']:
# Set radar in name environment variable
os.environ['RADAR_NAME'] = radar_name
# Run RadxRate using param file
!/usr/local/lrose/bin/Radx2Grid -params ./params/Radx2Grid.rate -debug -start "2021 07 06 22 00 00" -end "2021 07 06 22 30 00"
======================================================================
Program 'Radx2Grid'
Run-time 2024/02/26 09:00:44.
Copyright (c) 1992 - 2024
University Corporation for Atmospheric Research (UCAR)
National Center for Atmospheric Research (NCAR)
Boulder, Colorado, USA.
Redistribution and use in source and binary forms, with
or without modification, are permitted provided that the following
conditions are met:
1) Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
2) Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
3) Neither the name of UCAR, NCAR nor the names of its contributors, if
any, may be used to endorse or promote products derived from this
software without specific prior written permission.
4) If the software is modified to produce derivative works, such modified
software should be clearly marked, so as not to confuse it with the
version available from UCAR.
======================================================================
Running Radx2Grid in ARCHIVE mode
Input dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD
Start time: 2021/07/06 22:00:00
End time: 2021/07/06 22:30:00
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220003.963_to_20210706_220439.770_KGLD_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.343796
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.000119
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.123002
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000244
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 9.9e-05
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 1.4e-05
TIMING, task: Filling search matrix, secs used: 0.20521
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 6.1e-05
TIMING, task: Computing grid relative to radar, secs used: 1.65775
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 4.8e-05
TIMING, task: Interpolating, secs used: 1.35794
TIMING, task: Cart interp - before _writeOutputFile, secs used: 6.3e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_220003.nc
TIMING, task: Writing output files, secs used: 1.3292
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220448.793_to_20210706_220926.383_KGLD_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.549369
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.178548
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.123368
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000252
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 9.1e-05
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 2.6e-05
TIMING, task: Filling search matrix, secs used: 0.195028
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 7.5e-05
TIMING, task: Computing grid relative to radar, secs used: 1.6348
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 7e-05
TIMING, task: Interpolating, secs used: 1.32771
TIMING, task: Cart interp - before _writeOutputFile, secs used: 4.5e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_220448.nc
TIMING, task: Writing output files, secs used: 1.29946
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_220935.631_to_20210706_221411.627_KGLD_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.554999
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.163893
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.112151
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000233
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 9.2e-05
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 2.4e-05
TIMING, task: Filling search matrix, secs used: 0.187344
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 5.6e-05
TIMING, task: Computing grid relative to radar, secs used: 1.64057
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 4e-05
TIMING, task: Interpolating, secs used: 1.33401
TIMING, task: Cart interp - before _writeOutputFile, secs used: 3.9e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_220935.nc
TIMING, task: Writing output files, secs used: 1.29697
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_221420.555_to_20210706_221857.324_KGLD_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.545917
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.16451
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.111207
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000243
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000122
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 2.1e-05
TIMING, task: Filling search matrix, secs used: 0.175894
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 8.2e-05
TIMING, task: Computing grid relative to radar, secs used: 1.63977
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 5.2e-05
TIMING, task: Interpolating, secs used: 1.32484
TIMING, task: Cart interp - before _writeOutputFile, secs used: 6.8e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_221420.nc
TIMING, task: Writing output files, secs used: 1.293
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_221906.199_to_20210706_222341.850_KGLD_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.54779
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.16421
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.111837
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000269
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000123
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 2e-05
TIMING, task: Filling search matrix, secs used: 0.188867
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 5.4e-05
TIMING, task: Computing grid relative to radar, secs used: 1.64048
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 5.1e-05
TIMING, task: Interpolating, secs used: 1.32258
TIMING, task: Cart interp - before _writeOutputFile, secs used: 4.4e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_221906.nc
TIMING, task: Writing output files, secs used: 1.30141
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_222350.154_to_20210706_222826.584_KGLD_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.547801
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.164062
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.111464
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000287
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000107
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 1.3e-05
TIMING, task: Filling search matrix, secs used: 0.178924
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 6.2e-05
TIMING, task: Computing grid relative to radar, secs used: 1.63525
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 4.1e-05
TIMING, task: Interpolating, secs used: 1.33221
TIMING, task: Cart interp - before _writeOutputFile, secs used: 5.3e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_222350.nc
TIMING, task: Writing output files, secs used: 1.2995
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KGLD/20210706/cfrad.20210706_222834.963_to_20210706_223310.845_KGLD_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.546631
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.164184
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.114968
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.00025
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000108
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 2.1e-05
TIMING, task: Filling search matrix, secs used: 0.186268
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 5.4e-05
TIMING, task: Computing grid relative to radar, secs used: 1.63458
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used:
4.1e-05
TIMING, task: Interpolating, secs used: 1.3547
TIMING, task: Cart interp - before _writeOutputFile, secs used: 4.4e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_222834.nc
TIMING, task: Writing output files, secs used: 1.30158
======================================================================
Program 'Radx2Grid'
Run-time 2024/02/26 09:01:22.
Copyright (c) 1992 - 2024
University Corporation for Atmospheric Research (UCAR)
National Center for Atmospheric Research (NCAR)
Boulder, Colorado, USA.
Redistribution and use in source and binary forms, with
or without modification, are permitted provided that the following
conditions are met:
1) Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
2) Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
3) Neither the name of UCAR, NCAR nor the names of its contributors, if
any, may be used to endorse or promote products derived from this
software without specific prior written permission.
4) If the software is modified to produce derivative works, such modified
software should be clearly marked, so as not to confuse it with the
version available from UCAR.
======================================================================
Running Radx2Grid in ARCHIVE mode
Input dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX
Start time: 2021/07/06 22:00:00
End time: 2021/07/06 22:30:00
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_220249.032_to_20210706_220715.866_KUEX_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.342156
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.000137
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.123535
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000281
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000103
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 2.1e-05
TIMING, task: Filling search matrix, secs used: 0.198811
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 6.6e-05
TIMING, task: Computing grid relative to radar, secs used: 1.63091
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 5.4e-05
TIMING, task: Interpolating, secs used: 1.46338
TIMING, task: Cart interp - before _writeOutputFile, secs used: 4.9e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_220249.nc
TIMING, task: Writing output files, secs used: 1.30359
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_220723.969_to_20210706_221157.362_KUEX_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.553607
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.175919
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.122474
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000262
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000116
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 2.9e-05
TIMING, task: Filling search matrix, secs used: 0.193802
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 5.5e-05
TIMING, task: Computing grid relative to radar, secs used: 1.64728
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 3.8e-05
TIMING, task: Interpolating, secs used: 1.43093
TIMING, task: Cart interp - before _writeOutputFile, secs used: 4.7e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_220723.nc
TIMING, task: Writing output files, secs used: 1.28629
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_221204.520_to_20210706_221625.502_KUEX_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.553609
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.163882
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.111954
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000217
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 8.4e-05
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 1.8e-05
TIMING, task: Filling search matrix, secs used: 0.180958
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 5.4e-05
TIMING, task: Computing grid relative to radar, secs used: 1.64178
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 5.5e-05
TIMING, task: Interpolating, secs used: 1.44539
TIMING, task: Cart interp - before _writeOutputFile, secs used: 7.7e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_221204.nc
TIMING, task: Writing output files, secs used: 1.29286
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_221633.850_to_20210706_222054.868_KUEX_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.548838
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.164459
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.111337
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000253
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000104
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 1.5e-05
TIMING, task: Filling search matrix, secs used: 0.180636
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 4.6e-05
TIMING, task: Computing grid relative to radar, secs used: 1.64382
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 5e-05
TIMING, task: Interpolating, secs used: 1.44178
TIMING, task: Cart interp - before _writeOutputFile, secs used: 6.4e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_221633.nc
TIMING, task: Writing output files, secs used: 1.27319
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_222102.216_to_20210706_222523.504_KUEX_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.551414
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.164516
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.111421
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000249
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000113
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 2.7e-05
TIMING, task: Filling search matrix, secs used: 0.190138
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 6.7e-05
TIMING, task: Computing grid relative to radar, secs used: 1.65052
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 7.4e-05
TIMING, task: Interpolating, secs used: 1.43769
TIMING, task: Cart interp - before _writeOutputFile, secs used: 4.3e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_222102.nc
TIMING, task: Writing output files, secs used: 1.28675
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KUEX/20210706/cfrad.20210706_222531.244_to_20210706_222952.818_KUEX_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.567437
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.164316
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.11106
_scanDeltaAz: 1
_scanDeltaEl: 3.91113
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000258
_searchRadiusEl: 5.80113
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000108
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 2.4e-05
TIMING, task: Filling search matrix, secs used: 0.175721
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 7.6e-05
TIMING, task: Computing grid relative to radar, secs used: 1.64834
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 4.5e-05
TIMING, task: Interpolating, secs used: 1.43959
TIMING, task: Cart interp - before _writeOutputFile, secs used: 0.000101
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_222531.nc
TIMING, task: Writing output files, secs used: 1.28868
======================================================================
Program 'Radx2Grid'
Run-time 2024/02/26 09:01:55.
Copyright (c) 1992 - 2024
University Corporation for Atmospheric Research (UCAR)
National Center for Atmospheric Research (NCAR)
Boulder, Colorado, USA.
Redistribution and use in source and binary forms, with
or without modification, are permitted provided that the following
conditions are met:
1) Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
2) Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
3) Neither the name of UCAR, NCAR nor the names of its contributors, if
any, may be used to endorse or promote products derived from this
software without specific prior written permission.
4) If the software is modified to produce derivative works, such modified
software should be clearly marked, so as not to confuse it with the
version available from UCAR.
======================================================================
Running Radx2Grid in ARCHIVE mode
Input dir: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC
Start time: 2021/07/06 22:00:00
End time: 2021/07/06 22:30:00
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220000.765_to_20210706_220422.888_KDDC_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.357243
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.00012
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.122098
_scanDeltaAz: 0.6
_scanDeltaEl: 2.02148
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000251
_searchRadiusEl: 3.91148
_searchRadiusAz: 2.49
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 25
TIMING, task: Computing search limits, secs used: 0.000107
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 1.3e-05
TIMING, task: Filling search matrix, secs used: 0.11144
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 6.6e-05
TIMING, task: Computing grid relative to radar, secs used: 1.65071
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 7e-05
TIMING, task: Interpolating, secs used: 1.36112
TIMING, task: Cart interp - before _writeOutputFile, secs used: 4.4e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_220000.nc
TIMING, task: Writing output files, secs used: 1.31241
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220430.757_to_20210706_220912.758_KDDC_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.566128
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.214179
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.123597
_scanDeltaAz: 1
_scanDeltaEl: 2.46094
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000281
_searchRadiusEl: 4.35094
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000112
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 2.6e-05
TIMING, task: Filling search matrix, secs used: 0.133449
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative
, secs used: 6.6e-05
TIMING, task: Computing grid relative to radar, secs used: 1.64777
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 5.1e-05
TIMING, task: Interpolating, secs used: 1.37033
TIMING, task: Cart interp - before _writeOutputFile, secs used: 6.6e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_220430.nc
TIMING, task: Writing output files, secs used: 1.30478
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_220921.610_to_20210706_221350.957_KDDC_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.541639
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.164533
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.112281
_scanDeltaAz: 0.6
_scanDeltaEl: 2.63672
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000246
_searchRadiusEl: 4.52672
_searchRadiusAz: 2.49
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 25
TIMING, task: Computing search limits, secs used: 0.000102
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 1.8e-05
TIMING, task: Filling search matrix, secs used: 0.09974
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 6e-05
TIMING, task: Computing grid relative to radar, secs used: 1.65141
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 7.2e-05
TIMING, task: Interpolating, secs used: 1.31209
TIMING, task: Cart interp - before _writeOutputFile, secs used: 4.2e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_220921.nc
TIMING, task: Writing output files, secs used: 1.3053
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_221600.999_to_20210706_222043.450_KDDC_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.558003
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.164061
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.111251
_scanDeltaAz: 1
_scanDeltaEl: 2.46094
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000249
_searchRadiusEl: 4.35094
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000107
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 1.6e-05
TIMING, task: Filling search matrix, secs used: 0.12216
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 4.6e-05
TIMING, task: Computing grid relative to radar, secs used: 1.64259
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 6.1e-05
TIMING, task: Interpolating, secs used: 1.38129
TIMING, task: Cart interp - before _writeOutputFile, secs used: 4.4e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_221600.nc
TIMING, task: Writing output files, secs used: 1.30219
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_222051.218_to_20210706_222526.565_KDDC_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.55599
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.163995
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.111437
_scanDeltaAz: 1
_scanDeltaEl: 2.63672
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.000252
_searchRadiusEl: 4.52672
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 0.000112
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 1.6e-05
TIMING, task: Filling search matrix, secs used: 0.116885
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 5.4e-05
TIMING, task: Computing grid relative to radar, secs used: 1.64254
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 4.6e-05
TIMING, task: Interpolating, secs used: 1.38532
TIMING, task: Cart interp - before _writeOutputFile, secs used: 7.9e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_222051.nc
TIMING, task: Writing output files, secs used: 1.28428
INFO - Radx2Grid::_processFile
Input file path: /tmp/lrose_data/nexrad_mosaic/cfradial/rate/KDDC/20210706/cfrad.20210706_222533.934_to_20210706_223025.212_KDDC_SUR.nc
Reading in file ...
TIMING, task: Cart interp - reading data, secs used: 0.55596
TIMING, task: Cart interp - before _initOutputArrays, secs used: 0.164537
TIMING, task: Cart interp - after _initOutputArrays, secs used: 0.111567
_scanDeltaAz: 1
_scanDeltaEl: 2.46094
_isSector: 0
_spansNorth: N
TIMING, task: Cart interp - before computeSearchLimits, secs used: 0.00022
_searchRadiusEl: 4.35094
_searchRadiusAz: 2.89
_searchMinAz: 0
_searchNAz: 3801
_searchMaxDistAz: 29
TIMING, task: Computing search limits, secs used: 9.2e-05
Filling search matrix ...
TIMING, task: Cart interp - before fillSearchMatrix, secs used: 2.5e-05
TIMING, task: Filling search matrix, secs used: 0.114278
Computing grid relative to radar ...
TIMING, task: Cart interp - before _computeGridRelative, secs used: 6e-05
TIMING, task: Computing grid relative to radar, secs used: 1.6429
Interpolating ...
TIMING, task: Cart interp - before doInterp, secs used: 6.1e-05
TIMING, task: Interpolating, secs used: 1.38364
TIMING, task: Cart interp - before _writeOutputFile, secs used: 5e-05
Writing output file ...
Adding field: RATE_HYBRID
Adding field: PID
Adding field: DBZ
Adding field: range
Adding field: Coverage
Mdv2NcfTrans::addGlobalAttributes()
Mdv2NcfTrans::addDimensions()
Mdv2NetCDF::_addTimeVariables()
Mdv2NcfTrans::addCoordinateVariables()
Mdv2NcfTrans::addFieldVariables()
adding field: RATE_HYBRID
NcfFieldData::_setChunking()
Field: RATE_HYBRID
nyChunk: 460
nxChunk: 460
adding field: PID
NcfFieldData::_setChunking()
Field: PID
nyChunk: 460
nxChunk: 460
adding field: DBZ
NcfFieldData::_setChunking()
Field: DBZ
nyChunk: 460
nxChunk: 460
adding field: range
NcfFieldData::_setChunking()
Field: range
nyChunk: 460
nxChunk: 460
adding field: Coverage
NcfFieldData::_setChunking()
Field: Coverage
nyChunk: 460
nxChunk: 460
Mdv2NcfTrans::_putTimeVariables()
Mdv2NcfTrans::_putCoordinateVariables()
Mdv2NcfTrans::_putFieldDataVariables()
OutputMdv::_writeLdataInfo(): Data written to /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_222533.nc
TIMING, task: Writing output files, secs used: 1.29369
List files created by Radx2Grid
# List the Cartesian files created by Radx2Grid
!ls -R ${NEXRAD_DATA_DIR}/mdv/radarCart/K*/2*
/tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706:
ncf_20210706_220000.nc ncf_20210706_220921.nc ncf_20210706_222051.nc
ncf_20210706_220430.nc ncf_20210706_221600.nc ncf_20210706_222533.nc
/tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706:
ncf_20210706_220003.nc ncf_20210706_221420.nc ncf_20210706_222834.nc
ncf_20210706_220448.nc ncf_20210706_221906.nc
ncf_20210706_220935.nc ncf_20210706_222350.nc
/tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706:
ncf_20210706_220249.nc ncf_20210706_221204.nc ncf_20210706_222102.nc
ncf_20210706_220723.nc ncf_20210706_221633.nc ncf_20210706_222531.nc
Merge Cartesian files into mosaic, using MdvMerge2
# Run MdvMerge2 to merrge the Cart data from 3 radars into a mosaic
!/usr/local/lrose/bin/MdvMerge2 -params params/MdvMerge2.mosaic -start "2021 07 06 22 00 00" -end "2021 07 06 22 30 00" -debug
======================================================================
Program 'MdvMerge2'
Run-time 2024/02/26 09:02:28.
Copyright (c) 1992 - 2024
University Corporation for Atmospheric Research (UCAR)
National Center for Atmospheric Research (NCAR)
Boulder, Colorado, USA.
Redistribution and use in source and binary forms, with
or without modification, are permitted provided that the following
conditions are met:
1) Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
2) Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
3) Neither the name of UCAR, NCAR nor the names of its contributors, if
any, may be used to endorse or promote products derived from this
software without specific prior written permission.
4) If the software is modified to produce derivative works, such modified
software should be clearly marked, so as not to confuse it with the
version available from UCAR.
======================================================================
Archive trigger
Start time: 2021/07/06 22:00:00
End time: 2021/07/06 22:30:00
Prev time: 2021/07/06 21:55:00
Next time: 2021/07/06 22:00:00
Creating InputFile for url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Field names: DBZ RATE_HYBRID PID range
Creating InputFile for url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Field names: DBZ RATE_HYBRID PID range
Creating InputFile for url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Field names: DBZ RATE_HYBRID PID range
Next trigger: 2021/07/06 22:00:00
----> Trigger time: 2021/07/06 22:00:00
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:00:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_220003.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:00:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_220249.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:00:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_220000.nc
Writing merged MDV file, time 2021/07/06 22:00:00 to URL /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_220000.mdv.cf.nc
Next trigger: 2021/07/06 22:05:00
----> Trigger time: 2021/07/06 22:05:00
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:05:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_220448.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:05:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_220249.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:05:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_220430.nc
Writing merged MDV file, time 2021/07/06 22:05:00 to URL /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_220500.mdv.cf.nc
Next trigger: 2021/07/06 22:10:00
----> Trigger time: 2021/07/06 22:10:00
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:10:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_220935.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:10:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_221204.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:10:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_220921.nc
Writing merged MDV file, time 2021/07/06 22:10:00 to URL /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_221000.mdv.cf.nc
Next trigger: 2021/07/06 22:15:00
----> Trigger time: 2021/07/06 22:15:00
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:15:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_221420.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:15:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_221633.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:15:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_221600.nc
Writing merged MDV file, time 2021/07/06 22:15:00 to URL /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_221500.mdv.cf.nc
Next trigger: 2021/07/06 22:20:00
----> Trigger time: 2021/07/06 22:20:00
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:20:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_221906.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:20:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_222102.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:20:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_222051.nc
Writing merged MDV file, time 2021/07/06 22:20:00 to URL /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_222000.mdv.cf.nc
Next trigger: 2021/07/06 22:25:00
----> Trigger time: 2021/07/06 22:25:00
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:25:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_222350.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:25:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_222531.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:25:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_222533.nc
Writing merged MDV file, time 2021/07/06 22:25:00 to URL /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_222500.mdv.cf.nc
Next trigger: 2021/07/06 22:30:00
----> Trigger time: 2021/07/06 22:30:00
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:30:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_222834.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:30:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_222531.nc
READ_CLOSEST
Reading data for URL: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Mdvx read request
-----------------
Encoding type: ENCODING_FLOAT32 (FLOAT)
Compression type: COMPRESSION_NONE
Scaling type: SCALING_ROUNDED
Composite?: 0
FillMissing?: 0
Field names: DBZ, RATE_HYBRID, PID, range
FieldFileHeaders?: 0
Search mode: READ_CLOSEST
Search time: 2021/07/06 22:30:00
Search margin: 600 secs
Read dir: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read32BitHeaders?: false
Read dir url: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC
Read data from file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_222533.nc
Writing merged MDV file, time 2021/07/06 22:30:00 to URL /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_223000.mdv.cf.nc
Next trigger: 2021/07/06 22:35:00
Done
# List the mosaic files created by MdvMerge2
!ls -R ${NEXRAD_DATA_DIR}/mdv/radarCart/mosaic/2*
/tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706:
20210706_220000.mdv.cf.nc 20210706_221500.mdv.cf.nc 20210706_223000.mdv.cf.nc
20210706_220500.mdv.cf.nc 20210706_222000.mdv.cf.nc
20210706_221000.mdv.cf.nc 20210706_222500.mdv.cf.nc
Read in file from radar mosaic
# Read in example radar mosaic for a single time
filePathMosaic = os.path.join(nexradDataDir, 'mdv/radarCart/mosaic/20210706/20210706_221500.mdv.cf.nc')
dsMosaic = nc.Dataset(filePathMosaic)
print("Radar mosaic file path: ", filePathMosaic)
print("Radar mosaic data set: ", dsMosaic)
# Compute time
uTimeSecs = dsMosaic['start_time'][0]
startTime = datetime.datetime.fromtimestamp(int(uTimeSecs))
startTimeStr = startTime.strftime('%Y/%m/%d-%H:%M:%S UTC')
print("Start time: ", startTimeStr)
# print(dsMosaic['DBZ'])
#for dim in dsMosaic.dimensions.values():
# print(dim)
#for var in dsMosaic.variables.values():
# print("========================================")
# print(var)
# print("========================================")
# create 3D dbz array with nans for missing vals
dsDbz = dsMosaic['DBZ']
dbz3D = np.array(dsDbz)
fillValue = dsDbz._FillValue
# print("fillValue: ", fillValue)
# if 4D (i.e. time is dim0) change to 3D
if (len(dbz3D.shape) == 4):
dbz3D = dbz3D[0]
# Compute mosaic grid limits
(nZMosaic, nYMosaic, nXMosaic) = dbz3D.shape
lon = np.array(dsMosaic['x0'])
lat = np.array(dsMosaic['y0'])
ht = np.array(dsMosaic['z0'])
dLonMosaic = lon[1] - lon[0]
dLatMosaic = lat[1] - lat[0]
minLonMosaic = lon[0] - dLonMosaic / 2.0
maxLonMosaic = lon[-1] + dLonMosaic / 2.0
minLatMosaic = lat[0] - dLatMosaic / 2.0
maxLatMosaic = lat[-1] + dLatMosaic / 2.0
minHtMosaic = ht[0]
maxHtMosaic = ht[-1]
print("minLonMosaic, maxLonMosaic: ", minLonMosaic, maxLonMosaic)
print("minLatMosaic, maxLatMosaic: ", minLatMosaic, maxLatMosaic)
print("minHt, maxHt: ", minHtMosaic, maxHtMosaic)
print("hts: ", ht)
del lon, lat, ht
# Compute column-max reflectivity
dbzPlaneMax = np.amax(dbz3D, (0))
dbzPlaneMax[dbzPlaneMax == fillValue] = np.nan
print("Shape of composite DBZ grid: ", dbzPlaneMax.shape)
#print(dbzPlaneMax[dbzPlaneMax != np.nan])
#print(np.min(dbzPlaneMax[dbzPlaneMax != np.nan]))
#print(np.max(dbzPlaneMax))
Radar mosaic file path: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_221500.mdv.cf.nc
Radar mosaic data set: <class 'netCDF4._netCDF4.Dataset'>
root group (NETCDF4 data model, file format HDF5):
Conventions: CF-1.6
history: Data merged from following files:
/tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_221420.nc
/tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_221633.nc
/tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_221600.nc
source: NEXRAD radars
title: 3D RADAR MOSAIC
comment:
dimensions(sizes): time(1), bounds(2), x0(900), y0(700), z0(31)
variables(dimensions): float64 time(time), float64 start_time(time), float64 stop_time(time), float64 time_bounds(time, bounds), float32 x0(x0), float32 y0(y0), float32 z0(z0), int32 grid_mapping_0(), int32 mdv_master_header(time), int16 DBZ(time, z0, y0, x0), int16 RATE_HYBRID(time, z0, y0, x0), int16 PID(time, z0, y0, x0), int16 range(time, z0, y0, x0)
groups:
Start time: 2021/07/06-22:14:20 UTC
minLonMosaic, maxLonMosaic: -104.50500106811523 -95.50500106811523
minLatMosaic, maxLatMosaic: 35.4950008392334 42.49499702453613
minHt, maxHt: 1.25 20.0
hts: [ 1.25 1.5 1.75 2. 2.25 2.5 2.75 3. 3.5 4. 4.5 5.
5.5 6. 6.5 7. 7.5 8. 8.5 9. 10. 11. 12. 13.
14. 15. 16. 17. 18. 19. 20. ]
Shape of composite DBZ grid: (700, 900)
Create map for Cartesian grid plotting
# Create map for plotting lat/lon grids
def new_map(fig):
## Create projection centered on data
proj = ccrs.PlateCarree()
## New axes with the specified projection:
ax = fig.add_subplot(1, 1, 1, projection=proj)
## Set extent the same as radar mosaic
ax.set_extent([minLonMosaic, maxLonMosaic, minLatMosaic, maxLatMosaic])
## Add grid lines & labels:
gl = ax.gridlines( crs=ccrs.PlateCarree()
, draw_labels=True
, linewidth=1
, color='lightgray'
, alpha=0.5, linestyle='--'
)
gl.top_labels = False
gl.left_labels = True
gl.right_labels = False
gl.xlines = True
gl.ylines = True
gl.xformatter = LONGITUDE_FORMATTER
gl.yformatter = LATITUDE_FORMATTER
gl.xlabel_style = {'size': 8, 'weight': 'bold'}
gl.ylabel_style = {'size': 8, 'weight': 'bold'}
return ax
Plot column-max reflectivity in plan view, and a N/S and W/E cross section of reflectivity
# Plot column-max reflectivity
figDbzComp = plt.figure(figsize=(8, 8), dpi=150)
axDbzComp = new_map(figDbzComp)
plt.imshow(dbzPlaneMax,
cmap='pyart_Carbone42',
interpolation = 'bilinear',
origin = 'lower',
extent = (minLonMosaic, maxLonMosaic, minLatMosaic, maxLatMosaic))
axDbzComp.add_feature(cfeature.BORDERS, linewidth=0.5, edgecolor='black')
axDbzComp.add_feature(cfeature.STATES, linewidth=0.3, edgecolor='brown')
#axDbzComp.coastlines('10m', 'darkgray', linewidth=1, zorder=0)
plt.colorbar(label="DBZ", orientation="vertical", shrink=0.5)
plt.title("Radar mosaic column-max DBZ: " + startTimeStr)
Text(0.5, 1.0, 'Radar mosaic column-max DBZ: 2021/07/06-22:14:20 UTC')
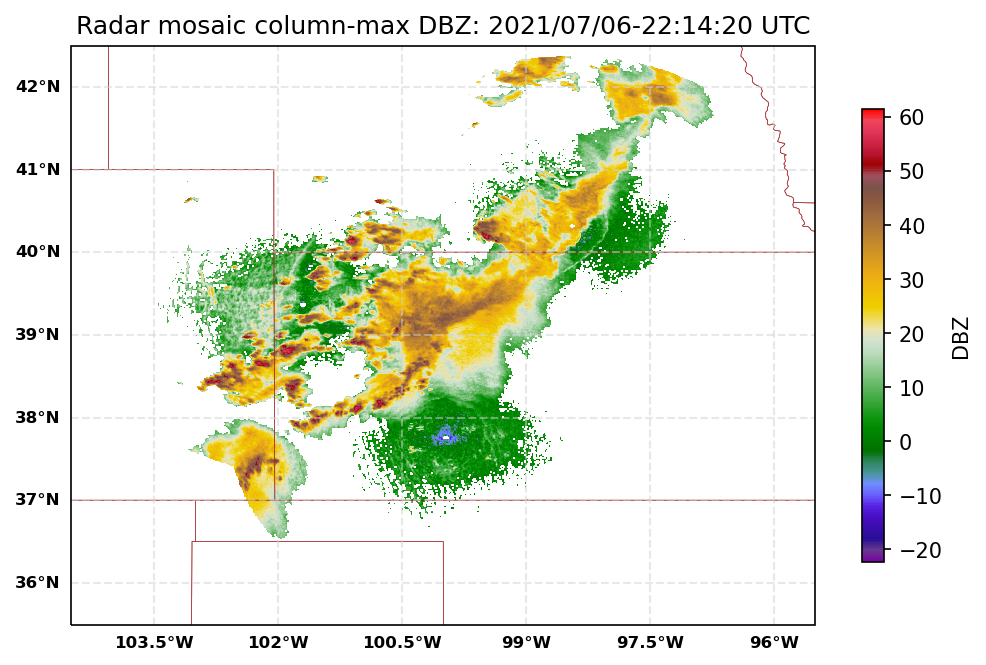
# Compute W-E DBZ vertical section
nYHalfMosaic = int(nYMosaic/2)
dbzVertWE = dbz3D[:, nYHalfMosaic:(nYHalfMosaic+1), :]
dbzVertWE = dbzVertWE.reshape(dbzVertWE.shape[0], dbzVertWE.shape[2])
print('dbzVertWE.shape: ', dbzVertWE.shape)
dbzVertWE[dbzVertWE == fillValue] = np.nan
dbzVertWEMax = np.amax(dbz3D, axis=1)
dbzVertWEMax[dbzVertWEMax == fillValue] = np.nan
print('dbzVertWEMax.shape: ', dbzVertWEMax.shape)
# Compute DBZ N-S vertical section
nXHalfMosaic = int(nXMosaic/2)
dbzVertNS = dbz3D[:, :, nXHalfMosaic:(nXHalfMosaic+1)]
dbzVertNS = dbzVertNS.reshape(dbzVertNS.shape[0], dbzVertNS.shape[1])
dbzVertNS[dbzVertNS == fillValue] = np.nan
print('dbzVertNS.shape: ', dbzVertNS.shape)
dbzVertNSMax = np.amax(dbz3D, axis=2)
dbzVertNSMax[dbzVertNSMax == fillValue] = np.nan
print('dbzVertNSMax.shape: ', dbzVertNSMax.shape)
dbzVertWE.shape: (31, 900)
dbzVertWEMax.shape: (31, 900)
dbzVertNS.shape: (31, 700)
dbzVertNSMax.shape: (31, 700)
# Plot mid W-E DBZ vertical section
fig2 = plt.figure(num=2, figsize=[12, 8], layout='constrained')
ax2a = fig2.add_subplot(211, xlim = (minLonMosaic, maxLonMosaic), ylim = (minHtMosaic, maxHtMosaic))
plt.imshow(dbzVertWE,
cmap='pyart_Carbone42',
interpolation = 'bilinear',
origin = 'lower',
extent = (minLonMosaic, maxLonMosaic, minHtMosaic, maxHtMosaic))
ax2a.set_aspect(0.15)
ax2a.set_xlabel('Longitude (deg)')
ax2a.set_ylabel('Height (km)')
plt.title("Vert slice mid W-E DBZ: " + startTimeStr)
# Plot mid N-S DBZ vertical section
ax2b = fig2.add_subplot(212, xlim = (minLatMosaic, maxLatMosaic), ylim = (minHtMosaic, maxHtMosaic))
plt.imshow(dbzVertNS,
cmap='pyart_Carbone42',
interpolation = 'bilinear',
origin = 'lower',
extent = (minLatMosaic, maxLatMosaic, minHtMosaic, maxHtMosaic))
ax2b.set_aspect(0.15)
ax2b.set_xlabel('Latitude (deg)')
ax2b.set_ylabel('Height (km)')
plt.title("Vert slice mid NS DBZ: " + startTimeStr)
plt.colorbar(label="DBZ", orientation="horizontal", fraction=0.1)
<matplotlib.colorbar.Colorbar at 0x7fd212a49490>
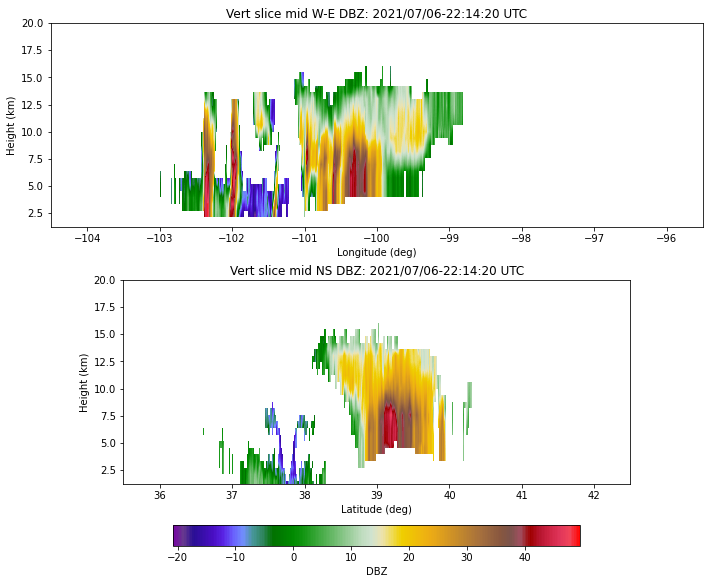
View the Ecco parameter file
The paths of the input files, and the output results file, are specified in the parameters.
Also included are all of the parameters used to control the algorithm.
# View the param file
!cat ./params/Ecco.nexrad_mosaic
/**********************************************************************
* TDRP params for Ecco
**********************************************************************/
//======================================================================
//
// Program name: Ecco.
//
// Ecco finds convective and stratiform regions within a Cartesian radar
// volume.
//
//======================================================================
//======================================================================
//
// PROCESS CONTROL.
//
//======================================================================
///////////// debug ///////////////////////////////////
//
// Debug option.
//
// If set, debug messages will be printed appropriately.
//
//
// Type: enum
// Options:
// DEBUG_OFF
// DEBUG_NORM
// DEBUG_VERBOSE
// DEBUG_EXTRA
//
debug = DEBUG_OFF;
///////////// instance ////////////////////////////////
//
// Process instance.
//
// Used for registration with procmap.
//
//
// Type: string
//
instance = "nexrad_mosaic";
///////////// mode ////////////////////////////////////
//
// Operating mode.
//
// In REALTIME mode, the program waits for a new input file. In ARCHIVE
// mode, it moves through the data between the start and end times set
// on the command line. In FILELIST mode, it moves through the list of
// file names specified on the command line.
//
//
// Type: enum
// Options:
// ARCHIVE
// REALTIME
// FILELIST
//
mode = ARCHIVE;
///////////// use_multiple_threads ////////////////////
//
// Option to use multiple threads for speed.
//
// Computing the texture is the most time consuming step. If this is
// true, then the texture will be computer for each vertical level in a
// separate thread, in parallel. This speeds up the processing. If this
// is false, the threads will be called serially. This is useful for
// debugging.
//
//
// Type: boolean
//
use_multiple_threads = TRUE;
//======================================================================
//
// DATA INPUT.
//
//======================================================================
///////////// input_url ///////////////////////////////
//
// URL for input data.
//
// This is used in REALTIME and ARCHIVE modes only. In FILELIST mode,
// the file paths are specified on the command line.
//
//
// Type: string
//
input_url = "$(NEXRAD_DATA_DIR)/mdv/radarCart/mosaic";
///////////// dbz_field_name //////////////////////////
//
// dBZ field name in input MDV files.
//
//
// Type: string
//
dbz_field_name = "DBZ";
//======================================================================
//
// ALGORITHM PARAMETERS.
//
//======================================================================
///////////// min_valid_height ////////////////////////
//
// Min height used in analysis (km).
//
// Only data at or above this altitude is used.
//
//
// Type: double
//
min_valid_height = 0;
///////////// max_valid_height ////////////////////////
//
// Max height used in analysis (km).
//
// Only data at or below this altitude is used.
//
//
// Type: double
//
max_valid_height = 25;
///////////// min_valid_dbz ///////////////////////////
//
// Minimum reflectivity threshold for this analysis (dBZ).
//
// Reflectivity below this threshold is set to missing.
//
//
// Type: double
//
min_valid_dbz = 0;
///////////// base_dbz ////////////////////////////////
//
// Set base DBZ value.
//
// Before computing the texture, we subtract the baseDBZ from the
// measured DBZ. This adjusts the DBZ values into the positive range.
// For S-, C- and X-band radars, this can be set to 0 dBZ, which is the
// default. For Ka-band radars this should be around -10 dBZ. For W-band
// radars -20 dBZ is appropriate.
//
//
// Type: double
//
base_dbz = 0;
///////////// min_valid_volume_for_convective /////////
//
// Min volume of a convective region (km3).
//
// Regions of smaller volume will be labeled SMALL.
//
//
// Type: double
//
min_valid_volume_for_convective = 20;
///////////// min_vert_extent_for_convective //////////
//
// Min vertical echo extent of a convective region (km).
//
// The vertical extent is computed as the mid height of the top layer in
// the echo minus the mid height of the bottom layer. For an echo that
// exists in only one layer, the vertical extent would therefore be
// zero. This parameter lets us require that a valid convective echo
// exist in multiple layers, which is desirable and helps to remove
// spurious echoes as candidates for convection.
//
//
// Type: double
//
min_vert_extent_for_convective = 3;
///////////// dbz_for_echo_tops ///////////////////////
//
// Reflectivity for determing echo tops.
//
// Echo tops are defined as the max ht with reflectivity at or above
// this value.
//
//
// Type: double
//
dbz_for_echo_tops = 18;
//======================================================================
//
// COMPUTING REFLECTIVITY TEXTURE.
//
//======================================================================
///////////// texture_radius_km ///////////////////////
//
// Radius for texture analysis (km).
//
// We determine the reflectivity 'texture' at a point by computing the
// standard deviation of the square of the reflectivity, for all grid
// points within this radius of the central point. We then compute the
// square root of that sdev.
//
//
// Type: double
//
texture_radius_km = 7;
///////////// min_valid_fraction_for_texture //////////
//
// Minimum fraction of surrounding points for texture computations.
//
// For a valid computation of texture, we require at least this fraction
// of points around the central point to have valid reflectivity.
//
//
// Type: double
//
min_valid_fraction_for_texture = 0.25;
///////////// min_valid_fraction_for_fit //////////////
//
// Minimum fraction of surrounding points for 2D fit to DBZ.
//
// We compute a 2D fit to the reflectivity around a grid point, to
// remove any systematic gradient. For a valid fit, we require at least
// this fraction of points around the central point to have valid
// reflectivity.
//
//
// Type: double
//
min_valid_fraction_for_fit = 0.67;
//======================================================================
//
// CONVERTING REFLECTIVITY TEXTURE TO CONVECTIVITY.
//
// Convectivity ranges from 0 to 1. To convert texture to convectivity,
// we apply a piece-wise linear transfer function. This section defines
// the lower texture limit and the upper texture limit. At or below the
// lower limit convectivity is set to 0. At or above the upper limit
// convectivity is set to 1. Between these two limits convectivity
// varies linearly with texture.
//
//======================================================================
///////////// texture_limit_low ///////////////////////
//
// Lower limit for texture.
//
// Below this texture the convectivity is set to 0.
//
//
// Type: double
//
texture_limit_low = 0;
///////////// texture_limit_high //////////////////////
//
// Upper limit for texture.
//
// Above this texture the convectivity is set to 1. Between the limits
// convectivity varies linearly with texture.
//
//
// Type: double
//
texture_limit_high = 30;
//======================================================================
//
// SETTING CONVECTIVE OR STRATIFORM FLAGS BASED ON CONVECTIVITY.
//
// If neither is set, we flag the point as MIXED.
//
//======================================================================
///////////// min_convectivity_for_convective /////////
//
// Minimum convectivity for convective at a point.
//
// If the convectivity at a point exceeds this value, we set the
// convective flag at this point.
//
//
// Type: double
//
min_convectivity_for_convective = 0.5;
///////////// max_convectivity_for_stratiform /////////
//
// Maximum convectivity for stratiform at a point.
//
// If the convectivity at a point is less than this value, we set the
// stratiform flag at this point. If it is above this but less than
// min_convectivity_for_convective we flag the point as MIXED.
//
//
// Type: double
//
max_convectivity_for_stratiform = 0.4;
//======================================================================
//
// DETERMINING ADVANCED ECHO TYPE USING CLUMPING AND TEMPERATURE.
//
// We performing clumping on the convectivity field to identify
// convective entities as objects. The main threshold used for the
// clumping is min_convectivity_for_convective. By default a secondary
// threshold is also used - see below.
//
//======================================================================
///////////// clumping_use_dual_thresholds ////////////
//
// Option to use dual thresholds to better identify convective clumps.
//
// NOTE: this step is performed in 2D. If set, the clumping is performed
// in two stages. First, an outer convectivity envelope is computed,
// using min_convectivity_for_convective. Then, using the parameters
// below, for each clump a search is performed for sub-clumps within the
// envelope of the main clump, suing the secondary threshold. If there
// is only one sub-clump, the original clump is used unchanged. If there
// are two or more valid sub-clumps, based on the parameters below,
// these sub-clumps are progrresively grown to where they meet, or to
// the original clump envelope. The final 3D clumps are computed by
// breaking the original clump into regions based upon these secondary
// 2D areas.
//
//
// Type: boolean
//
clumping_use_dual_thresholds = TRUE;
///////////// clumping_secondary_convectivity /////////
//
// Secondary convectivity threshold for clumping.
//
// We use the secondary threshold to find sub-clumps within the envelope
// of each original clump.
//
//
// Type: double
//
clumping_secondary_convectivity = 0.65;
///////////// all_subclumps_min_area_fraction /////////
//
// Min area of all sub-clumps, as a fraction of the original clump area.
//
// We sum the areas of the sub-clumps, and compute the fraction relative
// to the area of the original clump. For the sub-clumps to be valid,
// the computed fraction must exceed this parameter.
//
//
// Type: double
//
all_subclumps_min_area_fraction = 0.33;
///////////// each_subclump_min_area_fraction /////////
//
// Min area of each valid sub-clump, as a fraction of the original
// clump.
//
// We compute the area of each sub-clump, and compute the fraction
// relative to the area of the original clump. For a subclump to be
// valid, the area fraction must exceed this parameter.
//
//
// Type: double
//
each_subclump_min_area_fraction = 0.02;
///////////// each_subclump_min_area_km2 //////////////
//
// Min area of each valid sub-clump (km2).
//
// We compute the area of each sub-clump. For a subclump to be valid,
// the area must exceed this parameter.
//
//
// Type: double
//
each_subclump_min_area_km2 = 2;
//======================================================================
//
// SPECIFYING VERTICAL LEVELS FOR ADVANCED ECHO TYPE - TEMPERATURE or
// HEIGHT?.
//
// We need to specify the vertical separation between shallow, mid-level
// and high clouds. We use the freezing level to separate warm clouds
// and cold clouds. And we use the divergence level to separate the
// mid-level clouds from high-level clouds such as anvil. These vertical
// limits can be specified as heights MSL (in km), or as temperatures.
// If temperatures are used, we read in the temperature profile from a
// model.
//
//======================================================================
///////////// vert_levels_type ////////////////////////
//
// How we specify the vertical levels.
//
// If temperatures are used, we need to read in the temperature profile
// from a model.
//
//
// Type: enum
// Options:
// VERT_LEVELS_BY_TEMP
// VERT_LEVELS_BY_HT
//
vert_levels_type = VERT_LEVELS_BY_TEMP;
///////////// temp_profile_url ////////////////////////
//
// URL for temperature profile data, in MDV/Netcdf-CF format.
//
// We read in the model data that is closest in time to the reflectivity
// data.
//
//
// Type: string
//
temp_profile_url = "$(NEXRAD_DATA_DIR)/mdv/ruc";
///////////// temp_profile_field_name /////////////////
//
// Name of temperature field in the model data. This should be in
// degrees C.
//
//
// Type: string
//
temp_profile_field_name = "TMP";
///////////// temp_profile_search_margin //////////////
//
// Search margin for finding the temp profile data (secs).
//
// The temp profile must be within this number of seconds of the dbz
// data.
//
//
// Type: int
//
temp_profile_search_margin = 21600;
///////////// shallow_threshold_temp //////////////////
//
// Shallow cloud temperature threshold (degC).
//
// Shallow cloud tops are below this temperature. Used if
// vert_levels_type = VERT_LEVELS_BY_TEMP.
//
//
// Type: double
//
shallow_threshold_temp = 0;
///////////// deep_threshold_temp /////////////////////
//
// Deep cloud temperature threshold (degC).
//
// Deep clouds extend above this height. Used if vert_levels_type =
// VERT_LEVELS_BY_TEMP.
//
//
// Type: double
//
deep_threshold_temp = -25;
///////////// shallow_threshold_ht ////////////////////
//
// Shallow cloud height threshold if temperature is not available (km).
//
// Shallow cloud tops are below this height. Used if vert_levels_type =
// VERT_LEVELS_BY_HT.
//
//
// Type: double
//
shallow_threshold_ht = 4;
///////////// deep_threshold_ht ///////////////////////
//
// Deep cloud height threshold if temperature is not available (km).
//
// Deep clouds extend above this height. Used if vert_levels_type =
// VERT_LEVELS_BY_HT.
//
//
// Type: double
//
deep_threshold_ht = 9;
//======================================================================
//
// DETERMINING ADVANCED CATEGORY FROM CLUMP PROPERTIES.
//
// Based on the temp or height criteria above, we compute the deep, mid
// and shallow convective fractions within each sub-clump. We also
// determine whether there is stratiform echo below the clump. The
// following parameters are then used to determine the deep, elevated,
// mid or shallow echo types for the convection. If a determination is
// not clear, the overall category is set to mixed.
//
//======================================================================
///////////// min_conv_fraction_for_deep //////////////
//
// The minimun convective fraction in the clump for deep convection.
//
// The fraction of deep within the clump must exceed this for an echo
// type of deep.
//
//
// Type: double
//
min_conv_fraction_for_deep = 0.05;
///////////// min_conv_fraction_for_shallow ///////////
//
// The minimun convective fraction in the clump for shallow convection.
//
// The fraction of shallow within the clump must exceed this for an echo
// type of shallow.
//
//
// Type: double
//
min_conv_fraction_for_shallow = 0.95;
///////////// max_shallow_conv_fraction_for_elevated //
//
// The maximum shallow convective fraction in the clump for elevated
// convection.
//
// The fraction of shallow within the clump must be less than this for
// an echo type of elevated.
//
//
// Type: double
//
max_shallow_conv_fraction_for_elevated = 0.05;
///////////// max_deep_conv_fraction_for_elevated /////
//
// The maximum deep convective fraction in the clump for elevated
// convection.
//
// The fraction of deep within the clump must be less than this for an
// echo type of elevated.
//
//
// Type: double
//
max_deep_conv_fraction_for_elevated = 0.25;
///////////// min_strat_fraction_for_strat_below //////
//
// The minimun area fraction of stratiform echo below the clump to
// determine there is stratiform below.
//
// For elevated convection, we need to determine if there is stratiform
// echo below. For a designation of elevated, this is the minimum
// fraction of the area below the clump that has stratiform echo in the
// plane immediately below it.
//
//
// Type: double
//
min_strat_fraction_for_strat_below = 0.9;
//======================================================================
//
// DATA OUTPUT.
//
//======================================================================
///////////// output_url //////////////////////////////
//
// Output URL.
//
// Output files are written to this URL.
//
//
// Type: string
//
output_url = "$(NEXRAD_DATA_DIR)/mdv/ecco/mosaic";
///////////// write_partition /////////////////////////
//
// Write out partition fields.
//
// This will write out the 3D, 2D and column-max partition.
//
//
// Type: boolean
//
write_partition = TRUE;
///////////// write_texture ///////////////////////////
//
// Write out texture fields.
//
// This will write out the 3D and column-max texture.
//
//
// Type: boolean
//
write_texture = TRUE;
///////////// write_convectivity //////////////////////
//
// Write out convectivity fields.
//
// This will write out the 3D and column-max convectivity.
//
//
// Type: boolean
//
write_convectivity = TRUE;
///////////// write_3D_dbz ////////////////////////////
//
// Write out 3D dbz field.
//
// This will be an echo of the input field.
//
//
// Type: boolean
//
write_3D_dbz = TRUE;
///////////// write_col_max_dbz ///////////////////////
//
// Write out column maximum dbz field.
//
// This is the max reflectivity at any height.
//
//
// Type: boolean
//
write_col_max_dbz = TRUE;
///////////// write_convective_dbz ////////////////////
//
// Write out convective dbz field.
//
// This will write out the 3D convective DBZ field.
//
//
// Type: boolean
//
write_convective_dbz = TRUE;
///////////// write_tops //////////////////////////////
//
// Write out echo, convective and stratiform tops.
//
// These are 2D fields.
//
//
// Type: boolean
//
write_tops = TRUE;
///////////// write_fraction_active ///////////////////
//
// Write out 2D field showing fraction active.
//
// This the active fraction in the computational circle.
//
//
// Type: boolean
//
write_fraction_active = TRUE;
///////////// write_height_grids //////////////////////
//
// Write out 2D field showing shallow and deep heights.
//
// These are based on model temperature.
//
//
// Type: boolean
//
write_height_grids = FALSE;
///////////// write_temperature ///////////////////////
//
// Write out 3D temperature field.
//
// This comes from a model, remapped onto the reflectivity grid.
//
//
// Type: boolean
//
write_temperature = TRUE;
///////////// write_clumping_debug_fields /////////////
//
// Option to write fields to the output files for debugging the dual
// threshold clumping.
//
// If this is set, the following debug fields are written to the output
// files: .
//
//
// Type: boolean
//
write_clumping_debug_fields = FALSE;
Run Ecco
Ecco computes the convective/stratiform partition using radar reflectivity in Cartesian coordinates.
We run Ecco by specifying the parameter file, and the start and end times for the analysis.
# Run Ecco using param file
!/usr/local/lrose/bin/Ecco -params ./params/Ecco.nexrad_mosaic -debug -start "2021 07 06 22 00 00" -end "2021 07 06 22 30 00"
======================================================================
Program 'Ecco'
Run-time 2024/02/26 09:03:31.
Copyright (c) 1992 - 2024
University Corporation for Atmospheric Research (UCAR)
National Center for Atmospheric Research (NCAR)
Boulder, Colorado, USA.
Redistribution and use in source and binary forms, with
or without modification, are permitted provided that the following
conditions are met:
1) Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
2) Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
3) Neither the name of UCAR, NCAR nor the names of its contributors, if
any, may be used to endorse or promote products derived from this
software without specific prior written permission.
4) If the software is modified to produce derivative works, such modified
software should be clearly marked, so as not to confuse it with the
version available from UCAR.
======================================================================
Read in file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_220000.mdv.cf.nc
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/ecco/mosaic/20210706/20210706_220000.mdv.cf.nc
Read in file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_220500.mdv.cf.nc
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/ecco/mosaic/20210706/20210706_220500.mdv.cf.nc
Read in file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_221000.mdv.cf.nc
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/ecco/mosaic/20210706/20210706_221000.mdv.cf.nc
Read in file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_221500.mdv.cf.nc
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/ecco/mosaic/20210706/20210706_221500.mdv.cf.nc
Read in file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_222000.mdv.cf.nc
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/ecco/mosaic/20210706/20210706_222000.mdv.cf.nc
Read in file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_222500.mdv.cf.nc
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/ecco/mosaic/20210706/20210706_222500.mdv.cf.nc
Read in file: /tmp/lrose_data/nexrad_mosaic/mdv/radarCart/mosaic/20210706/20210706_223000.mdv.cf.nc
Wrote file: /tmp/lrose_data/nexrad_mosaic/mdv/ecco/mosaic/20210706/20210706_223000.mdv.cf.nc
Read in Ecco results for a selected time
# Read in ecco results for a selected time
filePathEcco = os.path.join(nexradDataDir, 'mdv/ecco/mosaic/20210706/20210706_221500.mdv.cf.nc')
dsEcco = nc.Dataset(filePathEcco)
print(dsEcco)
# create 3D ecco type array with nans for missing vals
eccoField = dsEcco['EchoType3D']
ecco3D = np.array(eccoField)
fillValue = eccoField._FillValue
print("fillValue: ", fillValue)
print("ecco3D.shape: ", ecco3D.shape)
# if 4D (i.e. time is dim0) change to 3D
if (len(ecco3D.shape) == 4):
ecco3D = ecco3D[0]
# Compute Ecco grid limits
(nZEcco, nYEcco, nXEcco) = ecco3D.shape
lon = np.array(dsEcco['x0'])
lat = np.array(dsEcco['y0'])
ht = np.array(dsEcco['z2'])
dLonEcco = lon[1] - lon[0]
dLatEcco = lat[1] - lat[0]
minLonEcco = lon[0] - dLonEcco / 2.0
maxLonEcco = lon[-1] + dLonEcco / 2.0
minLatEcco = lat[0] - dLatEcco / 2.0
maxLatEcco = lat[-1] + dLatEcco / 2.0
minHtEcco = ht[0]
maxHtEcco = ht[-1]
print("minLonEcco, maxLonEcco: ", minLonEcco, maxLonEcco)
print("minLatEcco, maxLatEcco: ", minLatEcco, maxLatEcco)
print("minHt, maxHt: ", minHtEcco, maxHtEcco)
print("ht: ", ht)
del lon, lat, ht
<class 'netCDF4._netCDF4.Dataset'>
root group (NETCDF4 data model, file format HDF5):
Conventions: CF-1.6
history: Converted from NetCDF to MDV, 2024/02/26 09:04:36
Ncf:history: Data merged from following files:
/tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KGLD/20210706/ncf_20210706_221420.nc
/tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KUEX/20210706/ncf_20210706_221633.nc
/tmp/lrose_data/nexrad_mosaic/mdv/radarCart/KDDC/20210706/ncf_20210706_221600.nc
Ncf:comment:
: Stratfinder used to identify stratiform regions
source: NEXRAD radars
title: 3D RADAR MOSAIC
comment:
dimensions(sizes): time(1), bounds(2), x0(900), y0(700), x1(139), y1(72), z0(1), z1(32), z2(31), nbytes_mdv_chunk_0000(485)
variables(dimensions): float64 time(time), float64 start_time(time), float64 stop_time(time), float64 time_bounds(time, bounds), float32 x0(x0), float32 y0(y0), float32 x1(x1), float32 y1(y1), float32 z0(z0), float32 z1(z1), float32 z2(z2), int32 grid_mapping_0(), int32 grid_mapping_1(), int32 mdv_master_header(time), int8 mdv_chunk_0000(time, nbytes_mdv_chunk_0000), int16 DbzTextureComp(time, z0, y0, x0), int16 ConvectivityComp(time, z0, y0, x0), int16 DbzComp(time, z0, y0, x0), int16 FractionActive(time, z0, y0, x0), int16 ConvTops(time, z0, y0, x0), int16 StratTops(time, z0, y0, x0), int16 EchoTops(time, z0, y0, x0), int16 TMP(time, z1, y1, x1), int8 EchoTypeComp(time, z0, y0, x0), int16 DbzConv(time, z2, y0, x0), int16 DbzTexture3D(time, z2, y0, x0), int16 Convectivity3D(time, z2, y0, x0), int16 Dbz3D(time, z2, y0, x0), int8 EchoType3D(time, z2, y0, x0)
groups:
fillValue: -128
ecco3D.shape: (1, 31, 700, 900)
minLonEcco, maxLonEcco: -104.50500106811523 -95.50500106811523
minLatEcco, maxLatEcco: 35.4950008392334 42.49499702453613
minHt, maxHt: 1.25 20.0
ht: [ 1.25 1.5 1.75 2. 2.25 2.5 2.75 3. 3.5 4. 4.5 5.
5.5 6. 6.5 7. 7.5 8. 8.5 9. 10. 11. 12. 13.
14. 15. 16. 17. 18. 19. 20. ]
Compute the column-max of the Ecco 3-D results
# Compute column-max echo type
eccoPlaneMax = np.amax(ecco3D, (0))
eccoPlaneMax[eccoPlaneMax == fillValue] = np.nan
print(eccoPlaneMax.shape)
print(eccoPlaneMax[eccoPlaneMax != np.nan])
print(np.min(eccoPlaneMax[eccoPlaneMax != np.nan]))
print(np.max(eccoPlaneMax))
(700, 900)
[nan nan nan ... nan nan nan]
nan
nan
Plot the column max of the Ecco results
# Plot column-max ecco
figEcco = plt.figure(figsize=(8, 8), dpi=150)
axEcco = new_map(figEcco)
plt.imshow(eccoPlaneMax,
cmap='rainbow', vmin=12, vmax=40,
interpolation = 'bilinear',
origin = 'lower',
extent = (minLonEcco, maxLonEcco, minLatEcco, maxLatEcco))
axEcco.add_feature(cfeature.BORDERS, linewidth=0.5, edgecolor='black')
axEcco.add_feature(cfeature.STATES, linewidth=0.3, edgecolor='brown')
cbarEcco = plt.colorbar(label="Echo type", cax=None, orientation="vertical", shrink=0.6)
cbarEcco.set_ticks([14,16,18,25,32,34,36,38],
labels=['StratLowLow', 'StratMid', 'StratHigh', 'Mixed',
'ConvElev', 'ConvLow', 'ConvMid', 'ConvDeep'])
plt.title("Column max of Echo Type for radar mosaic: " + startTimeStr)
Text(0.5, 1.0, 'Column max of Echo Type for radar mosaic: 2021/07/06-22:14:20 UTC')
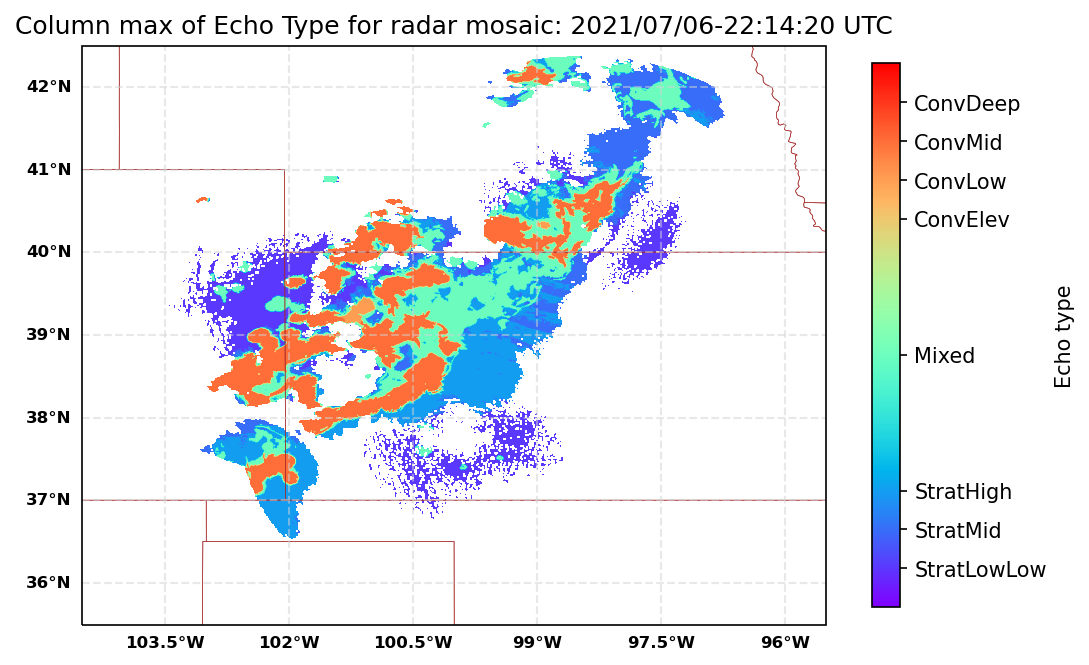
Plot vertical sections for Echo Type
# Compute W-E Ecco vertical section
nYHalfEcco = int(nYEcco/2)
eccoVertWE = ecco3D[:, nYHalfEcco:(nYHalfEcco+1), :]
eccoVertWE = eccoVertWE.reshape(eccoVertWE.shape[0], eccoVertWE.shape[2])
print('eccoVertWE.shape: ', eccoVertWE.shape)
eccoVertWE[eccoVertWE == fillValue] = np.nan
eccoVertWEMax = np.amax(ecco3D, axis=1)
eccoVertWEMax[eccoVertWEMax == fillValue] = np.nan
print('eccoVertWEMax.shape: ', eccoVertWEMax.shape)
# Compute ecco N-S vertical section
nXHalfEcco = int(nXEcco/2)
eccoVertNS = ecco3D[:, :, nXHalfEcco:(nXHalfEcco+1)]
eccoVertNS = eccoVertNS.reshape(eccoVertNS.shape[0], eccoVertNS.shape[1])
eccoVertNS[eccoVertNS == fillValue] = np.nan
print('eccoVertNS.shape: ', eccoVertNS.shape)
eccoVertNSMax = np.amax(ecco3D, axis=2)
eccoVertNSMax[eccoVertNSMax == fillValue] = np.nan
print('eccoVertNSMax.shape: ', eccoVertNSMax.shape)
eccoVertWE.shape: (31, 900)
eccoVertWEMax.shape: (31, 900)
eccoVertNS.shape: (31, 700)
eccoVertNSMax.shape: (31, 700)
# Plot mid W-E ecco vertical section
figEccoVert = plt.figure(num=2, figsize=[12, 8], layout='constrained')
axEv1 = figEccoVert.add_subplot(211, xlim = (minLonEcco, maxLonEcco), ylim = (minHtEcco, maxHtEcco))
plt.imshow(eccoVertWE,
vmin=12, vmax=40,
cmap='rainbow',
interpolation = 'bilinear',
origin = 'lower',
extent = (minLonEcco, maxLonEcco, minHtEcco, maxHtEcco))
axEv1.set_aspect(0.15)
axEv1.set_xlabel('Longitude (deg)')
axEv1.set_ylabel('Height (km)')
plt.title("Echo type vert slice mid W-E: " + startTimeStr)
cbar = plt.colorbar(label="ecco", cax=None, orientation="vertical", shrink=1.5)
cbar.set_ticks([14,16,18,25,32,34,36,38], labels=['StratLowLow', 'StratMid', 'StratHigh', 'Mixed', 'ConvElev', 'ConvLow', 'ConvMid', 'ConvDeep'])
# Plot mid N-S ecco vertical section
axEv2 = figEccoVert.add_subplot(212, xlim = (minLatEcco, maxLatEcco), ylim = (minHtEcco, maxHtEcco))
plt.imshow(eccoVertNS,
vmin=12, vmax=40,
cmap='rainbow',
interpolation = 'bilinear',
origin = 'lower',
extent = (minLatEcco, maxLatEcco, minHtEcco, maxHtEcco))
axEv2.set_aspect(0.15)
axEv2.set_xlabel('Latitude (deg)')
axEv2.set_ylabel('Height (km)')
plt.title("Echo type vert slice mid NS: " + startTimeStr)
Text(0.5, 1.0, 'Echo type vert slice mid NS: 2021/07/06-22:14:20 UTC')
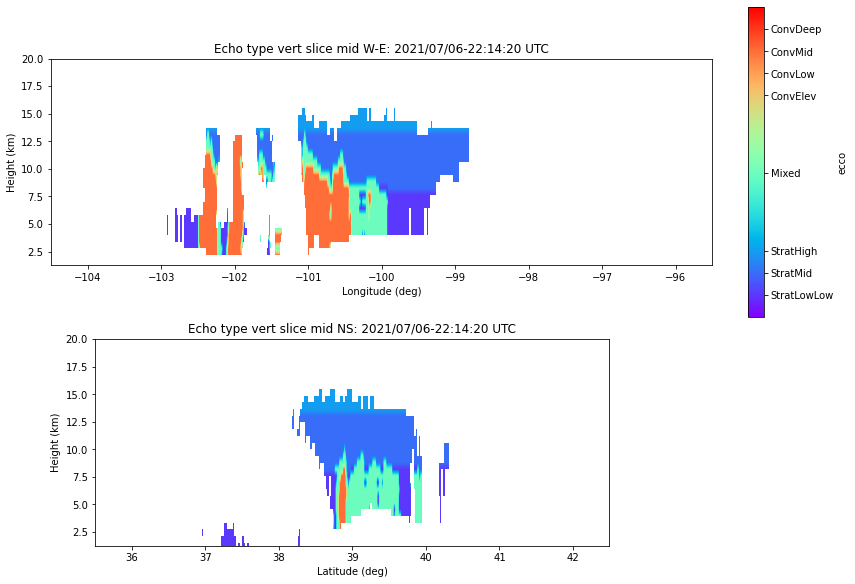